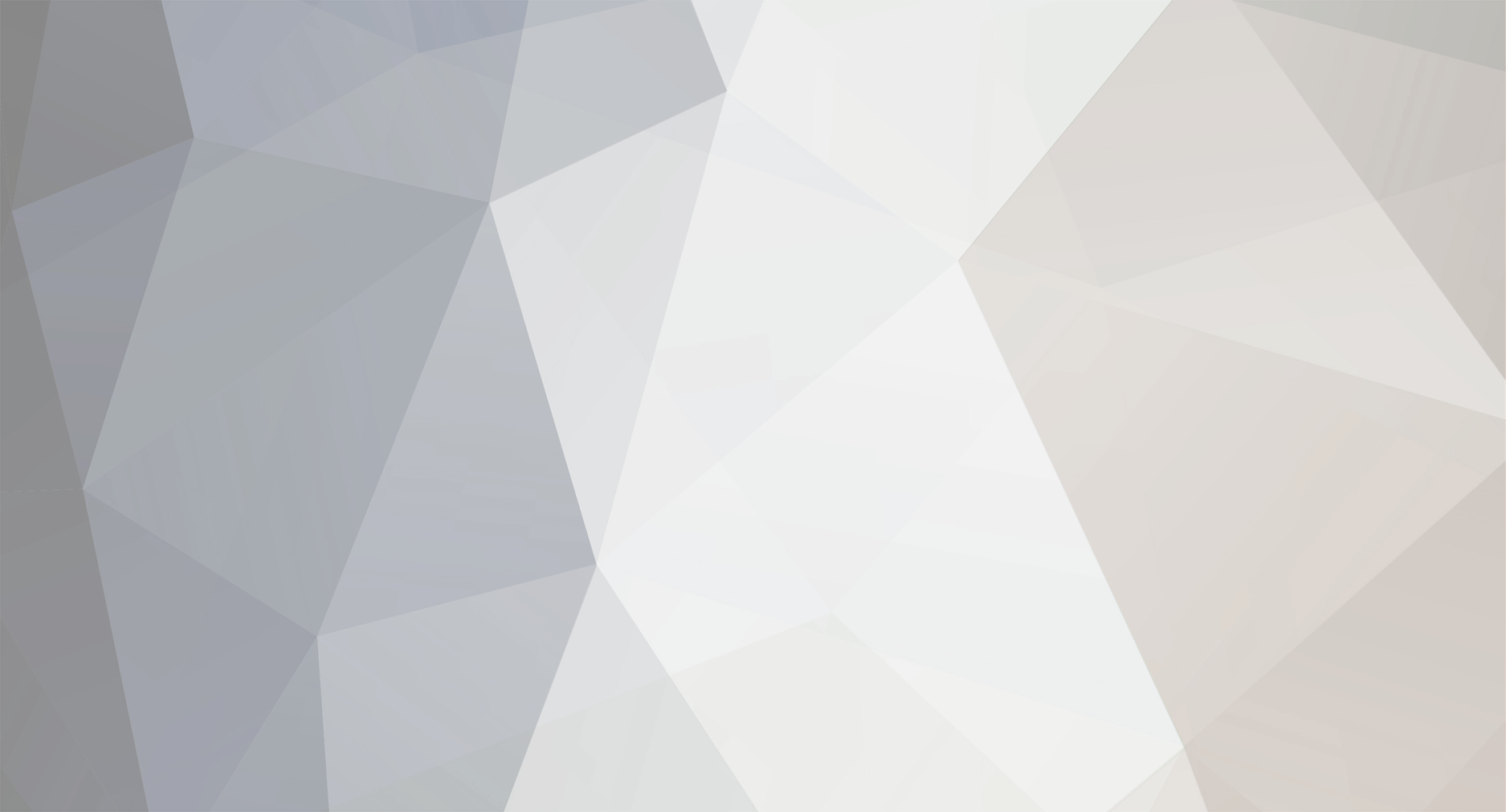
Antonio Conte
Members-
Posts
1084 -
Joined
-
Last visited
-
Days Won
126
Everything posted by Antonio Conte
-
Newsletter #70: Indexing Columns With Null
Antonio Conte replied to DaveB's topic in Newsletter, Blog, and Other Topics
It's bad for performance. Why that is is to technical for me to delve into, but my professor used an hour explaining why during a lesson some years ago. I took the hint... The other reason is because allowing null values is often an indicator of bad db design. If you have columns with lots of null values, you should probably normalize it differently. The way to do it is to add another table and join the data in those cases. An example: Person ( id, firstName, middleName, lastName, ....) The middleName column will obviosly have a lot of null values, because not everyone has a middle name. A way to solve it is to break it up: Person (id, firstName, lastName, ....) MiddleName (id, middleName) When you query, you simply join it: SELECT p.*, m.middleName FROM Person as p LEFT OUTER JOIN MiddleName AS m ON (p.id = m.id) -
Yes, it's object oriented. That doesn't really matter in the end here, though. Both the classes used are part of PHP, so you can drop the exact (updated) code I posted into your own code and use it. You don't have to understand the underlaying logic of DateTime or DateInterval to play with them. This should give you what you want // Paste the function into your code (like below) Or require/include a file with it function get_easter_datetime($year) { $days = easter_days($year); // Create a DateTime object $date = new DateTime("$year-03-21"); // and add the number of days after March 21 // on which Easter falls for the given year $date->add(new DateInterval("P{$days}D")); // Return the result as a unix timestamp return $date->getTimestamp(); } $date = strtotime('09-05-2013'); echo "Date: ".$date."<br />"; // May 9th, 2013 is the effective date of Ascension // The year can be easily extracted from the date $year = date('Y', $date); echo "Year: ".$year."<br />"; // Work with function here $easter = get_easter_datetime($year); // The ascension is 39 days after Easter $ascension = ($easter + (39*86400)); echo "Ascension: ".$ascension."<br />"; echo ($date == $ascension) ? "It's Ascension" : "There is a mistake in the code"; Assuming your logic is sound, this should work perfectly. It's as simple as pasting the function into your project and using get_easter_datetime() instead of easter_days(). Edit: Really sorry. I didn't notice the object was returned rather than a value. I ammended the function to return a unix timestamp instead. Edit 2: Added explanatory comments to the function.
-
Look at the notes in the manual. As it says there, easter_date() is based on the TZ environment variable rather than the default time zone. They do attach a function to solve the problem: /** * Get Unix timestamp for midnight on Easter of a given year * * This function fixes easter_days()'s TZ environment problem */ function get_easter_datetime($year) { $days = easter_days($year); $date = new DateTime("$year-03-21"); $date->add(new DateInterval("P{$days}D")); // Get the correct timestamp from the object return $date->getTimestamp(); } Hope that helps you out.
-
Use the code bbcode at the forum. It looks like this: "<>" and is found between the image and quote icon. It'll improve the readability a lot. Btw, unless you do something special with the class ".hidden", you can use $("something").show(); and .hide(); to get the same effect. If you always want the opposite, you can use .toggle(); An easier way to set the boolean flag: // Get title var filterB = $(this).attr('title'); // Is cameraIcon is clicked ? var iconClicked = (filterB === 'camIcon') ? true : false; Also, I had success getting the id value this way: $(".sunny a").click(function(){ console.log($(this).attr('id')); // You need to have id as a String )}; Good luck.
-
Hey, guys. I have a beginners question here. How do you add data to HTML elements from a source (a db, files, etc) using JavaScript functions found inside a file in a flexible way? I've gathered that you should always wrap your JS in an anonymous self-invoking function, so that functions and variables don't polute the global name space. These functions are then not possible to use moving on. (As is wanted behavior generally) I'll demonstrate... // All JS code from the file myAwesomeJs.js... // This file is loaded using HTML <script...> (function() { var Search = { setSource : function(data) {}; // Does lot's of operations }; Search.setSource(something); // Rechable/in scode... })(); // End of the file myAwesomeJs.js // Here is the page JS <script src="myAwesomeJs.js"></script> <script> $(document).ready(function() { Search.setSource(something); // Undifined. Function not rechable/not in scope... // Could of course place function declaration here... (But I don't want to) }); </script I could of course move the needed function inside the page's JS, inside document ready. I ask because I would like to know how to structure this properly. I'm guessing I need to expose some kind of variable that let's me access the function, but I'm asking because I want to do it correctly the first time. The function will get JSON data that it needs to parse. It should then perform calculations on the data and add elements to several input fields and span tags. myAwesomeJs.js contains several methods that will ease the pain in doing this, so I don't want to duplicate code. That's why I'm asking. Hope this is possible to understand.
-
Both are pretty rare. I've not had a problem that required object copying or cloning yet. Larry can probably think of a case where it would be needed, but I can't currently. As long as you know how to copy, and understand references-vs-values, I would say you are good to go. You'll remember what to do if you ever meet such a problem later on. It's pretty specialized.
-
The most basic and yet most important knowledge to gather here is values versus references. Make sure you understand that first and foremost, as it's very important. $user2 and $user1 references the same object in your example. The variables are simply pointers. Think of the object as a balloon and the variables as Strings tying it to the ground. Both variables references the same balloon, and will change the same balloon. Without any references (variables) a balloon will fly up (be garbage collected) into the atmosphere. Object copying is an edge-case as Jon says. If you need a lot of very similar, but still slightly different objects, this may be such a case where copying can save a lot of resources. On a general basis, you don't really have to think about the problem. As long as you understand the reference-vs-value logic, you're pretty much good to go in most cases.
-
It works because the query builder alters your input to work in several different forms. As long as the input is either: a.) A String that is a DB expression, i.e has parenthesis. COUNT(something)... or b.) Is a String (that will be exploded to an array first thing), or an array with elements that validates a regular expression... ...It will work. All valid elements found in your array will be kept, and the invalid ones removed. If it's an array, all valid elements will be exploded into a single String in the end. The "magic" here is obviously the regular expression, that is smart enough to judge your single array element of several DB columns as valid input. https://github.com/yiisoft/yii/blob/1.1.13/framework/db/CDbCommand.php#L603
-
Yii Application Log Running In Google Chrome
Antonio Conte replied to Edward's topic in The Yii Book
Check out screenshotting sites. Google around, and you'll find many. They'll help you check your design in pretty much every browser you'd like, Even the weird ones. If you need to check JS, Jon can probably provide some insight. -
The error is that your param is $query when it should be $result. To be more specific, the mysqli_fetch_array function needs a result resource. That resource is created by the mysqli query functions. You pass it the query string now, not the needed result resource. Moving forward, If you ever get the same error with Boolean instead of string, then it means your query has errors. Now you are just passing the wrong variable.
- 1 reply
-
- 2
-
-
Nah. I think I'll port the other way around to be honest with you. I've used mostly jQuery for referencing DOM elements so far as I've found it easier. I had no luck with innerHTML or val, so I went for .text(), .val() and .html() instead. I've gotten that to work recently, so I don't really need the jQuery stuff that much now. I have to include it because of Bootstrap anyway, but I'll try to keep my own code independent from any framework. One step at the time, though. About the global state. btns is declared just below NYBG, so it should be local scope. That's an easy miss, though. Thanks anyway.
-
Yeah, it works perfectly. I just updated the original linked script, so you can see the code there. I aso simplified it a bit by adding the IDs directly to the heading and definition list elements. Benefit.js: http://juvenorge.no/dev/benefit.js Script: http://juvenorge.no/dev/prototype.php This is the most relevant, new code: // Calculate data totals function calculateTotals() { // Display total and add-on list total.text("Total " + NYBG.getFormatedTotal() + ",- kr"); list.html(NYBG.getAddOnList()); // Assign values to hidden input fields benefitValue.val(NYBG.getTotal()); benefitList.val(NYBG.getAddOnListAsJSON()); // Attach new button listeners setButtonListeners(); } // Adds button listeners to all buttons function setButtonListeners() { var i, size; btns = document.getElementById('total-list').getElementsByTagName('button'); for (i = 0, size = btns.length; i < size; i+=1) { btns[i].onclick = setClojureForRemoveAddOnAt(i); } } // Closure for removing add-ons on click events function setClojureForRemoveAddOnAt(pos) { return function () { // Remove object from array NYBG.removeAddOnAt(pos); // Calculate values calculateTotals(); }; } The other event listeners simple call calculateTotals() now, and so does the closure. I think it was a very good solution, Jon. I'm wouldn't have thought of this on my own. Thank you very much!
-
I'm familiar with clojures. You should know that Laravel are heavily based on them. That was one of the things that impressed me the most, as some of their solutions result in extremely beautiful code. I'm not really familiar with the DOM yet, though. The definition list is obviously rebuild each time any of the event listeners ran. I only attached the listeners to the buttons when the addOn event was caught. That took me longer to figure out than I care to admit... My solution was to add a new method where everything regarding results was done. I attached the listeners there instead. Thanks, Jon. You rock!
-
Basically, I have problems writing the onclick listener you describe. How can I make the handler differentiate between the buttons, calling the NYBG.removeAddOnAt(POS) function with the position at the button and rebuild the list. I need something along the lines of: (some pseudo-code here) // Target ALL buttons with the class .removeAddOn $(".removeAddOn").click(function(){ alert("1"); // Then I must get the ID... Something along the lines of... // But I'm not really sure how to do it... var id = $(this).id; NYBG.removeAddOnAt(id); // rebuild list with NYBG.getAddOnList() }); But that won't activate when I click on any of the buttons. Once I've bound an event listener to it, I also need a way to get the ID to delete. When that is done, I can simply build the definition list again as you pointed out. Edit: Thank you for the nice words. I'm doing my best.
-
Hey, Guys I'm currently working on car advertisement system. Every add in the system must have a customer benefit for it to be valid. That benefit may be defined as a deducted money amount from the total or as one or more "add-ons" included without charge. Add-ons might be a GPS-system worth $x dollars and Air-condition worth $y dollars. The total customer benefit is summarized from the two, and is thus: Total = deducted_amount + (total_value_of_all_add_ons); The total is set using a total of three input fields. Add-ons: ---> Input 1: Name of the add-on, for example "GPS-system" ---> Input 2: Value of the add-on, for example "1000" Deducted price: ---> Input 3: Fixed deducted amount, for example: "2000" When adding an add-on, the following happens: - The add-on's price is added to the total - An JSON object is added to an array by partsList.push({"part":"GPS", "value="1000"} - An List element is added to a definition list (<dl></dl>) on the form (<dt>GPS</dt><dd>1000 <button.....>Remove</button></dd>). Problems: 1. How do I remove an object from the array by using the "Remove"-button seen right above here? (Calling the function NYBG.removeAddOnAt(POS) with the correct position); 2. How do I remove the definition item from the list when the Remove-button is clicked? See code and the script in action: http://juvenorge.no/dev/prototype.php (Add some items at the top first) Hope someone can help out here.
-
It's been cool following your project. Hit me up if you ever need help withsome CSS. I only did front-end coding & design before Larry pushed PHP knowledge into my stupid brain.
-
I use 1000 rounds Personally. Tweek it to your needs. For me, thats about 0.5 sec in total per calculated hash.
-
It's no shame in declining a job, even if you asked for it. Tell them in a professional way that you are not the perfect fit, wish them good luck and move on to new jobs. Declining the jobs that don't fit you is very important in the start.
-
Yeah, sure. I don't think I will rebuild an already published site with another framework. However, I study computer science, so I might consider doing it as part of learning a new framework - I do have the time for it. More likely, though, I will wait for a new project before diving into new technology.
-
I said a new one. I've actually considered porting this one as I'm part-owner of that particular project. If I decided to do something like that, it has more with learning new tech than really improving something. I'm still a student, so I a lot more time than you guys.
-
I use CodeIgniter too, but I regret building my current project on it. It's a good framework, but it's really starting to show its age. When I have a new project that needs a framework, I'll take a good look at both Laravel and YII 2. I've really liked what I've seen from Laravel, and I found myself nodding a great deal when I studied the public preview readme for YII 2. I think these framework tap into some of the strengths you find in frameworks from other languages, namely Rails, flask and Play!. They also make a lot of sense from a code design standpoint. I'll take a new look around when I need to.