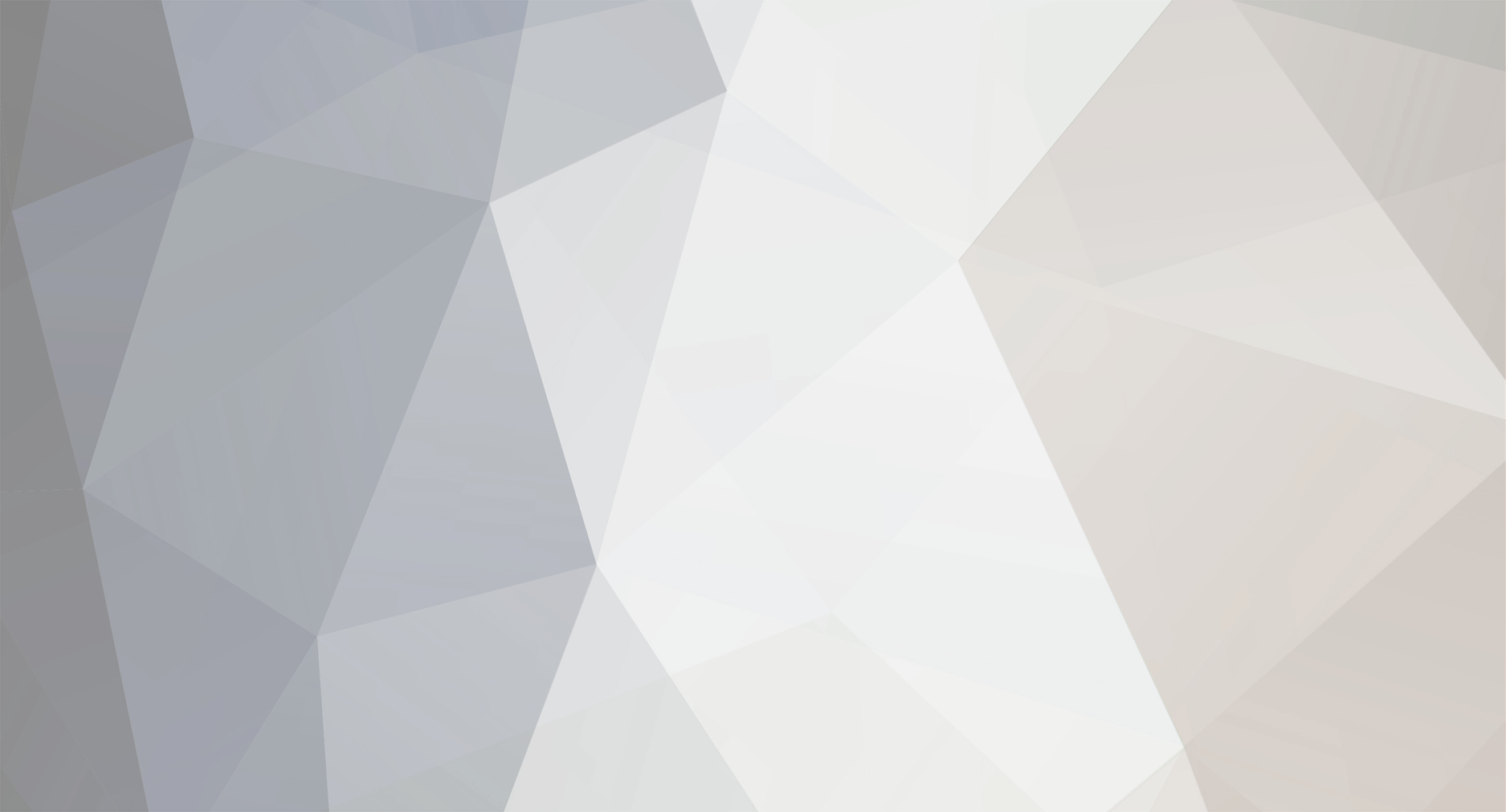
Deaddog
Members-
Posts
47 -
Joined
-
Last visited
Everything posted by Deaddog
-
I think I figured it out now. The solution is probably the PHP equivalent of fixing the car with duct tape, but it really seems to work this time. Making the JOIN was too advanced for me, so I simply made two separate queries. The ruh_id 1006 on line 6 will be the get value depending on what the user selects. So on the live site, that bit of code will look like this: $q2 = "SELECT employee_id FROM ruh WHERE ruh_id = $id"; Otherwise the test code looks like this: <legend>Test Form</legend> <select name="employee_id">'; include(MYSQL); $q = "SELECT employee_id, CONCAT(first_name ,last_name) FROM employee"; $r = mysqli_query($dbc, $q); $q2 = "SELECT employee_id FROM ruh WHERE ruh_id = '1006'"; $r2 = mysqli_query($dbc, $q2); $menu_row_selected = mysqli_fetch_array($r2, MYSQLI_NUM); if (mysqli_num_rows($r) > 0){ while ($menu_row = mysqli_fetch_array($r, MYSQLI_NUM)) { echo '<option value="'.$menu_row[0].'"'; if ($menu_row_selected[0]== $menu_row[0]) echo 'selected'; else echo ''; echo '>'.$menu_row[1].'</option>'; } } mysqli_free_result($r); echo '</select>
-
Yes that's right. The incident creator is probably someone in a workshop somewhere, but the guy entering these reports is updating into the db, sitting in a office someplace. With your help, I managed to get a working code, though maybe not as polished as it could be, it seems to do the trick. Here it is: It was the LEFT JOIN that was the key here. I also used the CONCAT advice. Thanks again. <?php require('includes/config.inc.php'); echo'<form> <fieldset> <legend>Test Form</legend> <select name="employee_id">'; include(MYSQL); $q = "SELECT e.employee_id, r.employee_id, CONCAT(e.first_name ,e.last_name) AS name, r.ruh_id FROM employee AS e LEFT JOIN ruh as r USING(employee_id) ORDER BY e.last_name ASC"; $r = mysqli_query($dbc, $q); $id = 1006; //Get ruh_id for incident. if (mysqli_num_rows($r) > 0){ while ($menu_row = mysqli_fetch_array($r, MYSQLI_NUM)) { if($menu_row[3]== $id){//select the employee that made initial report. echo '<option value="'.$menu_row[0].'" selected="selected">'.$menu_row[2].'</option>'; } if($menu_row[3] != $id) {//list all employees to allow editing, if required. echo '<option value="'.$menu_row[0].'">'.$menu_row[2].'</option>'; } } } mysqli_free_result($r); echo '</select> </fieldset>'; ?>
-
The idea is that the person who enters the report in the db is probably not the actual employee who reports the incident. The db entry will be done by someone in an office somewhere. The initial report will be written on paper and submitted to management. This system will give the office guy the chance to edit who makes the incident report in case a mistake is made on the initial entry. Anyway, I will work on the whileloop and the LEFT JOIN and get back to this post when I figure it all out. Thanks for the help.
-
Hi HartelySan, Let me try to explain it better. If I use the following SQL statement I don't get all the employee_ids in the the employee table: SELECT e.employee_id, r.ruh_id, e.first_name, e.last_name FROM employee AS e INNER JOIN ruh AS r WHERE r.employee_id = e.employee_id; You asked what the ruh_id was. Well, I'm making an incident reporting system and the ruh_id is the report id primary key. Not every employee will make a report, however, if a report is made, the dropdown to edit who made it must contain all the employees in the company (from the employee table) and the one employee (selected) whos report it is to be edited from the ruh table. The following (working) drop down returns all the employees in employee table and this is what I want to happen. However, it lacks the ability to preselect the current employee_id foriegn key in the ruh table for that particurly ruh_id. <?php require('includes/config.inc.php'); echo'<form> <fieldset> <legend>Test Form</legend> <select name="employee_id">'; include(MYSQL); $q = "SELECT employee_id, first_name, last_name FROM employee"; $r = mysqli_query($dbc, $q); if (mysqli_num_rows($r) > 0) { while ($menu_row = mysqli_fetch_array($r, MYSQLI_NUM)) { echo '<option value="'.$menu_row[0].'">'.$menu_row[1].' ' . $menu_row[2] . '</option>\n'; } } mysqli_free_result($r); echo '</select> </fieldset>'; ?> I was thinking maybe I need a foreach statement. I hope this makes it clearer.
-
Hi. I've managed to dig myself down into a muddled mess of ugly dropdown code and need some help. In plain english, this is what I want to happen: I have a table called employee which has a primary key of employee_id and a table called ruh which has a primary key called ruh_id and a foreign key called employee_id. I'm trying to make a select menu to update the employee_id on the ruh table. I would like the dropdown to return every employee_id, first_name, last_name from employee table and then select the foreign key employee_id from ruh table for the one value that should be selected. In this example, the one value should be employee_id of 68 when ruh_id =1003 . The end result should be a dropdown menu filled with all the employee_ids from the employee table and with 68 selected. The pupose of this is to allow the user to update the employee_id on the ruh table with any employee_id from the employee table. I hope it makes sense. I've been trying to figure this out for many hours and am slowly going insane. Help is appreciated. I found something similar here: http://www.larryullman.com/forums/index.php?/topic/1944-making-drop-down-date-values-sticky/?hl=selected <?php require('includes/config.inc.php'); require(MYSQL); $q = "SELECT e.employee_id, r.employee_id, e.first_name, e.last_name FROM employee AS e LEFT JOIN ruh AS r"; $r = @mysqli_query ($dbc, $q); while ($row = mysqli_fetch_array($r, MYSQLI_NUM)) { $ruh_id = 1003; // $employee_id = $row[0]; } echo '<label>Employee</label> <select name="employee_id">'; foreach ($employee_id as $value) { echo '<option value="' . $value . '"'; if ($value === $ruh_id) { echo ' selected="selected"'; } echo '>' . $value . '</option>'; } echo '</select>'; ?>
-
It's a happy day. That did it. I'm sure the code is probably rough around the edges to all you experienced folks, but it works. Here's a snippet. I'm sure there is a cleaner way to make the foreach() statements, but for now, it's fantastic. As Larry wrote in the last post, qual_id should have been qual_id[]. I used this same approach on the other values, i.e. employee_id[], etc... I know it takes a lot of effort to answer these forums posts, so thanks again for the help to Larry and HartleySan for this one. // Create the submission conditional and initialize the $errors array. if ($_SERVER['REQUEST_METHOD'] == 'POST'){ // Add the MySQL connection include(MYSQL); $errors = array(); foreach($_POST['qual_id'] as $row=>$qual_id) foreach($_POST['employee_id'] as $row=>$employee_id) foreach($_POST['issue_date'] as $row=>$issue_date) foreach($_POST['expiration_date'] as $row=>$expiration_date) { $qual_id=mysqli_real_escape_string($dbc, trim($qual_id)); $employee_id=mysqli_real_escape_string($dbc, trim($employee_id)); $issue_date=mysqli_real_escape_string($dbc, trim($issue_date)); $expiration_date=mysqli_real_escape_string($dbc, trim($expiration_date)); $query_row[] = "('$qual_id','$employee_id','$issue_date', '$expiration_date')"; } if (empty($errors)) { print_r($_POST['qual_id']); $q= "INSERT INTO emp_quals(qual_id, employee_id, issue_date, expiration_date) VALUES " . implode(", ", $query_row);
-
Ok. I've done it. This is the result: 4. It echos the qual_id. You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use near '' at line 1 Query: INSERT INTO emp_quals(qual_id, employee_id, issue_date, expiration_date) VALUES 4 The input form (multiple select) for qual_id looks like this: <label>Kvalifikasjon:</label><select multiple name="qual_id">'; <?php include(MYSQL); $q = "SELECT qual_id, qual_name FROM quals ORDER BY qual_id ASC"; $r = mysqli_query($dbc, $q); if (mysqli_num_rows($r) > 0) { while ($menu_row = mysqli_fetch_array($r, MYSQLI_NUM)) { echo '<option value="'.$menu_row[0].'">'.$menu_row[0].' - ' . $menu_row[1] . '</option>\n'; } } mysqli_free_result($r); echo '</select><br /><small>Bruk Ctrl knappen for å velge flere.</small><br />'; ?>
-
Thanks HartleySan, I've been to the PHP manual, in fact it is the first place I checked for advice. The specific part of the code that is giving problems is below. If it wasn't for the foreach statement maybe I could have figured it out by now. Since each qual_id must be a new row on the emp_quals table, it's tricky. if (empty($errors)) { print_r($_POST); foreach($_POST['qual_id'] as $row=>$qual_id) { $qual_id=mysqli_real_escape_string($dbc, trim($qual_id)); $employee_id=mysqli_real_escape_string($dbc, trim($_POST['employee_id'][$row])); $issue_date=mysqli_real_escape_string($dbc, trim($_POST['issue_date'][$row])); $expiration_date=mysqli_real_escape_string($dbc, trim($_POST['expiration_date'][$row])); $query_row[] = "('$qual_id','$employee_id','$issue_date', '$expiration_date')"; } $q= "INSERT INTO emp_quals(qual_id, employee_id, issue_date, expiration_date) VALUES " . implode(',',$query_row);
-
If there's one thing I have plenty of, it's debugging information . The problem is I don't understand it all. I have Scipt 18.3 debugging for !LIVE site. if (!LIVE) { //Development (print the error). //snippet from script 18.3. //Show the error message: echo '<div class"error">' . nl2br($message); // Add the variables and a backtrace: echo '<pre>' . print_r($e_vars, 1). "\n"; debug_print_backtrace(); echo '</pre></div>'; Below is the debugging info that was generated by script 18.3 - config.inc.php. <?php Array ( [qual_id] => 4 [employee_id] => 6 [issue_date] => [expiration_date] => [Submit] => Submit ) An error occurred in script 'C:\wamp\www\******\set_qual.php' on line 37: Invalid argument supplied for foreach() Date/Time: 3-23-2013 23:07:09 Array ( [GLOBALS] => Array *RECURSION* [_POST] => Array ( [qual_id] => 4 [employee_id] => 6 [issue_date] => [expiration_date] => [Submit] => Submit ) [_GET] => Array ( ) [_COOKIE] => Array ( [__utma] => 111872281.652457305.1361574854.1362520724.1362599419.29 [__utmz] => 111872281.1361574854.1.1.utmcsr=(direct)|utmccn=(direct)|utmcmd=(none) [PHPSESSID] => ****** ) [_FILES] => Array ( ) [_ENV] => Array ( ) [_REQUEST] => Array ( [qual_id] => 4 [employee_id] => 6 [issue_date] => [expiration_date] => [Submit] => Submit ) [_SERVER] => Array ( [HTTP_HOST] => localhost [HTTP_USER_AGENT] => Mozilla/5.0 (Windows NT 5.1; rv:19.0) Gecko/20100101 Firefox/19.0 [HTTP_ACCEPT] => text/html,application/xhtml+xml,application/xml;q=0.9,*/*;q=0.8 [HTTP_ACCEPT_LANGUAGE] => en-US,en;q=0.5 [HTTP_ACCEPT_ENCODING] => gzip, deflate [HTTP_REFERER] => http://localhost/******/set_qual.php [HTTP_COOKIE] => __utma=111872281.652457305.1361574854.1362520724.1362599419.29; __utmz=111872281.1361574854.1.1.utmcsr=(direct)|utmccn=(direct)|utmcmd=(none); PHPSESSID=****** [HTTP_CONNECTION] => keep-alive [CONTENT_TYPE] => application/x-www-form-urlencoded [CONTENT_LENGTH] => 86 [PATH] => C:\**** [SystemRoot] => C:\WINDOWS [COMSPEC] => C:\WINDOWS\system32\cmd.exe [PATHEXT] => .COM;.EXE;.BAT;.CMD;.VBS;.VBE;.JS;.JSE;.WSF;.WSH [WINDIR] => C:\WINDOWS [SERVER_SIGNATURE] => [SERVER_SOFTWARE] => Apache/2.2.21 (Win32) mod_ssl/2.2.21 OpenSSL/0.9.8r PHP/5.3.8 [SERVER_NAME] => localhost [SERVER_ADDR] => 127.0.0.1 [SERVER_PORT] => 80 [REMOTE_ADDR] => 127.0.0.1 [DOCUMENT_ROOT] => C:/wamp/www/ [SERVER_ADMIN] => admin@localhost [SCRIPT_FILENAME] => C:/wamp/www/******/set_qual.php [REMOTE_PORT] => 1202 [GATEWAY_INTERFACE] => CGI/1.1 [SERVER_PROTOCOL] => HTTP/1.1 [REQUEST_METHOD] => POST [QUERY_STRING] => [REQUEST_URI] => /******/set_qual.php [SCRIPT_NAME] => /******/set_qual.php [PHP_SELF] => /******/set_qual.php [REQUEST_TIME] => 1364076429 ) [page_title] => Ny Kvalifikasjon for ansatte! [thismonth] => March [_SESSION] => Array ( [user_id] => 1 [first_name] => Jason [user_level] => 0 ) [dbc] => mysqli Object ( [affected_rows] => 0 [client_info] => mysqlnd 5.0.8-dev - 20102224 - $Revision: 310735 $ [client_version] => 50008 [connect_errno] => 0 [connect_error] => [errno] => 0 [error] => [field_count] => 0 [host_info] => localhost via TCP/IP [info] => [insert_id] => 0 [server_info] => 5.5.16-log [server_version] => 50516 [sqlstate] => 00000 [protocol_version] => 10 [thread_id] => 5 [warning_count] => 0 ) [errors] => Array ( ) [qual_id] => 4 [employee_id] => 6 [issue_date] => [expiration_date] => ) #0 my_error_handler(2, Invalid argument supplied for foreach(), C:\wamp\www\******\set_qual.php, 37, Array ([GLOBALS] => Array ( *RECURSION*,[_POST] => Array ([qual_id] => 4,[employee_id] => 6,[issue_date] => ,[expiration_date] => ,[Submit] => Submit),[_GET] => Array (),[_COOKIE] => Array ([__utma] => 111872281.652457305.1361574854.1362520724.1362599419.29,[__utmz] => 111872281.1361574854.1.1.utmcsr=(direct)|utmccn=(direct)|utmcmd=(none),[PHPSESSID] => ******),[_FILES] => Array (),[_ENV] => Array (),[_REQUEST] => Array ([qual_id] => 4,[employee_id] => 6,[issue_date] => ,[expiration_date] => ,[Submit] => Submit),[_SERVER] => Array ([HTTP_HOST] => localhost,[HTTP_USER_AGENT] => Mozilla/5.0 (Windows NT 5.1; rv:19.0) Gecko/20100101 Firefox/19.0,[HTTP_ACCEPT] => text/html,application/xhtml+xml,application/xml;q=0.9,*/*;q=0.8,[HTTP_ACCEPT_LANGUAGE] => en-US,en;q=0.5,[HTTP_ACCEPT_ENCODING] => gzip, deflate,[HTTP_REFERER] => http://localhost/******/set_qual.php,[HTTP_COOKIE] => __utma=111872281.652457305.1361574854.1362520724.1362599419.29; __utmz=111872281.1361574854.1.1.utmcsr=(direct)|utmccn=(direct)|utmcmd=(none); PHPSESSID=******,[HTTP_CONNECTION] => keep-alive,[CONTENT_TYPE] => application/x-www-form-urlencoded,[CONTENT_LENGTH] => 86,[PATH] => C:\***** [SystemRoot] => C:\WINDOWS,[COMSPEC] => C:\WINDOWS\system32\cmd.exe,[PATHEXT] => .COM;.EXE;.BAT;.CMD;.VBS;.VBE;.JS;.JSE;.WSF;.WSH,[WINDIR] => C:\WINDOWS,[SERVER_SIGNATURE] => ,[SERVER_SOFTWARE] => Apache/2.2.21 (Win32) mod_ssl/2.2.21 OpenSSL/0.9.8r PHP/5.3.8,[SERVER_NAME] => localhost,[SERVER_ADDR] => 127.0.0.1,[SERVER_PORT] => 80,[REMOTE_ADDR] => 127.0.0.1,[DOCUMENT_ROOT] => C:/wamp/www/,[SERVER_ADMIN] => admin@localhost,[SCRIPT_FILENAME] => C:/wamp/www/******/set_qual.php,[REMOTE_PORT] => 1202,[GATEWAY_INTERFACE] => CGI/1.1,[SERVER_PROTOCOL] => HTTP/1.1,[REQUEST_METHOD] => POST,[QUERY_STRING] => ,[REQUEST_URI] => /******/set_qual.php,[SCRIPT_NAME] => /******/set_qual.php,[PHP_SELF] => /******/set_qual.php,[REQUEST_TIME] => 1364076429),[page_title] => Ny Kvalifikasjon for ansatte!,[thismonth] => March,[_SESSION] => Array ([user_id] => 1,[first_name] => Jason,[user_level] => 0),[dbc] => mysqli Object ([affected_rows] => ,[client_info] => ,[client_version] => ,[connect_errno] => ,[connect_error] => ,[errno] => ,[error] => ,[field_count] => ,[host_info] => ,[info] => ,[insert_id] => ,[server_info] => ,[server_version] => ,[sqlstate] => ,[protocol_version] => ,[thread_id] => ,[warning_count] => ),[errors] => Array (),[qual_id] => 4,[employee_id] => 6,[issue_date] => ,[expiration_date] => )) called at [C:\wamp\www\******\set_qual.php:37] An error occurred in script 'C:\wamp\www\******\set_qual.php' on line 46: Undefined variable: query_row Date/Time: 3-23-2013 23:07:09 Array ( [GLOBALS] => Array *RECURSION* [_POST] => Array ( [qual_id] => 4 [employee_id] => 6 [issue_date] => [expiration_date] => [Submit] => Submit ) [_GET] => Array ( ) [_COOKIE] => Array ( [__utma] => 111872281.652457305.1361574854.1362520724.1362599419.29 [__utmz] => 111872281.1361574854.1.1.utmcsr=(direct)|utmccn=(direct)|utmcmd=(none) [PHPSESSID] => ****** ) [_FILES] => Array ( ) [_ENV] => Array ( ) [_REQUEST] => Array ( [qual_id] => 4 [employee_id] => 6 [issue_date] => [expiration_date] => [Submit] => Submit ) [_SERVER] => Array ( [HTTP_HOST] => localhost [HTTP_USER_AGENT] => Mozilla/5.0 (Windows NT 5.1; rv:19.0) Gecko/20100101 Firefox/19.0 [HTTP_ACCEPT] => text/html,application/xhtml+xml,application/xml;q=0.9,*/*;q=0.8 [HTTP_ACCEPT_LANGUAGE] => en-US,en;q=0.5 [HTTP_ACCEPT_ENCODING] => gzip, deflate [HTTP_REFERER] => http://localhost/******/set_qual.php [HTTP_COOKIE] => __utma=111872281.652457305.1361574854.1362520724.1362599419.29; __utmz=111872281.1361574854.1.1.utmcsr=(direct)|utmccn=(direct)|utmcmd=(none); PHPSESSID=***** [HTTP_CONNECTION] => keep-alive [CONTENT_TYPE] => application/x-www-form-urlencoded [CONTENT_LENGTH] => 86 [PATH] => C:\**** [SystemRoot] => C:\WINDOWS [COMSPEC] => C:\WINDOWS\system32\cmd.exe [PATHEXT] => .COM;.EXE;.BAT;.CMD;.VBS;.VBE;.JS;.JSE;.WSF;.WSH [WINDIR] => C:\WINDOWS [SERVER_SIGNATURE] => [SERVER_SOFTWARE] => Apache/2.2.21 (Win32) mod_ssl/2.2.21 OpenSSL/0.9.8r PHP/5.3.8 [SERVER_NAME] => localhost [SERVER_ADDR] => 127.0.0.1 [SERVER_PORT] => 80 [REMOTE_ADDR] => 127.0.0.1 [DOCUMENT_ROOT] => C:/wamp/www/ [SERVER_ADMIN] => admin@localhost [SCRIPT_FILENAME] => C:/wamp/www/******/set_qual.php [REMOTE_PORT] => 1202 [GATEWAY_INTERFACE] => CGI/1.1 [SERVER_PROTOCOL] => HTTP/1.1 [REQUEST_METHOD] => POST [QUERY_STRING] => [REQUEST_URI] => /******/set_qual.php [SCRIPT_NAME] => /******/set_qual.php [PHP_SELF] => /******/set_qual.php [REQUEST_TIME] => 1364076429 ) [page_title] => Ny Kvalifikasjon for ansatte! [thismonth] => March [_SESSION] => Array ( [user_id] => 1 [first_name] => Jason [user_level] => 0 ) [dbc] => mysqli Object ( [affected_rows] => 0 [client_info] => mysqlnd 5.0.8-dev - 20102224 - $Revision: 310735 $ [client_version] => 50008 [connect_errno] => 0 [connect_error] => [errno] => 0 [error] => [field_count] => 0 [host_info] => localhost via TCP/IP [info] => [insert_id] => 0 [server_info] => 5.5.16-log [server_version] => 50516 [sqlstate] => 00000 [protocol_version] => 10 [thread_id] => 5 [warning_count] => 0 ) [errors] => Array ( ) [qual_id] => 4 [employee_id] => 6 [issue_date] => [expiration_date] => ) #0 my_error_handler(8, Undefined variable: query_row, C:\wamp\www\******\set_qual.php, 46, Array ([GLOBALS] => Array ( *RECURSION*,[_POST] => Array ([qual_id] => 4,[employee_id] => 6,[issue_date] => ,[expiration_date] => ,[Submit] => Submit),[_GET] => Array (),[_COOKIE] => Array ([__utma] => 111872281.652457305.1361574854.1362520724.1362599419.29,[__utmz] => 111872281.1361574854.1.1.utmcsr=(direct)|utmccn=(direct)|utmcmd=(none),[PHPSESSID] => ******),[_FILES] => Array (),[_ENV] => Array (),[_REQUEST] => Array ([qual_id] => 4,[employee_id] => 6,[issue_date] => ,[expiration_date] => ,[Submit] => Submit),[_SERVER] => Array ([HTTP_HOST] => localhost,[HTTP_USER_AGENT] => Mozilla/5.0 (Windows NT 5.1; rv:19.0) Gecko/20100101 Firefox/19.0,[HTTP_ACCEPT] => text/html,application/xhtml+xml,application/xml;q=0.9,*/*;q=0.8,[HTTP_ACCEPT_LANGUAGE] => en-US,en;q=0.5,[HTTP_ACCEPT_ENCODING] => gzip, deflate,[HTTP_REFERER] => http://localhost/******/set_qual.php,[HTTP_COOKIE] => __utma=111872281.652457305.1361574854.1362520724.1362599419.29; __utmz=111872281.1361574854.1.1.utmcsr=(direct)|utmccn=(direct)|utmcmd=(none); PHPSESSID=******,[HTTP_CONNECTION] => keep-alive,[CONTENT_TYPE] => application/x-www-form-urlencoded,[CONTENT_LENGTH] => 86,[PATH] => C:\***** C:\WINDOWS\system32\cmd.exe,[PATHEXT] => .COM;.EXE;.BAT;.CMD;.VBS;.VBE;.JS;.JSE;.WSF;.WSH,[WINDIR] => C:\WINDOWS,[SERVER_SIGNATURE] => ,[SERVER_SOFTWARE] => Apache/2.2.21 (Win32) mod_ssl/2.2.21 OpenSSL/0.9.8r PHP/5.3.8,[SERVER_NAME] => localhost,[SERVER_ADDR] => 127.0.0.1,[SERVER_PORT] => 80,[REMOTE_ADDR] => 127.0.0.1,[DOCUMENT_ROOT] => C:/wamp/www/,[SERVER_ADMIN] => admin@localhost,[SCRIPT_FILENAME] => C:/wamp/www/******/set_qual.php,[REMOTE_PORT] => 1202,[GATEWAY_INTERFACE] => CGI/1.1,[SERVER_PROTOCOL] => HTTP/1.1,[REQUEST_METHOD] => POST,[QUERY_STRING] => ,[REQUEST_URI] => /******/set_qual.php,[SCRIPT_NAME] => /******/set_qual.php,[PHP_SELF] => /******/set_qual.php,[REQUEST_TIME] => 1364076429),[page_title] => Ny Kvalifikasjon for ansatte!,[thismonth] => March,[_SESSION] => Array ([user_id] => 1,[first_name] => Jason,[user_level] => 0),[dbc] => mysqli Object ([affected_rows] => ,[client_info] => ,[client_version] => ,[connect_errno] => ,[connect_error] => ,[errno] => ,[error] => ,[field_count] => ,[host_info] => ,[info] => ,[insert_id] => ,[server_info] => ,[server_version] => ,[sqlstate] => ,[protocol_version] => ,[thread_id] => ,[warning_count] => ),[errors] => Array (),[qual_id] => 4,[employee_id] => 6,[issue_date] => ,[expiration_date] => )) called at [C:\wamp\www\******\set_qual.php:46] An error occurred in script 'C:\wamp\www\******\set_qual.php' on line 46: implode() [function.implode]: Invalid arguments passed Date/Time: 3-23-2013 23:07:09 Array ( [GLOBALS] => Array *RECURSION* [_POST] => Array ( [qual_id] => 4 [employee_id] => 6 [issue_date] => [expiration_date] => [Submit] => Submit ) [_GET] => Array ( ) [_COOKIE] => Array ( [__utma] => 111872281.652457305.1361574854.1362520724.1362599419.29 [__utmz] => 111872281.1361574854.1.1.utmcsr=(direct)|utmccn=(direct)|utmcmd=(none) [PHPSESSID] => ******* ) [_FILES] => Array ( ) [_ENV] => Array ( ) [_REQUEST] => Array ( [qual_id] => 4 [employee_id] => 6 [issue_date] => [expiration_date] => [Submit] => Submit ) [_SERVER] => Array ( [HTTP_HOST] => localhost [HTTP_USER_AGENT] => Mozilla/5.0 (Windows NT 5.1; rv:19.0) Gecko/20100101 Firefox/19.0 [HTTP_ACCEPT] => text/html,application/xhtml+xml,application/xml;q=0.9,*/*;q=0.8 [HTTP_ACCEPT_LANGUAGE] => en-US,en;q=0.5 [HTTP_ACCEPT_ENCODING] => gzip, deflate [HTTP_REFERER] => http://localhost/******/set_qual.php [HTTP_COOKIE] => __utma=111872281.652457305.1361574854.1362520724.1362599419.29; __utmz=111872281.1361574854.1.1.utmcsr=(direct)|utmccn=(direct)|utmcmd=(none); PHPSESSID=****** [HTTP_CONNECTION] => keep-alive [CONTENT_TYPE] => application/x-www-form-urlencoded [CONTENT_LENGTH] => 86 [PATH] => C:\**** [SystemRoot] => C:\WINDOWS [COMSPEC] => C:\WINDOWS\system32\cmd.exe [PATHEXT] => .COM;.EXE;.BAT;.CMD;.VBS;.VBE;.JS;.JSE;.WSF;.WSH [WINDIR] => C:\WINDOWS [SERVER_SIGNATURE] => [SERVER_SOFTWARE] => Apache/2.2.21 (Win32) mod_ssl/2.2.21 OpenSSL/0.9.8r PHP/5.3.8 [SERVER_NAME] => localhost [SERVER_ADDR] => 127.0.0.1 [SERVER_PORT] => 80 [REMOTE_ADDR] => 127.0.0.1 [DOCUMENT_ROOT] => C:/wamp/www/ [SERVER_ADMIN] => admin@localhost [SCRIPT_FILENAME] => C:/wamp/www/******/set_qual.php [REMOTE_PORT] => 1202 [GATEWAY_INTERFACE] => CGI/1.1 [SERVER_PROTOCOL] => HTTP/1.1 [REQUEST_METHOD] => POST [QUERY_STRING] => [REQUEST_URI] => /******/set_qual.php [SCRIPT_NAME] => /******/set_qual.php [PHP_SELF] => /******/set_qual.php [REQUEST_TIME] => 1364076429 ) [page_title] => Ny Kvalifikasjon for ansatte! [thismonth] => March [_SESSION] => Array ( [user_id] => 1 [first_name] => Jason [user_level] => 0 ) [dbc] => mysqli Object ( [affected_rows] => 0 [client_info] => mysqlnd 5.0.8-dev - 20102224 - $Revision: 310735 $ [client_version] => 50008 [connect_errno] => 0 [connect_error] => [errno] => 0 [error] => [field_count] => 0 [host_info] => localhost via TCP/IP [info] => [insert_id] => 0 [server_info] => 5.5.16-log [server_version] => 50516 [sqlstate] => 00000 [protocol_version] => 10 [thread_id] => 5 [warning_count] => 0 ) [errors] => Array ( ) [qual_id] => 4 [employee_id] => 6 [issue_date] => [expiration_date] => ) #0 my_error_handler(2, implode() [function.implode]: Invalid arguments passed, C:\wamp\www\******\set_qual.php, 46, Array ([GLOBALS] => Array ( *RECURSION*,[_POST] => Array ([qual_id] => 4,[employee_id] => 6,[issue_date] => ,[expiration_date] => ,[Submit] => Submit),[_GET] => Array (),[_COOKIE] => Array ([__utma] => 111872281.652457305.1361574854.1362520724.1362599419.29,[__utmz] => 111872281.1361574854.1.1.utmcsr=(direct)|utmccn=(direct)|utmcmd=(none),[PHPSESSID] => ******),[_FILES] => Array (),[_ENV] => Array (),[_REQUEST] => Array ([qual_id] => 4,[employee_id] => 6,[issue_date] => ,[expiration_date] => ,[Submit] => Submit),[_SERVER] => Array ([HTTP_HOST] => localhost,[HTTP_USER_AGENT] => Mozilla/5.0 (Windows NT 5.1; rv:19.0) Gecko/20100101 Firefox/19.0,[HTTP_ACCEPT] => text/html,application/xhtml+xml,application/xml;q=0.9,*/*;q=0.8,[HTTP_ACCEPT_LANGUAGE] => en-US,en;q=0.5,[HTTP_ACCEPT_ENCODING] => gzip, deflate,[HTTP_REFERER] => http://localhost/******/set_qual.php,[HTTP_COOKIE] => __utma=111872281.652457305.1361574854.1362520724.1362599419.29; __utmz=111872281.1361574854.1.1.utmcsr=(direct)|utmccn=(direct)|utmcmd=(none); PHPSESSID=*****,[HTTP_CONNECTION] => keep-alive,[CONTENT_TYPE] => application/x-www-form-urlencoded,[CONTENT_LENGTH] => 86,[PATH] => C:******** CGI/1.1,[SERVER_PROTOCOL] => HTTP/1.1,[REQUEST_METHOD] => POST,[QUERY_STRING] => ,[REQUEST_URI] => /******/set_qual.php,[SCRIPT_NAME] => /******/set_qual.php,[PHP_SELF] => /******/set_qual.php,[REQUEST_TIME] => 1364076429),[page_title] => Ny Kvalifikasjon for ansatte!,[thismonth] => March,[_SESSION] => Array ([user_id] => 1,[first_name] => Jason,[user_level] => 0),[dbc] => mysqli Object ([affected_rows] => ,[client_info] => ,[client_version] => ,[connect_errno] => ,[connect_error] => ,[errno] => ,[error] => ,[field_count] => ,[host_info] => ,[info] => ,[insert_id] => ,[server_info] => ,[server_version] => ,[sqlstate] => ,[protocol_version] => ,[thread_id] => ,[warning_count] => ),[errors] => Array (),[qual_id] => 4,[employee_id] => 6,[issue_date] => ,[expiration_date] => )) #1 implode(,, ) called at [C:\wamp\www\******\set_qual.php:46] One thing that stands out is, my_error_handler(2, implode() [function.implode]: Invalid arguments passed on line 372. It seems to be so close, but just not right. Any suggestions? -Jason
-
I've changed the code from line 31 and now get no values returned when using implode. Also, I'm getting the following error: #0 my_error_handler(2, implode() [function.implode]: Invalid arguments passed, C:\wamp\www\****\set_qual.php, 46, Array ([GLOBALS] => Array ( *RECURSION*,[_POST] => Array ([qual_id] => 11,[employee_id] => 13,[issue_date] => ,[expiration_date] => ,[Submit] => Submit),[_GET] => Array (),[_COOKIE] => Array ([__utma] => 111872281.652457305.1361574854.1362520724.1362599419.29,[__utmz] => 111872281.1361574854.1.1.utmcsr=(direct)|utmccn=(direct)|utmcmd=(none),[PHPSESSID] => qi20vv92fs1s1v75d4a12tguf0),[_FILES] => Array (),[_ENV] => Array (),[_REQUEST] => Array ([qual_id] => 11,[employee_id] => 13,[issue_date] => ,[expiration_date] => ,[Submit] => Submit),[_SERVER] => Array ([HTTP_HOST] => localhost,[HTTP_USER_AGENT] => Mozilla/5.0 (Windows NT 5.1; rv:19.0) Gecko/20100101 Firefox/19.0,[HTTP_ACCEPT] => text/html,application/xhtml+xml,application/xml;q=0.9,*/*;q=0.8,[HTTP_ACCEPT_LANGUAGE] => en-US,en;q=0.5,[HTTP_ACCEPT_ENCODING] => gzip, deflate,[HTTP_REFERER] => http://localhost/storesund/set_qual.php,[HTTP_COOKIE] => __utma=111872281.652457305.1361574854.1362520724.1362599419.29; __utmz=111872281.1361574854.1.1.utmcsr=(direct)|utmccn=(direct)|utmcmd=(none); PHPSESSID=qi20vv92fs1s1v75d4a12tguf0,[HTTP_CONNECTION] => keep-alive,[CONTENT_TYPE] => application/x-www-form-urlencoded,[CONTENT_LENGTH] => 68,[PATH] => C:\oracle\ora8\jdk\bin\;C:\oracle\ora8\BIN\;C:\Program Files\Oracle\jre\1.1.8\bin\;C:\Program Files\Oracle\jre\1.3.1\bin\;C:\oracle\ora92\bin\;C:\WINDOWS\system32;C:\WINDOWS;C:\WINDOWS\System32\Wbem;C:\Program Files\SAS Institute\Shared Files\Formats\;C:\Program Files\IBM\Personal Communications\;C:\Program Files\IBM\Trace Facility\;C:\Program Files\SASALFA,[SystemRoot] => C:\WINDOWS,[COMSPEC] => C:\WINDOWS\system32\cmd.exe,[PATHEXT] => .COM;.EXE;.BAT;.CMD;.VBS;.VBE;.JS;.JSE;.WSF;.WSH,[WINDIR] => C:\WINDOWS,[SERVER_SIGNATURE] => ,[SERVER_SOFTWARE] => Apache/2.2.21 (Win32) mod_ssl/2.2.21 OpenSSL/0.9.8r PHP/5.3.8,[SERVER_NAME] => localhost,[SERVER_ADDR] => 127.0.0.1,[SERVER_PORT] => 80,[REMOTE_ADDR] => 127.0.0.1,[DOCUMENT_ROOT] => C:/wamp/www/,[SERVER_ADMIN] => admin@localhost,[SCRIPT_FILENAME] => C:/wamp/www/storesund/set_qual.php,[REMOTE_PORT] => 2160,[GATEWAY_INTERFACE] => CGI/1.1,[SERVER_PROTOCOL] => HTTP/1.1,[REQUEST_METHOD] => POST,[QUERY_STRING] => ,[REQUEST_URI] => /storesund/set_qual.php,[SCRIPT_NAME] => /storesund/set_qual.php,[PHP_SELF] => /storesund/set_qual.php,[REQUEST_TIME] => 1363990601),[page_title] => Ny Kvalifikasjon for ansatte!,[thismonth] => March,[_SESSION] => Array ([user_id] => 1,[first_name] => Jason,[user_level] => 0),[dbc] => mysqli Object ([affected_rows] => ,[client_info] => ,[client_version] => ,[connect_errno] => ,[connect_error] => ,[errno] => ,[error] => ,[field_count] => ,[host_info] => ,[info] => ,[insert_id] => ,[server_info] => ,[server_version] => ,[sqlstate] => ,[protocol_version] => ,[thread_id] => ,[warning_count] => ),[errors] => Array (),[qual_id] => 11,[employee_id] => 13,[issue_date] => ,[expiration_date] => ))#1 implode(,, ) called at [C:\wamp\www\****\set_qual.php:42] if (empty($errors)) { print_r($_POST); foreach($_POST['qual_id'] as $row=>$qual_id) { $qual_id=mysqli_real_escape_string($dbc, trim($qual_id)); $employee_id=mysqli_real_escape_string($dbc, trim($_POST['employee_id'][$row])); $issue_date=mysqli_real_escape_string($dbc, trim($_POST['issue_date'][$row])); $expiration_date=mysqli_real_escape_string($dbc, trim($_POST['expiration_date'][$row])); $query_row[] = "('$qual_id','$employee_id','$issue_date', '$expiration_date')"; } $q= "INSERT INTO emp_quals(qual_id, employee_id, issue_date, expiration_date) VALUES " . implode(',',$query_row); $r = @mysqli_query ($dbc, $q);
-
Hello, I've googled this thing to death, but still having problems. I'm trying to insert multiple rows into Mysql with one INSERT INTO query (line 39) using an array and the implode(). The user can select multiple qualifications for one employee. I have the multiple select listing all the qualifications from the quals table on the db. Another select table is listing all the employee names from the employee table. Each employee can have multiple qualifications. I would like the qual_id, employee_id, issue_date, and expiration_date inserted into emp-quals table. The following error keeps appearing: Array ( [qual_id] => 3 [employee_id] => 13 [issue_date] => [expiration_date] => [Submit] => Submit [/] => ) An error occurred in script 'C:\wamp\www\****\set_qual.php' on line 36: Uninitialized string offset: 0 And Column count doesn't match value count at row 1 Query: INSERT INTO emp_quals(qual_id, employee_id, issue_date, expiration_date) VALUES ('("3", 3, 3, 3")', '("1", 1, 1, 1")', '("", , , ")', '("", , , ")', '("S", S, S, S")', '("", , , ")') It seems to be close to working, but I'm not sure how to tweak it. Here is the entire code: <?php #Ny Kvalifikasjon for Ansatte. require('includes/config.inc.php'); $page_title = 'Ny Kvalifikasjon for ansatte!'; include ('includes/header.html'); // Create the submission conditional and initialize the $errors array. if ($_SERVER['REQUEST_METHOD'] == 'POST'){ // Add the MySQL connection include(MYSQL); $errors = array(); $qual_id = mysqli_real_escape_string($dbc, trim($_POST['qual_id'])); $employee_id = mysqli_real_escape_string($dbc, trim($_POST['employee_id'])); $issue_date = mysqli_real_escape_string($dbc, trim($_POST['issue_date'])); $expiration_date = mysqli_real_escape_string($dbc, trim($_POST['expiration_date'])); if (empty($_POST['qual_id'])) { $errors[] = 'Kvalifikasjonen mangler!'; } else { $qual_id = mysqli_real_escape_string($dbc, trim($_POST['qual_id'])); } if (empty($_POST['employee_id'])) { $errors[] = 'Ansatte mangler!'; } else { $employee_id = mysqli_real_escape_string($dbc, trim($_POST['employee_id'])); } if (empty($errors)) { print_r($_POST); //Added print_r tip from margaux at L.U.forum post: http://www.larryullman.com/forums/index.php?/topic/2131-103-edit-user-script-modification/ $values = array(); foreach($_POST as $row ) { $values[] = '("'.mysql_real_escape_string($row['qual_id']).'", '.$row['employee_id'].', '.$row['issue_date'].', '.$row['expiration_date'].'")'; } $q = "INSERT INTO emp_quals(qual_id, employee_id, issue_date, expiration_date)"; $q .= " VALUES ('".implode("', '", $values)."')"; $r = @mysqli_query ($dbc, $q); if ($r) { //See pg 276 echo '<h1>Success!</h1> <p>En ny kvalifikasjon er lagret.</p> <p><a href="qualification.php">Gå til kvalifikasjons oversikt.</a><br /></p>'; } else { echo '<h1>System Error</h1> <p class="error">You could not be registered due to a system error. We apologize for any inconvenience.</p>'; echo '<p>' . mysqli_error($dbc) . '<br /><br />Query: ' . $q . '</p>'; } // End of if ($r) IF. mysqli_close($dbc); include ('includes/footer.html'); exit (); }else { // Report the errors echo '<h1>Error!</h1> <p class="error">The following error(s) occurred:<br />'; foreach ($errors as $msg) { // Print each error messages echo " - $msg<br />\n"; } echo '</p><p>Please try again.</p><p><br /></p>'; } // End of if (empty($errors)) IF. }// End of main submit conditional. //DISPLAY THE FORM ?> <h1>Ny Kvalifikasjon for Ansatte</h1> <form action="set_qual.php" method="post"> <fieldset> <legend>Ny Kvalifikasjon for Ansatte</legend> <label>Qualification:</label><select multiple name="qual_id">'; <?php include(MYSQL); $q = "SELECT qual_id, qual_name FROM quals ORDER BY qual_id ASC"; $r = mysqli_query($dbc, $q); if (mysqli_num_rows($r) > 0) { while ($menu_row = mysqli_fetch_array($r, MYSQLI_NUM)) { echo '<option value="'.$menu_row[0].'">'.$menu_row[0].' - ' . $menu_row[1] . '</option>\n'; } } mysqli_free_result($r); echo '</select><br /><small>Bruk Ctrl knappen for å velge flere.</small><br />'; ?> <label>Employee Name:</label><select name="employee_id">'; <?php $q = "SELECT employee_id, ansattnumber, last_name, first_name FROM employee ORDER BY ansattnumber ASC"; $r = mysqli_query($dbc, $q); if (mysqli_num_rows($r) > 0) { while ($menu_row = mysqli_fetch_array($r, MYSQLI_NUM)) { echo '<option value="'.$menu_row[0].'">' . $menu_row[1] . ' - ' .$menu_row[2] .', '. $menu_row[3]. '</option>\n'; } } mysqli_free_result($r); echo '</select><br />'; ?> <br /> <label>Start dato yyyy-mm-dd:</label><input type="text" name="issue_date" size="15" maxlength="15" value="<?php if(isset($_POST['issue_date'])) echo $_POST['issue_date']; ?>" /><br /><br /> <label>Utløpsdato yyyy-mm-dd:</label><input type="text" name="expiration_date" size="15" maxlength="15" value="<?php if(isset($_POST['expiration_date'])) echo $_POST['expiration_date']; ?>" /><br /><br /> <p><input type="submit" name="Submit" value="Submit" class="button" /></p> <input type="hidden" name= /> </fieldset> </form> <?php include ('includes/footer.html'); ?> Any assistance would be appreciated.
-
Brilliant. It works perfect. At first I kept getting unexpected T_String on the foreach line, until I saw that your loop through the array comment did not have //. Here is the final code in case it may help someone else. Thanks again margaux! <?php # Script 10.3 - edit_user.php // This page is accessed through view_users.php. $page_title = 'Edit a User'; include ('includes/header.html'); echo '<h1>Edit a User</h1>'; //Check for a valid user id if ( (isset($_GET['id'])) && (is_numeric($_GET['id'])) ) { $id = $_GET['id']; } elseif ( (isset($_POST['id'])) && (is_numeric($_POST['id'])) ){ $id = $_POST['id']; } else { echo '<p class="error">This page has been accessed in error.</p>'; include ('includes/footer.html'); exit(); } // End of user id check // Add the MySQL connection include ('mysqli_connect.php'); if ($_SERVER['REQUEST_METHOD'] == 'POST') { //Make use of an array to track all errors. $errors = array(); if (!isset($_POST['car_type'])) { $errors[] = 'You forgot to enter your car_type.'; } else { $car_type = mysqli_real_escape_string($dbc, trim($_POST['car_type'])); } if (empty($_POST['first_name'])) { $errors[] = 'You forgot to enter your first name.'; } else { $fn = mysqli_real_escape_string($dbc, trim($_POST['first_name'])); } if (empty($_POST['last_name'] )) { $errors[] = 'You forgoe to enter your last name.'; } else { $ln = mysqli_real_escape_string($dbc, trim($_POST['last_name'])); } if (empty($_POST['email'] )) { $errors[] = 'You forgot to enter your email.'; } else { $e = mysqli_real_escape_string($dbc, trim($_POST['email'])); } if (empty($errors)) { $q = "SELECT user_id FROM users WHERE email='$e' AND user_id !=$id"; $r = @mysqli_query($dbc, $q); if (mysqli_num_rows($r) == 0) { $q = "UPDATE users SET car_type='$car_type', first_name='$fn', last_name='$ln', email='$e' WHERE user_id=$id LIMIT 1"; $r = @mysqli_query($dbc, $q); if (mysqli_affected_rows($dbc) == 1) { echo '<p>The user has been edited.</p>'; } else { echo '<p class="error">The user could not be edited due to a system error. </p>'; echo '<p>'.mysqli_error($dbc) . '<br />Query: ' . $q .'</p>'; } } else { echo '<p class="error">The email address has already been registered.</p>'; } } else { echo '<p class="error">The following error(s) occurred:<br />'; foreach ($errors as $msg) { echo " - $msg<br />\n"; } echo '</p><p>Please try again.</p>'; } } //End of submit conditional. //Retrieve the information for the user being edited $q = "SELECT car_type, first_name, last_name, email FROM users WHERE user_id=$id"; $r = @mysqli_query ($dbc, $q); if (mysqli_num_rows($r) == 1) { $row = mysqli_fetch_array ($r, MYSQLI_NUM); //DISPLAY THE FORM echo '<form action="edit_user.php" method="post"> <fieldset> <legend>Update User</legend> <label>Car Type:</label> <select name="car_type">'; $car_type = array('VW Van','Chevy Camaro', 'Toyota Corolla'); //create an array for car_types foreach($car_type as $value) {//loop through the array echo ' <option value="'. $value .'"'; //set the option value equal to the array value if ($row[0] == $value) echo 'selected'; //make sticky echo ">$value</option>"; //end option field } echo '</select><br /><br />'; //end select input field echo' <label>First Name:</label><input type="text" name="first_name" size="15" maxlength="15" value="' .$row[1] . '" /><br /> <label>Last Name:</label><input type="text" name="last_name" size="15" maxlength="30" value="' .$row[2] . '" /><br /> <label>Email Address:</label><input type="text" name="email" size="20" maxlength="60" value="' .$row[3] . '" /><br /> <br /> <p><input type="submit" name="submit" class="button" value="Submit" /></p> <input type="hidden" name="id" value="' . $id . '" /> </form>'; }else { echo '<p class="error">This page has been accessed in error.</p>'; } mysqli_close($dbc); include ('includes/footer.html'); ?>
-
Ok. Here is the entire code for view users.php script. <?php #Sript 10.5 - view_users.php //This script retrieves all the records from the users table. // This new version links to edit and delete pages. //Add the title, header, and h1 tag. $page_title = 'View Registered Users'; include ('includes/header.html'); echo '<h1>Registered Users</h1>'; // connect to the database, use require for "mission critical" includes. require_once ('mysqli_connect.php'); //Add number of records to show per page as a variable. $display = 15; //Determine the total number pages. if (isset($_GET['p']) && is_numeric($_GET['p'])) { // if the number of records is already determined. $pages = $_GET['p']; } else { // Count the number of records $q = "SELECT COUNT(user_id) FROM users"; $r = mysqli_query($dbc, $q); //mysqli_query — Performs a query on the database: mysqli $link , string $query $row = mysqli_fetch_array($r, MYSQLI_NUM); $records = $row[0]; //Calculate the number of pages if ($records > $display){ $pages = ceil($records/$display); //ceil — Round fractions up } else { $pages = 1; } } //End of p IF. // Determine where in the database to start returning results. if (isset($_GET['s']) && is_numeric($_GET['s'])) { $start = $_GET['s']; } else{ $start = 0; } //Determine the sort...default is by registration date. $sort = (isset($_GET['sort'])) ? $_GET['sort'] : 'rd'; /*Taken from forum post http://board.phpbuilder.com/showthread.php?10368862-RESOLVED-ASC-DESC-Table. $sort_order = 'asc'; if(isset($_GET['sort_by'])) { if($_GET['sort_by'] == 'asc') { $sort_order = 'desc'; } else { $sort_order = 'asc'; } } End forum code */ //Determine the sorting order. $ln_sort = 'ln'; $car_type_sort = 'car_type'; $fn_sort = 'fn'; $rd_sort = 'rd'; switch ($sort) { case 'ln': $order_by = 'last_name ASC'; $ln_sort = 'lnr'; break; case 'lnr': $order_by = 'last_name DESC'; break; case 'car_type': $order_by = 'car_type ASC'; $car_type_sort = 'rcar_type'; break; case 'rcar_type': $order_by = 'car_type DESC'; break; case 'fn': $order_by = 'first_name ASC'; $fn_sort = 'fnr'; break; case 'fnr': $order_by = 'first_name DESC'; break; case 'rd': $order_by = 'registration_date ASC'; $rd_sort = 'rdr'; break; case 'rdr': $order_by = 'registration_date DESC'; break; default: $order_by = 'registration_date ASC'; $rdr_sort = 'rdr'; $sort = 'rd'; break; } //Define the query: $q = "SELECT car_type, last_name, first_name, DATE_FORMAT(registration_date, '%M %d, %Y') AS dr, user_id FROM users ORDER BY $order_by LIMIT $start, $displsay"; //Run the query $r = @mysqli_query($dbc, $q); //Start the table header echo '<table align="center" cellspacing="0" cellpadding="5" width="75%"> <tr> <td align="left"><b>Edit</b></td> <td align="left"><b>Delete</b></td> <td align="left"><b><a href="view_users.php?sort='. $car_type_sort . '">Car Type</a></b></td> <td align="left"><b><a href="view_users.php?sort='. $ln_sort . '">Last Name</a></b></td> <td align="left"><b><a href="view_users.php?sort='. $fn_sort .'">First Name</a></b></td> <td align="left"><b><a href="view_users.php?sort='. $rd_sort .'">Date Registered</a></b></td> </tr> '; //Fetch and print all the records. $bg = '#eeeeee'; while ($row = mysqli_fetch_array($r, MYSQLI_ASSOC)) { $bg = ($bg=='#eeeeee' ? '#ffffff' : '#eeeeee'); echo '<tr bgcolor="' .$bg . '"> <td align="left"><a href="edit_user.php?id=' . $row['user_id'] . '">Edit</a></td> <td align="left"><a href="delete_user.php?id=' . $row['user_id'] . '">Delete</a></td> <td align="left">' .$row['car_type'] . '</td> <td align="left">' .$row['last_name'] .'</td> <td align="left">' .$row['first_name'] .'</td> <td align="left">' .$row['dr'] .'</td> </tr> '; } //End of WHILE loop. echo '</table>'; mysqli_free_result ($r); mysqli_close($dbc); //Make the links to other pages, if necessary. if ($pages > 1) { echo '<br /><p>'; $current_page = ($start/$display) + 1; // If it's not the first page, make a Previous buttion: if ($current_page != 1) { echo '<a href="view_users.php?s=' . ($start - $display) . '&p=' . $pages . '&sort=' . $sort . '">Previous</a>'; } // Make all the numbered pages. for ($i = 1; $i <= $pages; $i++) { if ($i != $current_page) { echo '<a href="view_users.php?s=' . (($display * ($i - 1))) .'&p=' . $pages . '&sort=' . $sort . '">' . $i . '</a>'; } else { echo $i . ' '; } } // End of FOR loop. //If it's not the last page, make a Next buttion: if ($current_page != $pages) { echo '<a href="view_users.php?s=' . ($start + $display) . '&p=' . $pages . '&sort=' . $sort . '">Next</a>'; } echo '</p>'; // Close the paragraph } // End the page links section include ('includes/footer.html'); ?> And here is the entire coder for the the edit_users.php. Lines 92, 93, and 94 are the problem lines. <?php # Script 10.3 - edit_user.php // This page is accessed through view_users.php. $page_title = 'Edit a User'; include ('includes/header.html'); echo '<h1>Edit a User</h1>'; //Check for a valid user id if ( (isset($_GET['id'])) && (is_numeric($_GET['id'])) ) { $id = $_GET['id']; } elseif ( (isset($_POST['id'])) && (is_numeric($_POST['id'])) ){ $id = $_POST['id']; } else { echo '<p class="error">This page has been accessed in error.</p>'; include ('includes/footer.html'); exit(); } // End of user id check // Add the MySQL connection include ('mysqli_connect.php'); if ($_SERVER['REQUEST_METHOD'] == 'POST') { //Make use of an array to track all errors. $errors = array(); if (empty($_POST['car_type'])) { $errors[] = 'You forgot to enter your car_type.'; } else { $car_type = mysqli_real_escape_string($dbc, trim($_POST['car_type'])); } if (empty($_POST['first_name'])) { $errors[] = 'You forgot to enter your first name.'; } else { $fn = mysqli_real_escape_string($dbc, trim($_POST['first_name'])); } if (empty($_POST['last_name'] )) { $errors[] = 'You forgoe to enter your last name.'; } else { $ln = mysqli_real_escape_string($dbc, trim($_POST['last_name'])); } if (empty($_POST['email'] )) { $errors[] = 'You forgot to enter your email.'; } else { $e = mysqli_real_escape_string($dbc, trim($_POST['email'])); } if (empty($errors)) { $q = "SELECT user_id FROM users WHERE email='$e' AND user_id !=$id"; $r = @mysqli_query($dbc, $q); if (mysqli_num_rows($r) == 0) { $q = "UPDATE users SET car_type='$car_type', first_name='$fn', last_name='$ln', email='$e' WHERE user_id=$id LIMIT 1"; $r = @mysqli_query($dbc, $q); if (mysqli_affected_rows($dbc) == 1) { echo '<p>The user has been edited.</p>'; } else { echo '<p class="error">The user could not be edited due to a system error. </p>'; echo '<p>'.mysqli_error($dbc) . '<br />Query: ' . $q .'</p>'; } } else { echo '<p class="error">The email address has already been registered.</p>'; } } else { echo '<p class="error">The following error(s) occurred:<br />'; foreach ($errors as $msg) { echo " - $msg<br />\n"; } echo '</p><p>Please try again.</p>'; } } //End of submit conditional. //Retrieve the information for the user being edited $q = "SELECT car_type, first_name, last_name, email FROM users WHERE user_id=$id"; $r = @mysqli_query ($dbc, $q); if (mysqli_num_rows($r) == 1) { $row = mysqli_fetch_array ($r, MYSQLI_NUM); //DISPLAY THE FORM echo '<form action="edit_user.php" method="post"> <fieldset> <legend>Update User</legend> <label>Car Type:</label> <select> <option value="' . $row[0] . '">VW Van</option> <option value="' . $row[0] . '">Chevy Camaro</option> <option value="' . $row[0] . '">Toyota Corolla</option> </select><br /> <br /> <label>First Name:</label><input type="text" name="first_name" size="15" maxlength="15" value="' .$row[1] . '" /><br /> <label>Last Name:</label><input type="text" name="last_name" size="15" maxlength="30" value="' .$row[2] . '" /><br /> <label>Email Address:</label><input type="text" name="email" size="20" maxlength="60" value="' .$row[3] . '" /><br /> <br /> <p><input type="submit" name="submit" class="button" value="Submit" /></p> <input type="hidden" name="id" value="' . $id . '" /> </form>'; }else { echo '<p class="error">This page has been accessed in error.</p>'; } mysqli_close($dbc); include ('includes/footer.html'); ?>
-
Margaux and HS thanks for the replies: Yes, this was a typo. As you said, missing a double quote. <option value="'. $row[0] . '>VW Van</option> What I'm trying to achieve is the same as the 10.3 edit_user script except that in addition to first name, last name and email, I would like to UPDATE the user's car type using a select, dropdown. The two screenshot links will hopefully make sense. I would like to change Ringo Starr's car type from Chevy Camaro to VW Van. Clicking on the Edit user here: https://docs.google.com/file/d/0B5uX0wJkztzXMjJVTWRoR3liNW8/edit?usp=sharing Should display as shown here: https://docs.google.com/file/d/0B5uX0wJkztzXOUI5dmhiRTh3cjA/edit?usp=sharing With the exception of a dropdown menu for car type, like this: https://docs.google.com/file/d/0B5uX0wJkztzXUVE3d1hiLXlFZ1U/edit?usp=sharing Here is the code from the book, script 10.3, with the html. echo '<form action="edit_user.php" method="post"> <fieldset> <legend>Update User</legend> <label>Car Type:</label><input type="text" name="car_type" size="15" maxlength="15" value="' .$row[0] . '" /><br /> <label>First Name:</label><input type="text" name="first_name" size="15" maxlength="15" value="' .$row[1] . '" /><br /> <label>Last Name:</label><input type="text" name="last_name" size="15" maxlength="30" value="' .$row[2] . '" /><br /> <label>Email Address:</label><input type="text" name="email" size="20" maxlength="60" value="' .$row[3] . '" /><br /> <br /> <p><input type="submit" name="submit" class="button" value="Submit" /></p> <input type="hidden" name="id" value="' . $id . '" /> </form>'; I have tried to change line 4 to this: (below code) <label>Car Type:</label> <select> <option value="' . $row[0] . '">VW Van</option> <option value="' . $row[0] . '">Chevy Camaro</option> <option value="' . $row[0] . '">Toyota Corolla</option> </select> But when submitting, only get this: https://docs.google.com/file/d/0B5uX0wJkztzXaFJXeEhzY1lWZmM/edit?usp=sharing btw There is only one table (users) in the database, with the following columns: user_id, car_type, first_name, last_name, email, pass, and registration_date. :
-
Hi, I'm trying to modify the 10.3 edit user script on page 310, specifically, the create form section. I would like to use a <select> tag instead of a text input for one of the form fields. It seems very basic, however, I'm struggling to get the code to work for the select option values. Maybe it's a matter of single or double quotes, but I need some help to actually figure this out. The form is inside an echo. I've tried: echo ' <form action="edit-users.php" method="post"> <fieldset> <legend>Update User Information</legend> <label>Your First Car:</label> <select name="car_type"> <option value="'. $row[0] .'>VW Van</option> <option value="'. $row[0] .'>Toyota Corolla</option> <option value="'. $row[0] .'>Chevy Camaro</option> </select> <br /> <br /> <label>First Name:</label><input type="text" name="first_name" size="20" maxlength="60" value="' .$row[1] . '" /><br /> <label>Last Name:</label><input type="text" name="last_name" size="20" maxlength="60" value="' .$row[2] . '" /><br /> <label>Email:</label><input type="text" name="email" size="20" maxlength="60" value="' .$row[3] . '" /><br /><br /> <p><input type="submit" class="button" name="submit" value="Submit" /></p> <input type="hidden" name="id" value="' . $id . '" /> </fieldset> </form>'; And this: echo ' <form action="edit-users.php" method="post"> <fieldset> <legend>Update User Information</legend> <label>Your First Car:</label> <select name="car_type"> <option value="VW Van"' if($row['0'] =='VW Van') echo "selected='selected'"'>VW Van</option> <option value="Toyota Corolla"'if ($row['0'] =='Toyota Corolla') echo "selected='selected'"'>Toyota Corolla</option> <option value="Chevy Camaro"'if ($row['0'] =='Chevy Camaro') echo "selected='selected'"'>Chevy Camaro</option </select> <br /> <br /> <label>First Name:</label><input type="text" name="first_name" size="20" maxlength="60" value="' .$row[1] . '" /><br /> <label>Last Name:</label><input type="text" name="last_name" size="20" maxlength="60" value="' .$row[2] . '" /><br /> <label>Email:</label><input type="text" name="email" size="20" maxlength="60" value="' .$row[3] . '" /><br /><br /> <p><input type="submit" class="button" name="submit" value="Submit" /></p> <input type="hidden" name="id" value="' . $id . '" /> </fieldset> </form>'; Plus I have tried numorous other combinations with no luck. Any help will be appreciated.
-
Thanks for the kick in the right direction. That worked! Here is the code, in case it may help someone else: //Define the query: NOTE: acr is the table name. One, five, and freetext are form input names. $q = "SELECT * FROM acr WHERE one LIKE \"%$one%\" AND five LIKE \"$five%\" AND (six LIKE \"%$freetext%\" OR two LIKE \"%$freetext%\" OR four LIKE \"%$freetext%\" OR seven LIKE \"%$freetext%\" OR nine LIKE \"%$freetext%\" OR eight LIKE \"%$freetext%\") ORDER BY $order_by LIMIT $start, $display"; //Run the query $r = @mysqli_query($dbc, $q) or die("Error: ".mysqli_error($dbc)); //Start the table header echo '<table align="center" cellspacing="15" cellpadding="2" width="100%"> <tr>"There were ' . $records . ' hits for your search '.$q . '. " <tr> <td align="left"><b>Details</b></td> <td align="left"><b><a href="data-search.php?one='.$one.' &five='.$five.' &freetext='.$freetext.'&sort='. $one_sort. '">One</a></b></td> <td align="left"><b><a href="data-search.php?one='.$one.' &five='.$five.' &freetext='.$freetext.'&sort='. $two_sort .'">Two</a></b></td> <td align="left"><b><a href="data-search.php?one='.$one.' &five='.$five.' &freetext='.$freetext.'&sort='. $three_sort.'">Three</a></b></td> <td align="left"><b><a href="data-search.php?one='.$one.' &five='.$five.' &freetext='.$freetext.'&sort='. $four_sort .'">Four</a></b></td> <td align="left"><b><a href="data-search.php?one='.$one.' &five='.$five.' &freetext='.$freetext.'&sort='. $five_sort .'">Five</a></b></td> <td align="left"><b><a href="data-search.php?one='.$one.' &five='.$five.' &freetext='.$freetext.'&sort='. $six_sort .'">Six</a></b></td> <td align="left"><b><a href="data-search.php?one='.$one.' &five='.$five.' &freetext='.$freetext.'&sort='. $seven_sort .'">Seven</a></b></td> <td align="left"><b><a href="data-search.php?one='.$one.' &five='.$five.' &freetext='.$freetext.'&sort='. $eight_sort .'">Eight</a></b></td> </tr>'; //Fetch and print all the records. $bg = '#eeeeee'; while ($row = mysqli_fetch_array($r, MYSQLI_ASSOC)){ $bg = ($bg=='#eeeeee' ? '#ffffff' : '#eeeeee'); echo ' <tr bgcolor="' .$bg . '"> <td valign ="top" align="left"><a href="edit.php?id=' . $row['acr_id'] . '">Details</a></td> <td valign ="top" align="left">'.$row['one'] .'</td> <td valign ="top" align="left">'.$row['two'] .'</td> <td valign ="top" align="left">'.$row['three'] .'</td> <td valign ="top" align="left">'.$row['four'] .'</td> <td valign ="top" align="left">'.$row['five'] .'</td> <td valign ="top" align="left">'.$row['six'] .'</td> <td valign ="top" align="left">'.$row['seven'] .'</td> <td valign ="top" align="left">'.$row['eight'] .'</td> </tr> '; } //End of WHILE loop. echo '</table>';
-
Hi, I've modified script 10.5 by adding a search form at the top. The search correctly returns a paginated table of results with page numbers and a next link. However, there are some problems that is beyond my knowledge of php. The first is that I don't know how to prevent the undefined index error caused by the blank form fields when the next link or a page number link is clicked. In other words, trying to view the paged results creates an undefined index error. The second thing is that clicking the sort link returns all the database records, not just the search results. The code is posted. Any help would be appreciated. <?php $page_title = 'Search'; include ('includes/header.html'); require("mysqli_connect_skn.php"); ?> <form action="<?php echo $_SERVER['PHP_SELF']; ?>" method="GET"> <h1>Search the Database</h1> <h3>Return (hopefully) Paginated search results with sortable columns</h3> <p>Form Field One: <input name="one" type="text" size="6" maxlength="6" value="<?php if(isset($_POST['one'])) echo $_POST['one']; ?>" /> </p> <p>Form Field Two: <input name="five" type="text" size="5" maxlength="5" value="<?php if(isset($_POST['five'])) echo $_POST['five']; ?>" /> </p> <p>Form Field Three: <input name="freetext" type="text" size="40" maxlength="40" value="<?php if(isset($_POST['freetext'])) echo $_POST['freetext']; ?>" /> </p> <p> <input type="submit" value="Search"> </p> </form> <?php #Sript 10.5 - view_users.php //Add number of records to show per page as a variable. $one = mysqli_real_escape_string($dbc, trim($_GET['one'])); $five = mysqli_real_escape_string($dbc, trim($_GET['five'])); $freetext = mysqli_real_escape_string($dbc, trim($_GET['freetext'])); $display = 10; //Determine the total number pages. if (isset($_GET['p']) && is_numeric($_GET['p'])) { // if the number of records is already determined. $pages = $_GET['p']; } else { // Count the number of records $q = "SELECT COUNT(acr_id) FROM acr WHERE one LIKE \"%$one%\" AND five LIKE \"$five%\" AND (six LIKE \"%$freetext%\" OR two LIKE \"%$freetext%\" OR four LIKE \"%$freetext%\" OR seven LIKE \"%$freetext%\" OR nine LIKE \"%$freetext%\" OR eight LIKE \"%$freetext%\")"; $r = mysqli_query($dbc, $q); $row = mysqli_fetch_array($r, MYSQLI_NUM); $records = $row[0]; //Calculate the number of pages if ($records > $display){ $pages = ceil($records/$display); //ceil — Round fractions up } else { $pages = 1; } } //End of p IF. // Determine where to start returning results. if (isset($_GET['s']) && is_numeric($_GET['s'])) { $start = $_GET['s']; } else{ $start = 0; } //Determine the default sort. $sort = (isset($_GET['sort'])) ? $_GET['sort'] : 'three'; //Determine the sorting order. $one_sort = 'one'; $seq_sort = 'two'; $date_sort = 'three'; $four_sort = 'four'; $five_sort = 'five'; $comp_sort = 'six'; $seven_sort = 'seven'; $eight_sort = 'eight'; switch ($sort) { case 'one': $order_by = 'one ASC'; $one_sort = 'rone'; break; case 'rone': $order_by = 'one DESC'; break; case 'two': $order_by = 'two ASC'; $seq_sort = 'rtwo'; break; case 'rtwo': $order_by = 'two DESC'; break; case 'three': $order_by = 'three ASC'; $date_sort = 'rthree'; break; case 'rthree': $order_by = 'three DESC'; break; case 'four': $order_by = 'four ASC'; $four_sort = 'rfour'; break; case 'rfour': $order_by = 'four DESC'; break; case 'five': $order_by = 'five ASC'; $five_sort = 'rfive'; break; case 'rfive': $order_by = 'five DESC'; break; case 'six': $order_by = 'six ASC'; $comp_sort = 'rsix'; break; case 'rsix': $order_by = 'six DESC'; break; case 'seven': $order_by = 'seven ASC'; $seven_sort = 'rseven'; break; case 'rseven': $order_by = 'seven DESC'; break; case 'eight': $order_by = 'eight ASC'; $eight_sort = 'reight'; break; case 'reight': $order_by = 'eight DESC'; break; } //Define the query: $q = "SELECT * FROM acr WHERE one LIKE \"%$one%\" AND five LIKE \"$five%\" AND (six LIKE \"%$freetext%\" OR two LIKE \"%$freetext%\" OR four LIKE \"%$freetext%\" OR seven LIKE \"%$freetext%\" OR nine LIKE \"%$freetext%\" OR eight LIKE \"%$freetext%\") ORDER BY $order_by LIMIT $start, $display"; //Run the query $r = @mysqli_query($dbc, $q) or die("Error: ".mysqli_error($dbc)); //Start the table header echo '<table align="center" cellspacing="15" cellpadding="2" width="100%"> <tr>"There were ' . $records . ' hits for your search '.$q . '. " <tr> <td align="left"><b>Details</b></td> <td align="left"><b><a href="data-search.php?sort='. $one_sort. '">One</a></b></td> <td align="left"><b><a href="data-search.php?sort='. $two_sort .'">Two</a></b></td> <td align="left"><b><a href="data-search.php?sort='. $three_sort.'">Three</a></b></td> <td align="left"><b><a href="data-search.php?sort='. $four_sort .'">Four</a></b></td> <td align="left"><b><a href="data-search.php?sort='. $five_sort .'">Five</a></b></td> <td align="left"><b><a href="data-search.php?sort='. $six_sort .'">Six</a></b></td> <td align="left"><b><a href="data-search.php?sort='. $seven_sort .'">Seven</a></b></td> <td align="left"><b><a href="data-search.php?sort='. $eight_sort .'">Eight</a></b></td> </tr> '; //Fetch and print all the records. $bg = '#eeeeee'; while ($row = mysqli_fetch_array($r, MYSQLI_ASSOC)){ $bg = ($bg=='#eeeeee' ? '#ffffff' : '#eeeeee'); echo ' <tr bgcolor="' .$bg . '"> <td valign ="top" align="left"><a href="edit.php?id=' . $row['acr_id'] . '">Details</a></td> <td valign ="top" align="left">'.$row['one'] .'</td> <td valign ="top" align="left">'.$row['two'] .'</td> <td valign ="top" align="left">'.$row['three'] .'</td> <td valign ="top" align="left">'.$row['four'] .'</td> <td valign ="top" align="left">'.$row['five'] .'</td> <td valign ="top" align="left">'.$row['six'] .'</td> <td valign ="top" align="left">'.$row['seven'] .'</td> <td valign ="top" align="left">'.$row['eight'] .'</td> </tr> '; } //End of WHILE loop. echo '</table>'; mysqli_free_result ($r); mysqli_close($dbc); //Make the links to other pages, if necessary. if ($pages > 1) { echo '<br /><p>'; $current_page = ($start/$display) + 1; // If it's not the first page, make a Previous buttion: if ($current_page != 1) { echo '<a href="'.$_SERVER['PHP_SELF'].'?s=' . ($start - $display) . '&p=' . $pages . '&sort=' . $sort . '">Previous</a>'; } // Make all the numbered pages. for ($i = 1; $i <= $pages; $i++) { if ($i != $current_page) { echo '<a href="'.$_SERVER['PHP_SELF'].'?s=' . (($display * ($i - 1))) .'&p=' . $pages . '&sort=' . $sort . '">' . $i . '</a>'; } else { echo $i . ' '; } } // End of FOR loop. //If it's not the last page, make a Next buttion: if ($current_page != $pages) { echo '<a href="'.$_SERVER['PHP_SELF'].'?s=' . ($start + $display) . '&p=' . $pages . '&sort=' . $sort . '">Next</a>'; } echo '</p>'; // Close the paragraph } // End the page links section include ('includes/footer.html'); ?>