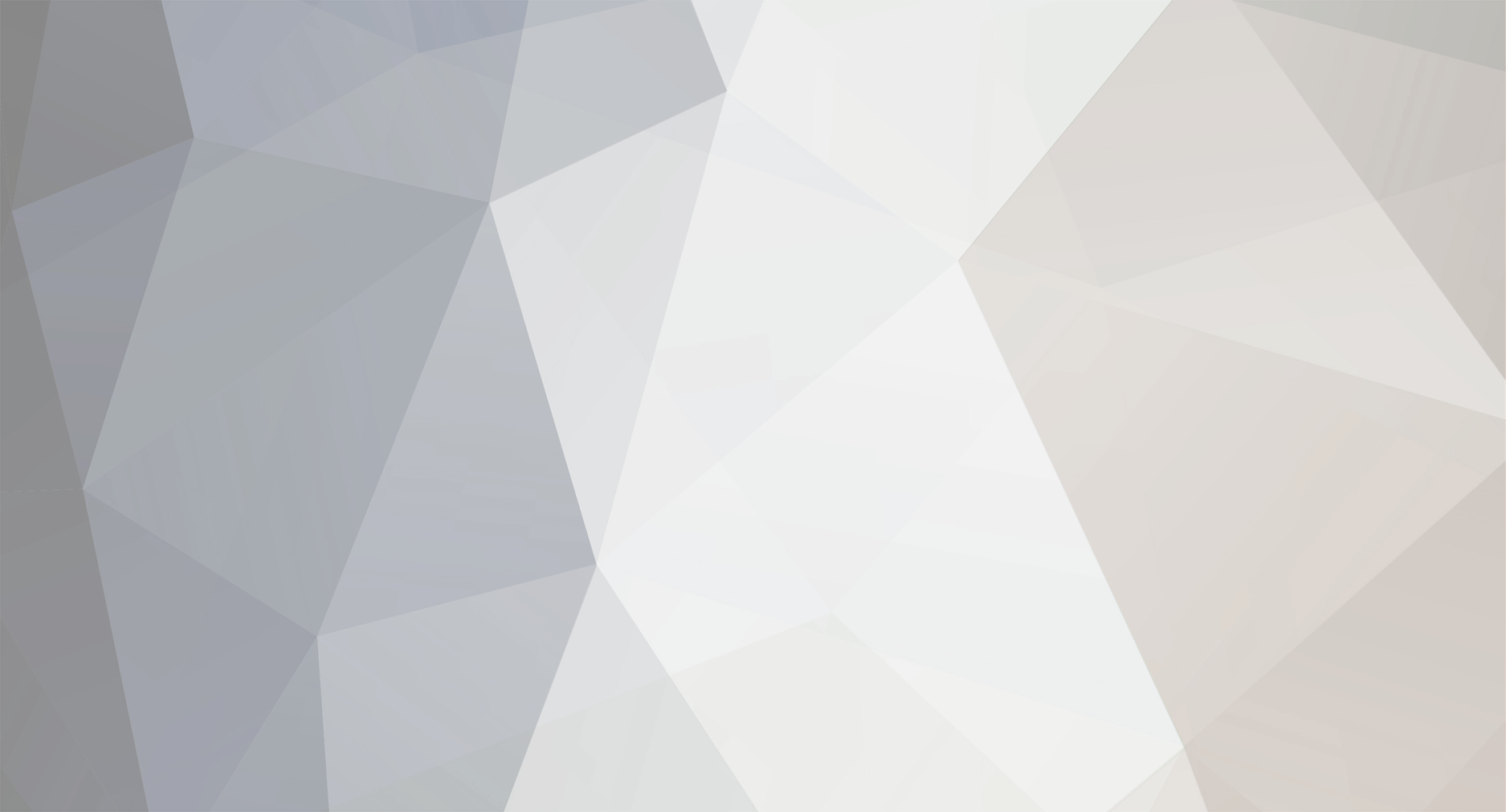
theo
-
Posts
11 -
Joined
-
Last visited
Posts posted by theo
-
-
when I save the data
this is the only error message: the following errors occurred - Array
-
<?php # submit_docs.php # insert submitted documents of ESA $page_title = 'Submit ESA Documents'; include ('includes/header.html'); //Page header: echo '<h1>Submit ESA Documents</h1>'; require ('includes/mysqli_connect.php'); //connect to db //check form: if ($_SERVER['REQUEST_METHOD'] == 'POST') { $errors[] = array(); if (empty($_POST['municipality'])) { $errors[] = 'You forgot to select municipality'; } else { $muni = mysqli_real_escape_string($dbc, trim($_POST['municipality'])); } if (empty($_POST['remarks'])) { $errors[] = 'You forgot to enter remarks'; } else { $rema = mysqli_real_escape_string($dbc, trim($_POST['remarks'])); } if (isset($_POST['submitted'])) { $submitted = implode(',', $_POST['submitted']); $subm = mysqli_real_escape_string($dbc, trim($submitted)); } //if no errors: if (empty($errors)) { //register data of submitted docs //make query: $q = "INSERT INTO subdocs (lgu_id, sub_docs, remarks, registration_date) VALUES ('$id', '$subm', '$rema', NOW())"; $r = @mysqli_query($dbc, $q); //run the query if ($r) { //if it ran ok //print message: echo '<h1>Thank You for submitting ESA Documents</h1>'; } else { //public message: echo '<h1>System Error</h1>'; //debugging message: echo '<p>' . mysqli_error($dbc) . '<br /><br />Query:' . $q . '</p>'; } //end of if mysqli_close($dbc); //close the database include('includes/footer.html'); exit(); } else { //report errors echo '<h1>ERROR</h1> <p class="error">The following errors occurred'; foreach ($errors as $msg) { echo " - $msg<br />\n"; } echo '<p>Please Try Again</p>'; } mysqli_close($dbc); } //make the query: $q = "SELECT lgu_id, municipality FROM lgu ORDER BY municipality ASC"; $r = @mysqli_query ($dbc, $q); //run the query if ($r) { //if OK //fetch and print record echo '<form action="submit_docs.php" method="post">'; echo '<p><b>Municipality:</b> <select name="municipality">'; while ($row = mysqli_fetch_array($r, MYSQLI_ASSOC)) { echo "<option value=\"$row[municipality]\">$row[municipality]</option>\n"; } echo '</select></p>'; echo '<p>Submitted Documents:</p> <p><input type="checkbox" name="submitted[]" value="Project Proposal">Project Proposal <input type="checkbox" name="submitted[]" value="MOA">Memorandum Of Agreement <input type="checkbox" name="submitted[]" value="Certificate of Eligibility">Certificate of Eligibility <input type="checkbox" name="submitted[]" value="Masterlist">Masterlist <input type="checkbox" name="submitted[]" value="Rehabilitaion Plan">Rehabilitation Plan </p>'; echo '<p>Remarks:</p> <p><textarea rows="12" cols="50" name="remarks"></textarea></p> <p><input type="submit" name="submit" value="register" /></p> <input type="hidden" name="lgu_id" value="' . $row['lgu_id'] . '"'; echo '</form>'; } ?>
the error is array
-
having problem with my form:
created checkbox using array;
then I want to save the value of the array to the database
-
thanx..hehe i forgot to update my table online..only the table in the localhost was updated..
-
mysql:5.0.91
php:5.2.12
SELECT CONCAT(masterpx.mpx_lname, ', ', masterpx.mpx_fname) AS name, DATE_FORMAT(inpx_admission.inpx_admission_date, '%M %d, %Y') AS indate FROM masterpx INNER JOIN inpx_admission ON masterpx.mpx_id=inpx_admission.mpx_idfk AND inpx_admission.admission_status = 'admitted' ORDER BY masterpx.mpx_lname
help pls..when i use these query i receive this error message:
Warning: mysqli_num_rows() expects parameter 1 to be mysqli_result
Unknown column 'inpx_admission.admission_status' in 'on clause'
-
hi stuart thanx for the help..i was able to crack the problem..
-
php:5.2.9
mysql:5.0.51a
hi, thanks for the interest.
there is indeed an error found: --->Cannot add or update a child row: a foreign key constraint fails (`docverse`.`inpx_admission`, CONSTRAINT `inpx_admission_ibfk_1` FOREIGN KEY (`mpx_idfk`) REFERENCES `masterpx` (`mpx_id`))
im trying to create a patient record then automatically admits the patient in the other table..
flow of the program:
1. search if the patient is new or has an existing record --
2. if new patient? add the patient(table:masterpatientrecord) then add to the second table(table:inpatient)
3. if patient exist in the masterpatient record? echo/print/update record then add to the second table..
current outcome: patient record works fine..can insert new records but not to the secord table; for existing patient records both can update record and insert to the second table..---->
-
having trouble with my second table: first table insert user record --> works fine/record added to table
second table insert the user record to another table --> problem starts here..it seems the primary key keeps on incrementing but it does not show in the table:
you can check the site at
CODE:
$q = "INSERT INTO masterpx (mpx_lname, mpx_fname, mpx_mname, mpx_bday,
mpx_age, mpx_occupation, mpx_contact, mpx_address, mpx_spouse, mpx_father,
mpx_mother, mpx_religion, mpx_nationality, mpx_citizenship,
mpx_gender, mpx_cstatus, mpx_date_added) VALUES ('$ln', '$fn', '$mn',
'$bday', '$age', '$oc', '$co', '$ad', '$sp', '$fa', '$mo', '$re', '$na',
'$ci', '$ge', '$cs', NOW())";
// run the query:
$r = @mysqli_query($dbc, $q);
if (mysqli_affected_rows($dbc) == 1) {
// insert to inpatient table:
$q = "INSERT INTO inpx_admission (inpx_admission_date, mpx_idfk, doc_idfk)
VALUES (NOW(), '$px_id', '$doc_id')";
$r = @mysqli_query($dbc, $q);
-
thank you sir..
-
having problem with sticky forms inside php:
inside html tags:
<form action="" method="post">
<p>Lastname:<input type="text" name="last_name" size="20" maxlength="20"
value="<?php if (isset($_POST['last_name']))
echo $_POST['last_name']; ?>" /></p>
</form>
inside php: ---> what is the correct syntax
<?php
echo '<form action="" method="post">
<p>Lastname:<input type="text" name="last_name" size="20" maxlength="20"
value="php code here???" /></p>';
?>
Need Help: Checkbox - Array - Insert To Database
in PHP and MySQL for Dynamic Web Sites: Visual QuickPro Guide (4th Edition)
Posted
sir
thank you.