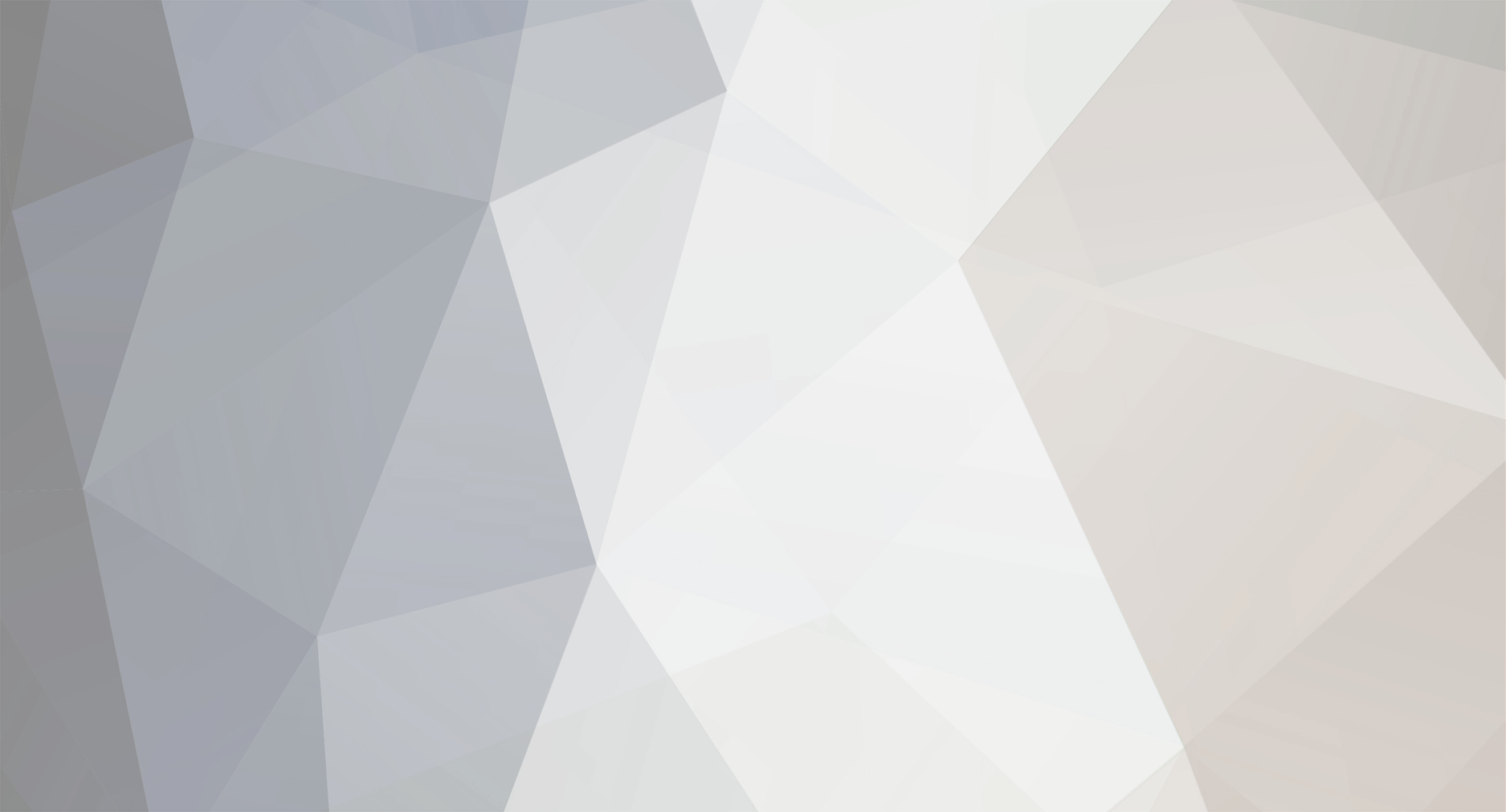
Roy
-
Posts
6 -
Joined
-
Last visited
Posts posted by Roy
-
-
Let's see if I even got this right...
From what I understand, the following creates a named function and assigns it to a variable:
var someVar = function functionName() { //function body };
And this creates an anonymous function assigned to a variable:
var someFunction = function() { //function body };
What I don't understand is what the functional difference is between the two, so could someone please explain this to me?
-
Thank you for clarifying. I guess I was somewhat confused by the wording in the book
-
In chapter 6, on page 230, it is said that when you, intentionally, want to create a sparsely populated arrays it is best to be explicit about it in the following way:
var myList = [1, undefined, 3, undefined, 5];
However, this leads to a problem when using an if in condition in a for loop, like so:
for (var i = 0, count = myList.length; i < count; i++) { if (i in myList) { console.log(myList[i]); } }
Under normal circumstances, in a sparsely populated array, this would lead to only the defined elements (1, 3 and 5) outputting to the console. But when explicitely using undefined to create these "holes", it leads to the entire list, including undefined elements being outputted.
Full example:
var myList = [1, undefined, 3, undefined, 5]; for (var i = 0, count = myList.length; i < count; i++) { if (i in myList) { console.log(myList[i]); } } 1 undefined 3 undefined 5
This, however, does work:
var myList = [1, , 3, , 5]; for (var i = 0, count = myList.length; i < count; i++) { if (i in myList) { console.log(myList[i]); } } 1 3 5
-
-
I've been racking my brain over how to achieve this, but I'm stumped.
Here's the code I use in the relevant contact.js:
function process() { 'use strict'; //set initial check for validation to true because there are no errors yet var okay = true; //reference to email and comments elements var email = document.getElementById('email'); var comments = document.getElementById('comments'); //validate email if (!email || !email.value || (email.value.length < 6) || (email.value.indexOf('@') == -1)) { okay = false; alert('Please enter a valid email address'); }//end if //validate comments if (!comments || !comments.value || (comments.value.indexOf('<') != -1)) { okay = false; alert('Please enter your comments, without any HTML!'); }//end if return okay; }//end process() function //event listener function init() { 'use strict'; //call process upon submission of form document.getElementById('theForm').onsubmit = process; }//end init function() window.onload = init;
Question Regarding Named And Anonymous Funcitons Assigned To Variables
in Modern Javascript: Develop and Design
Posted
Thank you both for the information. It is greatly appreciated!