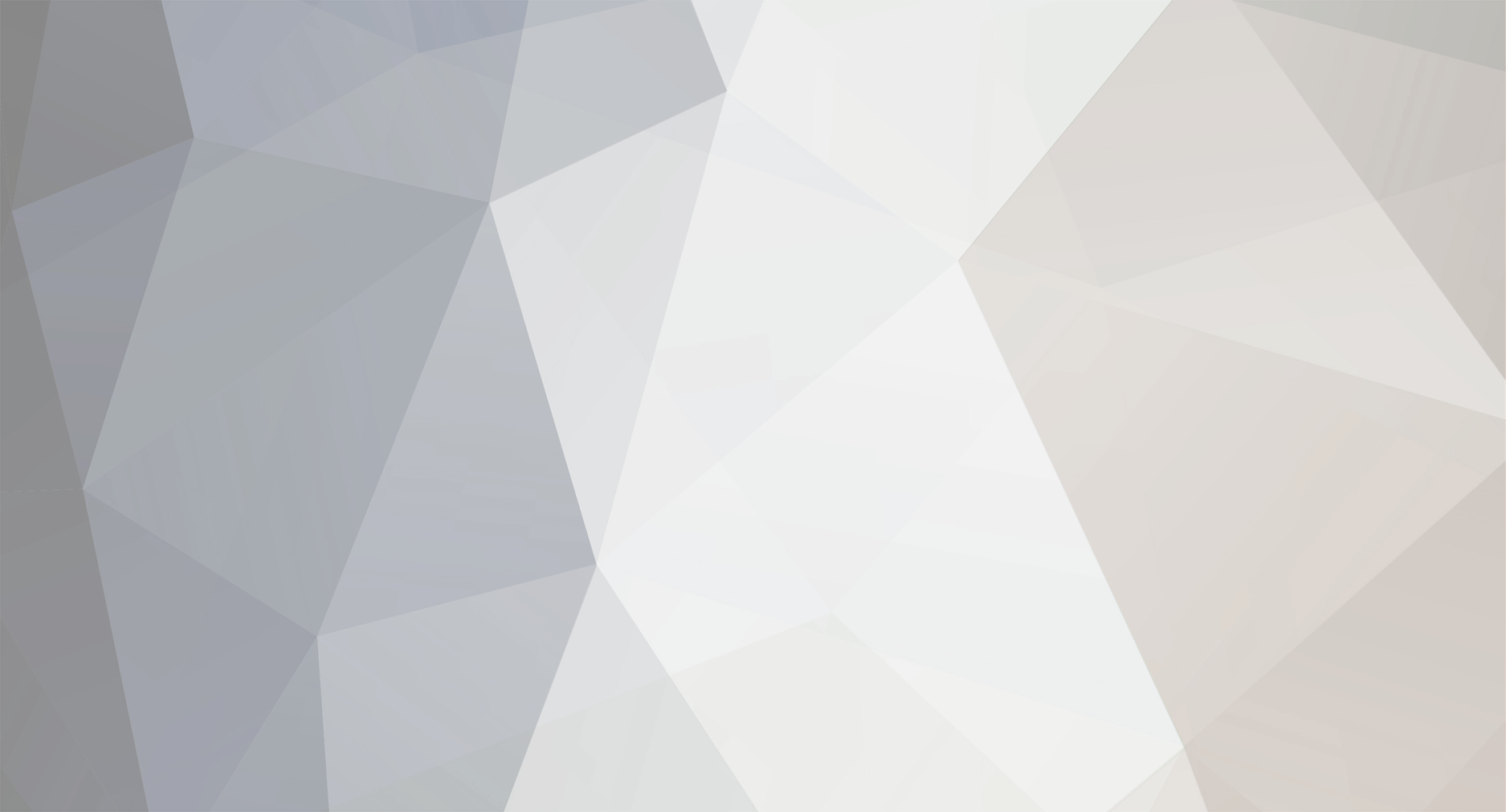
Necuima
Members-
Posts
359 -
Joined
-
Last visited
-
Days Won
7
Everything posted by Necuima
-
C++ with PHP - Possible?
Necuima replied to Necuima's topic in C++ Programming: Visual QuickStart Guide
Hi Larry, Yes, I can access the contents of a file but can I get access to its full path - the application will be running on my PC with PHP via localhost/XAMPP? Thanks, Necuima -
C++ with PHP - Possible?
Necuima replied to Necuima's topic in C++ Programming: Visual QuickStart Guide
Hi Larry, I want the front end to be C++ so that I have access to the PC file system. Apparently it can be done with cpprestsdk and I will check it out. I wonder if I could do it the other way around with PHP on the server calling a C++ module on my PC to get access to the PC file system? I want to access a directory on the PC which, apparently cannot be done with PHP or an HTML form. I want the full path of the directory, not an upload. Re being comfortable with PHP - yes you are right on that count but I'm learning a lot more of C++ particularly using Visual Studio as the IDE which has nice PC forms functionality. Thanks for your reply. Cheers -
C++ with PHP - Possible?
Necuima replied to Necuima's topic in C++ Programming: Visual QuickStart Guide
That should be "I did something like this a few years ago with Adobe AIR and FLEX....". leveraging both your AIR and FLEX books. Cheers -
Hi Larry, Is it possible to have a Windows Form in C++ 'communicate' with a PHP back-end with all functionality being on one PC? I.e., the implementation of PHP in my case would be via XAMPP. Specifically I'd like to have a C++ Windows Form as that has access to the PC file system, and then pass some data from the PC file system to a PHP back-end which would do some work on the file passed to it. Once the work was done then some communication back to the Windows Form front-end to say that the work had been done. I did something like this a few years ago with Adobe AIR as the front-end and PHP as the back end, but would now like to do something similar but with the Windows Form C++ as the font-end. Looking forward to your advice. Cheers from Oz.
-
To avoid compiler warnings change the following: Change 'char jpg_signature' to 'unsigned char jpg_signature' and 'char buff[12]' to 'unsigned char buff[12]'. Tested and still works correctly. The method should probably be named 'checkMimeType'. Cheers
-
Here's some C++ to check if a file is of MIME type 'image/jpeg'. private: String^ GetMimeType(String^ FileName) { // MessageBox::Show("GetMimeType called with " + FileName); const unsigned short JPG_SIGNATURE_LENGTH = 3; char jpg_signature[JPG_SIGNATURE_LENGTH] = { 255, 216, 255 }; // an array of chars with the 'signature' for jpg files char buff[12]; // create a buffer for the first 12 bytes of the file // convert managed string FileName to a standard string // ifstream expects a pointer to a C string (array) of chars char* InputFileName = (char*)Marshal::StringToHGlobalAnsi(FileName).ToPointer(); std::ifstream fileInput(InputFileName, std::ios::binary); // opens the file as a binary file if (fileInput.is_open()) { // MessageBox::Show("fileInput is open for " + FileName); fileInput.read(reinterpret_cast<char *>(&buff), sizeof(buff)); // read the first 12 bytes of the binary input file // now compare the first three bytes for (int i = 0; i < JPG_SIGNATURE_LENGTH; i++) { if (jpg_signature[i] != buff[i]) { fileInput.close(); return "Not jpeg"; // this will break the for loop and exit the method } } // end for loop // the first 3 bytes matched the 'signature' for a jpeg file fileInput.close(); return "image/jpeg"; } else { MessageBox::Show("fileInput open failed for " + FileName); } } // end GetMimeType This is a combination of Visual Studio managed C++ and standard C++. The path of the file is passed to the method. It may be of interest to someone. Cheers from Oz.
-
Hi Larry, Now have it working with a Visual Studio 2017 C++ front-end using the same C sharp DLL. It is very similar. The changes are: using namespace FileTypesLib; FileTypes^ fileTypes = gcnew FileTypes(); if (openFileDialog1->ShowDialog() != System::Windows::Forms::DialogResult::Yes) { // weird!! The negative case is the one that works?? String^ theFileName = openFileDialog1->FileName; // save the file name in the text box this->txtSelectedFile->Text = theFileName; // get the MIME type and save it in the form text box txtMIMEType->Text = fileTypes->GetMimeType(theFileName); } Again, it may be of interest to someone. Cheers
-
But I did spend some more time on it! I leveraged the class library kindly provided by Ed Gadziemski (https://www.codeproject.com/Articles/849083/Determining-File-Type-A-Demonstration-of-Different) and developed a small C# UI front-end in Visual Studio 2017. I used C# as that is what Ed's class library is written in. Here's part of the UI/front-end code: using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; using FileTypesLib; namespace ReadFile { public partial class ReadFileCS : Form { FileTypes fileTypes = new FileTypes(); public ReadFileCS() { InitializeComponent(); } private void Form1_Load(object sender, EventArgs e) { } private void btnSelectFile_Click(object sender, EventArgs e) { var filePath = string.Empty; txtMIMETypeBox.Clear(); using (OpenFileDialog openFileDialog = new OpenFileDialog()) { openFileDialog.InitialDirectory = "e:\\pictures"; openFileDialog.Filter = "image files (*.jpg)|*.jpg|All files (*.*)|*.*"; openFileDialog.FilterIndex = 2; openFileDialog.RestoreDirectory = true; if (openFileDialog.ShowDialog() == DialogResult.OK) { //Get the path of specified file filePath = openFileDialog.FileName; txtSelected_File.Text = filePath; btnSelectFile.Focus(); // get the file's MIME type txtMIMETypeBox.Text = fileTypes.GetMimeType(filePath); } // end dialog box opened OK } // end using (OpenFileDialog openFileDialog = new OpenFileDialog()) } // end private void btnSelectFile_Click(object sender, EventArgs e) private void btnExit_Click(object sender, EventArgs e) { this.Close(); } } // end public partial class ReadFileCS : Form } // end namespace ReadFile It may be of interest to someone! Season's Greetings from Oz.
-
I finally found how to create the variable required which was LPWSTR* and now it compiles OK but won't link. It is more trouble than it is worth but I found one already developed in C# which I might play around with in due course. The solution in C# is from https://www.codeproject.com/Articles/849083/Determining-File-Type-A-Demonstration-of-Different For anyone interested, here's the code to get the variables all in the correct format (Visual Studio 2017 C++17 Windows Form): if (openFileDialog1->ShowDialog() != System::Windows::Forms::DialogResult::Yes) { // weird!! The negative case is the one that works?? //save the file name in the text box this->txtFileName->Text = openFileDialog1->FileName; // see: https://docs.microsoft.com/en-us/previous-versions/windows/internet-explorer/ie-developer/platform-apis/ms775107(v=vs.85) // convert the file name to the form that the FindMimeFromData expects // fopen expects a C string char* InputFileName = (char*)Marshal::StringToHGlobalAnsi(txtFileName->Text).ToPointer(); // now 'looking for' String^ managed_looking_for = L"image/jpeg"; marshal::marshal_context context; // sets the context - needed - see: https://msdn.microsoft.com/en-us/library/bb384865.aspx wchar_t const* c_looking_for = context.marshal_as<wchar_t const*>(managed_looking_for); // now 'out' String^ managed_out = gcnew String(" "); LPWSTR* c_out = (LPWSTR*)Marshal::StringToHGlobalUni(managed_out).ToPointer(); char buff[256]; FILE *in = fopen(InputFileName, "rb"); fread(buff, 1, 256, in); FindMimeFromData(NULL, NULL, buff, 256, c_looking_for, FMFD_DEFAULT, c_out, 0); printf("%ls\n", c_out); } else MessageBox::Show("An error occurred during the file select process - please try again."); } // end file select button clicked Just FYI the linker errors are: unresolved token (0A000B0F) "extern "C" long __stdcall FindMimeFromData(struct IBindCtx *,wchar_t const *,void *,unsigned long,wchar_t const *,unsigned long,wchar_t * *,unsigned long)" (?FindMimeFromData@@$$J232YGJPAUIBindCtx@@PB_WPAXK1KPAPA_WK@Z) referenced in function "private: void __clrcall TestFileType::MyForm::btnSelectFile_Click(class System::Object ^,class System::EventArgs ^)" (?btnSelectFile_Click@MyForm@TestFileType@@$$FA$AAMXP$AAVObject@System@@P$AAVEventArgs@4@@Z) I do not intend to spend any more time on this at the moment! Cheers from Oz.
-
Hi, Larry OK'd the posting of this code example which I eventually got to work. The environment was Windows 10, Visual Studio 2017 with the C++17 language in a Windows Form. I wanted to select a directory that had sub-directories and process jpg files in either the top-level directory or and of its sub-directories. I used recursive_directory_iterator to get access to the directories. Here's the code just FYI in case anyone else is crazy enough like me to want to do this. private: System::Void btnSelectDir_Click(System::Object^ sender, System::EventArgs^ e) { // the 'select the root directory' button was clicked FolderBrowserDialog^ folderBrowserDialog1 = gcnew FolderBrowserDialog; directory_list = gcnew List<String^>(20); // initially allow for 20 entries but the capacity will expand automatically if needed directory_count = 0; total_image_files_count = 0; folderBrowserDialog1->SelectedPath = "E:\\Documents\\"; // adjust as necessary // the following control allows the selection of the input root directory System::Windows::Forms::DialogResult result = folderBrowserDialog1->ShowDialog(); if (result == System::Windows::Forms::DialogResult::OK) { this->txtDirName->Text = folderBrowserDialog1->SelectedPath; selected_root_directory = folderBrowserDialog1->SelectedPath; // selected_root_directory is a managed string // we have selected a root directory OK // convert it to the format required by recursive_directory_iterator marshal::marshal_context context; // sets the context - needed - but don't know why! std::string unmanaged_selected_path = context.marshal_as<std::string>(selected_root_directory); // Managed string to standard string fs::path selected_path = unmanaged_selected_path; // standard string to path // first see if the selected root directory contains any jpg files - if so add it to the list if (the_directory_contains_at_least_one_jpg_file(selected_path)) { // add the directory to the list directory_list->Add(selected_root_directory); // directory_list has (and needs) global scope directory_count++; } // end the selected root directory contained at least one jpg type file // now see if the selected root directory contains any sub-directories for (auto& p : fs::recursive_directory_iterator(selected_path)) { // 'p' is the path data returned from each iteration - it may reference a sub-directory or a file if (fs::is_directory(p.path())) { // we have a directory std::string path_details = p.path().string(); // convert the path details to a standard string String^ managed_path_details = gcnew String(path_details.c_str()); // convert the standard string to a managed string etc... fs was the namespace I set up for the file system. Cheers from Oz.
-
I am going around and around in circles trying to create an LPWSTR (wchar_t*) variable in Visual Studio C++ 2017. MS and Stack Overflow provide lots of examples but I can't get any of them to work! I THINK that I have all the other variables/parameters set OK for the native C 'FindMimeFromData' function. I'll keep experimenting! And if I find a solution I will post it.
-
Hi Larry, I have found a function 'FindMimeFromData' and will see if I can figure out how to use it. Will let you know how I get on. Cheers, Necuima
-
Hi, If anyone has some C++ code to check a file's MIME type, I'd really appreciate a copy or a URL to find it. I am specifically trying to verify that if a file that I am reading (from a Windows file system) has a jpg file suffix, or variants thereof, that the file is indeed of MIME type image/jpeg. The development environment in this instance is Visual Studio 2017. I have scoured Google and Stack Overflow but haven't come up with anything. Many thanks in anticipation, Cheers, Necuima
-
Hi Larry, Have you come across a restriction re access to localhost in the latest version of Windows 10? Prior to the latest version, my XAMPP/localhost scripts were all running perfectly but since the 'upgrade' to the latest version of Windows 10, now there is no access to localhost at all, not even to phpinfo.php. I have tried several different browsers and they all exhibit the same restriction so it seems nothing to do with the browser. I have Googled extensively and it seems that it is a known 'feature' of the latest incarnation of Windows 10 with lots of suggestions on how to deal with the restriction but so far anyway, all the 'fixes' that I have tried are to no avail. Are you aware of this? If so, do you have any advice on how to fix this? Thanks, Necuima
-
Yes, I did not know that the 'with www' and 'without www' environments are separate. What initially threw me was that the session cookie was there in the browser in both situations. I have added some code in the site's .htaccess to remove the www if one is there. Thanks as always for your insights. Cheers from Oz.
-
Hi Larry, I eventually found the root cause. If the initial URL uses the www prefix, the MVC redirect (via index.php) redirects without the www and the issue shows. If the initial URL does not use the www prefix, then all works OK. I think will use .htaccess to remove any wwws if they are there in the initial URL. Looking forward to your insights. Cheers from Oz, Necuima
-
In the end I followed some advice you have given, no doubt many times. I set up a new (temporary) test website using the same hosting folks as the production site. I pared the code right back and implemented it step by step and the problem has 'gone away'! I wish I knew though what the root cause of the problem was, but I've not finished my step-by-step process yet so as I add more and more modules the problem may recur. But at least then I'll know which module was causing the problem. Thanks as always for your advice. Necuima P.S., I am using your model-view-controller architecture as per your 'advanced' book.
-
Re your other suggestion, I can see that the session cookie is getting set on the first try and is still there on the second try and gets deleted properly when I log out. And a new session gets set OK when I then log in again after logging out. A new session cookie is getting set OK without the problem as in this case I have not re-started the browser.
-
Hi Larry, because I keep getting no session data when I test for its presence (the first time) I thought that I could use $GLOBALS instead. I am setting the values in $_SESSION, checking that they are set immediately afterwards, but in the second script my !isset test on the $_SESSION data says that the data are not set the first time I try to log in. But, without closing the browser down, the second time I try to log in I always get in - i.e., the second time the !isset test indicates that the $_SESSION data are indeed set and I can use them OK. I don't understand this at all! Again, your thoughts will be welcomed.
-
Hi Larry, I am having the strangest issue with $_SESSION, which I have used many times before following the guidance in your books, and have never had a problem like this. For several days now I have been trying to debug an issue in a login script in a website. In a nutshell, a $_SESSION gets set in one module but even though I check that the $_SESSION (session_start())starts OK in another module in the same domain, and the $_SESSION (PHPSESSID) cookie is in the browser OK, the $_SESSION data are not there via if (!isset($_SESSION['MGCC_member_data'])) check. BUT if I log in again, without closing the browser down, then everything works fine! As far as I can tell, I have not sent anything to the browser before the $_SESSION data are checked. I have raised this with the hosting folks but would appreciate any insights that you may have. Thanks as always, Necuima
-
Thanks for getting back to me. I think it is something to do with timing - maybe some JS code that runs at the bottom of the module interfering with the setting of the $_SESSION variables. But before I send you a whole bunch of code, can you please help me to understand why $GLOBALS array variables are not getting passed from script to script even though the PHP manual seems to indicate that $GLOBLS are super-globals? In the second script, still within the same domain, I do a dump of the variables but the ones I have set are not in there. $_SERVER; print_r($GLOBALS); The ones following are not there. Here's the setting code: $GLOBALS['MEMBER_KEY'] = $member_key; $GLOBALS['MEMBER_NUMBER'] = $member_number; $GLOBALS['MEMBER_FN'] = $member_first_name; $GLOBALS['MEMBER_LN'] = $member_last_name; Again, any advice will be most appreciated. Thanks, Necuima