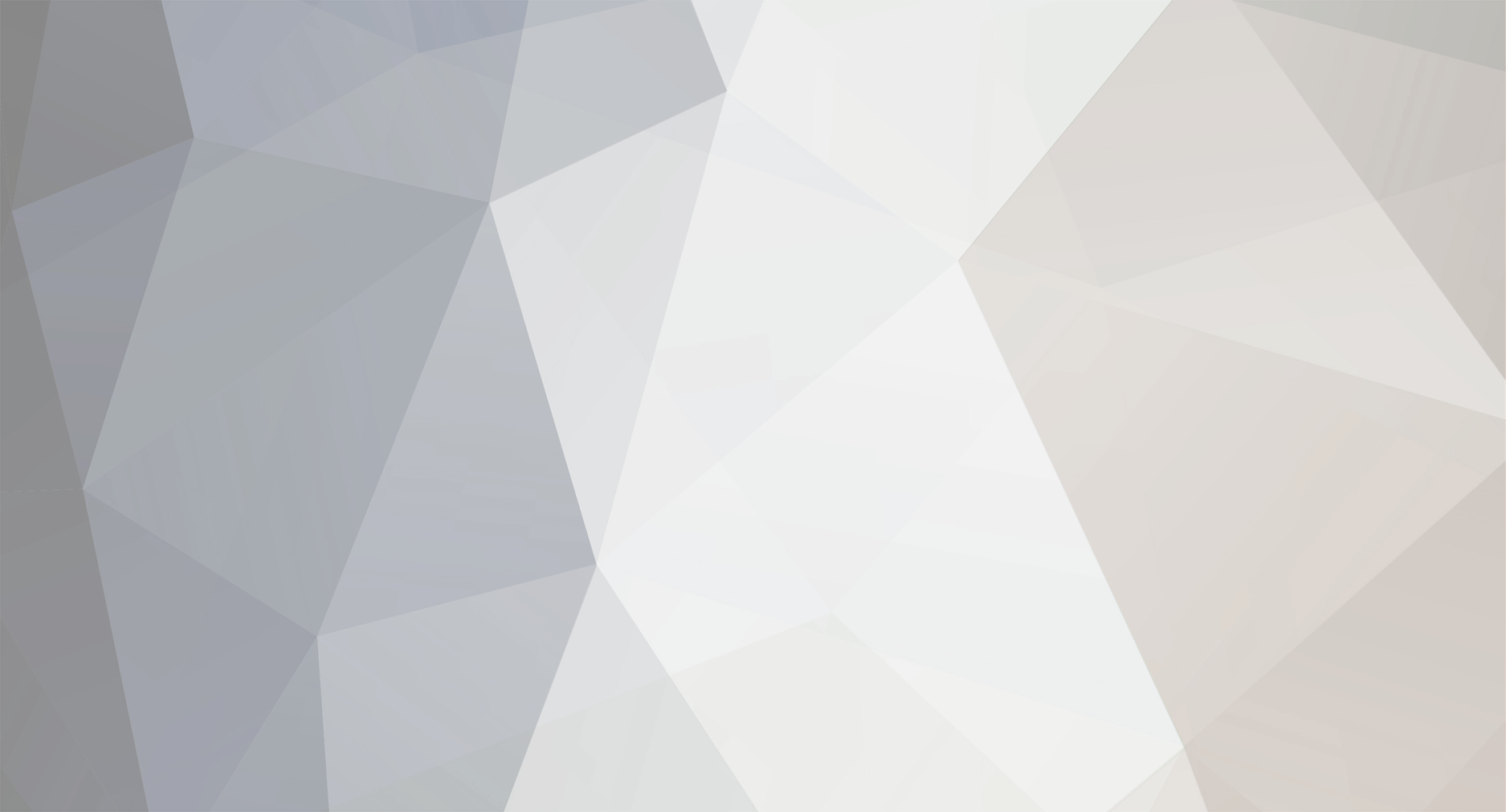
tuxandpucks
-
Posts
17 -
Joined
-
Last visited
Posts posted by tuxandpucks
-
-
Thanks for the help. I tried your suggestion and without success. Also, now I end up with my ide telling me I have an error. I have attached a picture to show what I mean.
$dbc = mysqli_connect('localhost', 'login', 'password', 'database'); " . or die('Error connecting to MySQL server.');
It seems you've left the ;" . after mysqli_connect() which is causing your whole sql definition to mess up.
Take out the semicolon, quotation, and the period and see if it works:
$dbc = mysqli_connect('localhost', 'login', 'password', 'database') or die('Error connecting to MySQL server.');
-
Hey everyone,
I am in need of that high quality help I receive when visiting this website. In short, I have a php script that is supposed to automatically submit data to a MySQL database but the data doesn't seem to make it to the database. I am not good at debugging and I am not getting any errors on the pages when I submit an entry.
Basically I have this code which takes in the information and as you know/can tell it calls on another php script to do the auto submit to the database. Here is this code:
</head> <?php include('../templates/bodyandlogo.htm'); ?> <?php include('../templates/navmenu.htm'); ?> <br /> <br /> <h1>PoemScribe: Order Form</h1> <br /> <br /> <p>Please complete the form below and click submit to order your personalized poem.</p> <br /> <br /> <div id="form"> <form action="../php_scripts/orderform.php" method="post"> <label for="firstname">First Name:</label> <div class="input"><input type="text" id="firstname" name="firstname" /></div><br /> <label for="lastname">Last Name:</label> <div class="input"><input type="text" id="lastname" name="lastname" /></div><br /> <label for="email">Email:</label> <div class="input"><input type="text" id="email" name="email" /></div><br /> <label for="selectpoemtype">Select Poem Type:<br /> 4-Line Poem: $15 8-Line Poem: $25 12-Line Poem: $40 16-Line Poem: $50 20-Line Poem: $75 Please type in your desired poem length. Example: "4line". </label><br /> <div class="input"><input type="text" id="selectpoemtype" name="selectpoemtype" /><br /> <br /> <br /> <label for="keynames">"Key names to include?"</label> <div class="input"><input type="text" id="keynames" name="keynames"/></div><br /> <label for="specificwords">Any specific words you would like to include in your poem?</label> <div class="input"><input type="text" id="specificwords" name="specificwords" /></div><br /> <label for="poemisfor">Who is the poem for?</label> <div class="input"><input type="text" id="poemisfor" name="poemisfor" /></div><br /> <label for="relationship">Relationship to you?</label> <div class="input"><input type="text" id="relationship" name="relationship" /></div><br /> <label for="occasion">What is the occasion for this poem?</label> <div class="input"><input type="text" id="occasion" name="occasion" /></div><br /> <label for="overallmessage">What do you want the overall message of this poem to be?</label> <div class="input"><input type="text" id="overallmessage" name="overallmessage" /></div><br /> <label for="anythingelse">Any other information you would like for me to know?</label> <div class="input"><input type="text" id="anythingelse" name="anythingelse" /></div><br /> <div class="input"><input type="submit" value="Submit" name="submit"</div> </form> </div><!--form--> <br /> <br /> <?php include('../templates/footer.htm');
And then the code that is called on (the script called orderform.php):
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en" lang="en"> <head><title>PoemScribe Order Form</title> </head> <body> <h2>PoemScribe</h2> <?php $email = "myemail@gmail.com"; // Email to notify on error $first_name = $_POST['firstname']; $last_name = $_POST['lastname']; $email = $_POST['email']; $select_poem_type = $_POST['selectpoemtype']; $key_names = $_POST['keynames']; $specific_words = $_POST['specificwords']; $poem_is_for = $_POST['poemisfor']; $relationship = $_POST['relationship']; $occasion = $_POST['occasion']; $over_all_message = $_POST['overallmessage']; $anything_else = $_POST['anythingelse']; $dbc = mysqli_connect('localhost', 'login', 'password', 'database'); " . or die('Error connecting to MySQL server.'); $query = INSERT INTO poem_orders '(firstname, lastname, email, selectpoemtype, keynames, specificwords, poemisfor, " . "relationship, occasion, overallmessage, anythingelse)" . "VALUES ('$first_name', '$last_name', '$email', '$select_poem_type' '$key_names', '$specific_words', '$poem_is_for', " . "'$relationship', '$occasion', '$over_all_message', '$anything_else')"; echo 'Thank you, ' . $firstname . $lastname; echo 'Thanks for submitting the form.<br />'; echo ' Poem Type: ' . $selectpoemtype; echo ' Key names to include: ' . $keynames . '<br />'; echo 'Specific Words To Include: '. $specificwords . '<br />'; echo 'Poem is for: ' . $poemisfor . '<br />'; echo 'Relationship: ' . $relationship . '<br />'; echo 'Occasion: ' . $occasion . '<br />'; echo 'Overall Message: ' . $overallmessage . '<br />'; echo 'You also added: ' . $anything_else; mysqli_close($dbc); ?> </body> </html>
Again, I am trying to figure out why my database isn't being populated when someone clicks on the submit button. I tried the code Larry shows on pages 352-354 of this book, but I couldn't get it to work, so I am now trying the code posted above. Any help is appreciated.
Thanks in advance,
Randy
-
Well after conducting a quick test (I know its not been repeated, there are many confounding variables and ultimately means nothing) but using SUBSTRING to select all articles beginning with A from a 7000 row dataset took 0.0291 seconds in comparison to 0.3323 seconds using LIKE. I'm sure I could quite easily produce results to the contrary also, depending on the dataset etc... but to me if you want to extract a row that starts with a single character you should test specifically for that character.
That said if I was really worried about performance and using a huge dataset I'd probably add another column as a foreign key to represent the letters as then it could be indexed.
So maybe my table should have each of the letters of the alphabet added as a subset to the Poems field?
Right now I have id, title, poem, category
Would it be better to organize more by adding all letters A-Z as a subset of "Title"?
Thanks for the help.
-
New thought about your tip:
So I should just have one link on my website like, "browse poems" rather than each individual letter?
And then the "browse poems" link would lead to another page with all the possible poem titles?
-
One tip. Instead of having a.php, b.php, etc. Make one general page called poems.php (or something) and base the query of poems on a $_POST og $_GET-variable. It would make more sense to develope ONE version than 26.
Tip will be used. Thanks.
Question about the MySQL setup. I click the "insert" tab on phpmyadmin to insert the poems, correct? I did this and it looks obvious as to that is what it is for, but i want to be sure.
thank you, randy
-
Thanks for the input. If you are curious, the web page I am working on for this, is: http://www.poemscribe.com/pages/samplepoems.htm
I guess my next step for design is to decide how I am going to make it so that when a visitor presses one of the buttons, a list of poems comes up. I haven't decided how I want to go about this.
Randy
-
Here is a mockup of my layout. I am not sure if it will help you, but you have helped a lot and I don't mind doing a little to make progress:
-
And yes, i forgot the actuall poem.
1. Click on the table poems in phpMyAdmin
2. Select "structure"
3. Look after "Add X column(s) below this structure
4. Select after "title" or what you called it.
5. Click add, call it "poem/text/whatever"
6. Select "Longtext" as type from the drop down list
One question: Is the letters only for sorting alphabetically? Then you could add the Letter directly into the poems table. You could also drop the whole categorization and select only queries that begins with the letter accoring to a variable etc. It depends on what you want.
With categories, you could sort the poems accoring to categories AND letters. It would give you some more choices. I dunno what your looking for.
This is how the data MAY look like:
poems(id, title, poem, category_id)
1 | my title........... | Poem Text | 1
2 | my best friend | Poem Text | 4
3 | my title........... | Poem Text | 2
4 | my title........... | Poem Text | 1
5 | my friend........ | Poem Text | 4
poems_categories (category_id, category_name)
1 | Dreams
2 | Nature
3 | Religious
4 | Frendship
5 | Whatever
Yes, the letters are only for sorting/alphabetizing the poems.
If it is better to see a wireframe/mockup of my plan I can certainly post it.
Thanks again. I will give this a try (what you posted).
-
Excellent. Thank you. I will give your advice a go and see what happens. Thanks again to all for the hand-to-hand guiding to get me started.
-
I really, truly appreciate the direct coaching you folks are giving me. Here is what phpmyadmin printed, when I selected "Print".
poems
Field Type Null Default Comments
id int(11) No
title varchar(255) No
category_id int(3) No
Indexes:
Keyname Type Unique Packed Field Cardinality Collation Null Comment
PRIMARY BTREE Yes No id 0 A
and this snippet was also showing after I created the db:
CREATE TABLE `tuxandp1_poems`.`poems` (
`id` INT NOT NULL AUTO_INCREMENT PRIMARY KEY ,
`title` VARCHAR( 255 ) NOT NULL ,
`category_id` INT( 3 ) NOT NULL
) ENGINE = MYISAM ;
I guess my next step is to ask, How do I enter each of the letters of the alphabet so I may start entering poem titles? Or, is there something else to do first?
Thank you,
Randy
-
Then this would be a good structure:
poems (id, title, category_id*)
- id (primary_key, auto_increment INT)
- title (varchar 255)
- category_id (INT 3) - Foreign key to table poems_category's category_id
poems_category (category_id, category_name)
- categeory_id (primary_key, auto_increment INT 3)
- category_name (varchar 255)
Do you need help generating the code for the structure? I have to go now. Sure someone can help you in the mean tine.
Yes, I would need that help to generate the code. I will, in the meantime, see what I can do with creating a database with my web hosting providers tools.
Thank you, Randy
-
Wow, thanks for the help!
I think the only content I would want to submit, since I write all the poems, would be:
Title, Category.
At the moment I can't think of any other columns for the table.
Thanks again,
Randy
-
I actually would love to use Mysql and my hosting provider has phpmyadmin already for me to use......i simply have never used Mysql and although i looked into it even using larry's php book i jist struggled woth it. If u could provide an example of how to enter my data i would certainly be willing to try agaon. O want to have my database table callwd poems, then letters a to z and just list my poems under each letter. Thank you for the help. Randy
-
Hey everyone,
I am attempting to create an array with my various poem titles that visitors to my website will be able to click on the poem title and read it.
My question is, how do I add the actual poem to this array (this is a short example of my code)?
So far I created the overall array for all the letters to sort with, A, B, C, etc., and the titles of each poem. Do I somehow add a link to the title to make that happen and have the link simply point to another html page? I'm starting to think that an actual poem with many lines doesn't fit into an array. Not sure what to do.
Code:
$poemsa = array ('All Over Town', 'Angel Upon A Breath',);
$poemsb = array ('The Battle of the Bulge');
$poemsc = array ('Choose', 'Coffee Anyone?', 'Coffee History', 'Combustion', 'Crash Test', );
$poemsd = array ('Double Meaning');
//Multidimensional Array
$poems = array(
'A' => $poemsa,
'B' => $poemsb,
'C' => $poemsc,
'D' => $poemsd,
So far I can successfully run these two bits of code:
Code:
print "<p>Poem:<i>{$poems['A'][0]}</i>.</p>";
foreach ($poems as $letter => $poem) {
echo "<p>$letter: $poem</p>\n";
}
Any ideas?
Thank you,
Randy
-
You can do either really. It depends on how long your poems are and how many you have. It would probably be cleaner to use MYSQL rather than having a multitude of potentially long arrays. I would also suggest that using MYSQL would also you to take advantage of a great tool that you may find useful in conjunction with you PHP skills. It isn't solely for inputting data, many people use it just to display items or objects.
Thank you for the feedback. You have convinced me.
-
Hi everyone,
I am currently reading this book, PHP Visual 4th addition. I noticed that PHP can make arrays and multidementional arrays. This is of course new to me. My question revolves around what I am trying to accomplish. What I want to do is allow website visitors to browse my various poems on my site and pick one and so on and maybe browse some more and on and on.
I thought I could make a database with MySQL for a simple list of poems that would be accessible to visitors as they visit my website. I don't know a lot about MySQL except what I read in the last couple of chapters in this book, so my question is this: Should I go ahead and try MySQL or should I just use php to create an array or a multidimensional array for website visitors to access my data with?
I ask because as I don't know a lot about MySQL, I am not sure if it is a tool more suited for website visitors who are expected to enter data(log in and password) as opposed to just accessing my data (poems) and I wasn't sure which (php/MySQL) is best for my task.
Thank you,
Randy
Form Submit Info Not Making It To Database
in PHP for the Web: Visual QuickStart Guide (4th Edition)
Posted
Thank you for taking the time to correct me. I will give this a try tonight.
Randy