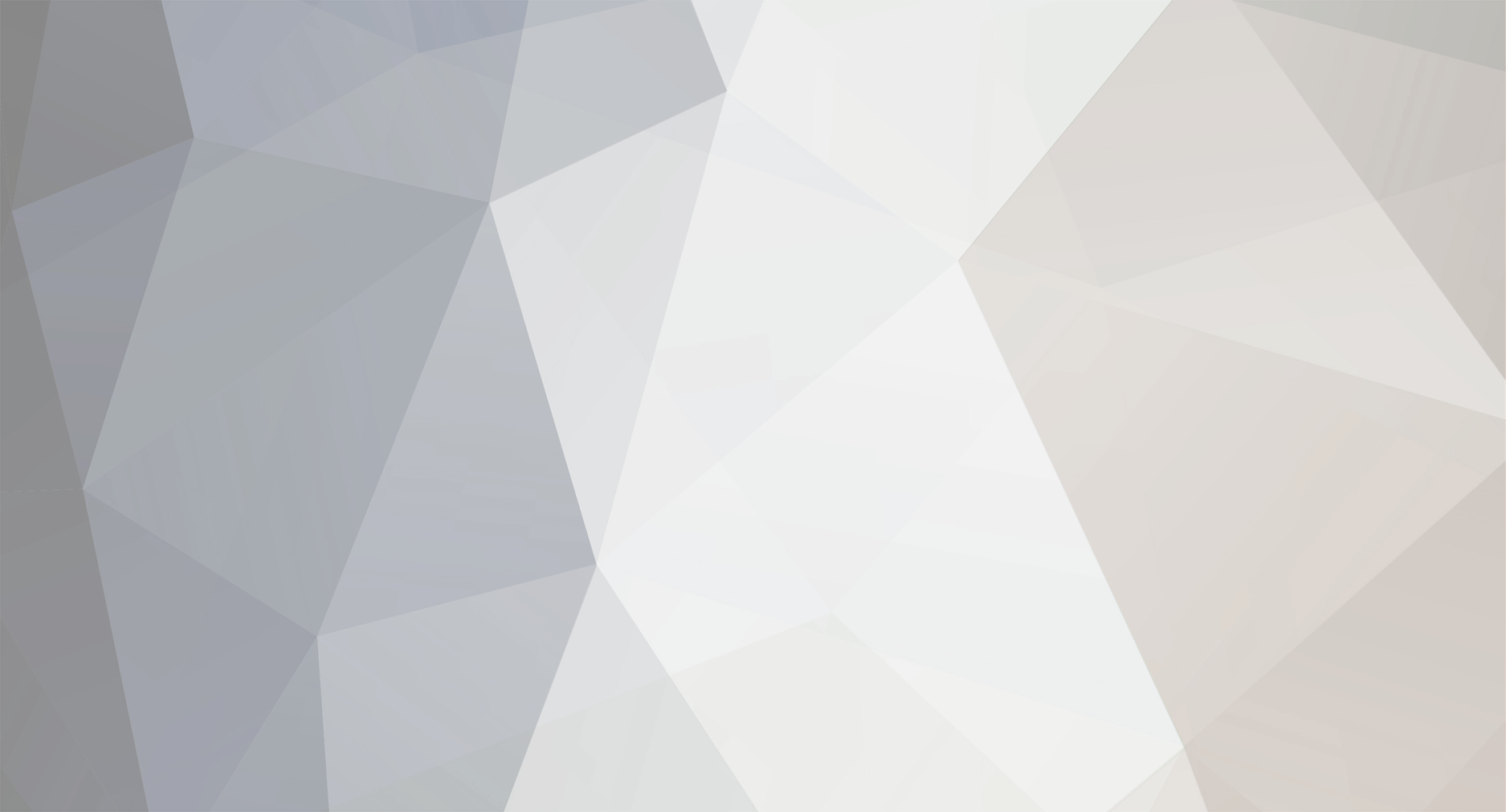
john.woolley
-
Posts
12 -
Joined
-
Last visited
Posts posted by john.woolley
-
-
Update: I fixed it with this line of code (thanks for your help again HartleySAN.
require ('../includes/mysqli_connect_dating.php'); // connect to the DB $errors = array(); // Initialize an error array. $ua = $_POST['useralias']; $q = "SELECT useralias from USERS where useralias='$ua'"; $r = mysqli_query($dbc, $q); $rowcount = mysqli_num_rows($r); if ($rowcount == 1) { $errors[] = 'That screen name has already been taken'; } $em = $_POST['email']; $q = "SELECT email from USERS where email='$em'"; $r = mysqli_query($dbc, $q); $rowcount = mysqli_num_rows($r); if ($rowcount == 1) { $errors[] = 'That email address is already in use'; }
I basically took the line that if I didn't need to nest the IF statement then I shouldn't, so the script checks to see if the password and screen name are unique. If not then it puts it into errors.
How does the code look? Is there a more concise way of writing it?
-
<? // Check for form submission: if ($_SERVER['REQUEST_METHOD'] == 'POST') { require ('../includes/mysqli_connect_dating.php'); // connect to the DB $errors = array(); // Initialize an error array. /* Check for a useralias name: if (empty($_POST['useralias'])) { $errors[] = 'You forgot to enter your Screen Name.'; } elseif { $ua = $_POST['useralias']; $q = "SELECT useralias from USERS where email='$ua'"; $r = mysqli_query($dbc, $q); $row = mysqli_fetch_array($r, MYSQLI_ASSOC); if ($row) {$errors[] = 'That user alias has already been taken.';} } else { $ua = mysqli_real_escape_string($dbc, trim($_POST['useralias'])); } */ if (!empty($_POST['useralias'])) { $ua = $_POST['useralias']; $q = "SELECT useralias from USERS where email='$ua'"; $r = mysqli_query($dbc, $q); $rowcount=mysqli_num_rows($r); if ($rowcount > 1) { $errors[] = 'That user alias has already been taken.'; } else { $ua = mysqli_real_escape_string($dbc, trim($_POST['useralias'])); } } else { $errors[] = 'You forgot to enter your Screen Name.'; } // Check for a first name: if (empty($_POST['firstname'])) { $errors[] = 'You forgot to enter your first name.'; } else { $fn = mysqli_real_escape_string($dbc, trim($_POST['firstname'])); } // Check for a last name: if (empty($_POST['lastname'])) { $errors[] = 'You forgot to enter your last name.'; } else { $ln = mysqli_real_escape_string($dbc,trim($_POST['lastname'])); } // Check for an email address: if (empty($_POST['email'])) { $errors[] = 'You forgot to enter your email address.'; } else { $e = mysqli_real_escape_string($dbc, trim($_POST['email'])); } // Check for an GENDER: if (empty($_POST['gender'])) { $errors[] = 'You forgot to enter your Gender.'; } else { $g = mysqli_real_escape_string($dbc, trim($_POST['gender'])); } //TRY IT THIS WASY if ($_POST['udobdate'] == '' && $_POST['udobmonth'] == '' && $_POST['udobyear'] == '') { $errors[] = 'You forgot to enter your Date of Birth.'; } else { $udob = $_POST['udobyear'] . '/' . $_POST['udobmonth'] . '/' . $_POST['udobdate']; $dob = mysqli_real_escape_string($dbc, $udob); } // Check for a password and match against the confirmed password: if (!empty($_POST['pass1'])) { if ($_POST['pass1'] != $_POST['pass2']) { $errors[] = 'Your password did not match the confirmed password.'; } else { $p = mysqli_real_escape_string($dbc, trim($_POST['pass1'])); //USE THE ESCAPE PIECE TO SECURE THE USER INPUT } } else { $errors[] = 'You forgot to enter your password.'; } if (empty($errors)) { // If everything's OK. // Register the user in the database... require ('../includes/mysqli_connect_dating.php'); // Connect to the db. // Make the query: $q = "INSERT INTO users (useralias, firstname, lastname, email, gender, dob, password, registration_date) VALUES ('$ua', '$fn', '$ln', '$e', '$g', '$dob', SHA1('$p'), NOW() )"; $r = @mysqli_query ($dbc, $q); // Run the query. if ($r) { // If it ran OK. // Declare the session variable ready for register 2 session and go to the next page ? - Print a message: $_SESSION['user'] = $_POST['email']; echo ' <h1>Thank you! ' . $_SESSION['user'] . '</h1> <p>You are now registered. In Chapter 12 you will actually be able to log in!</p><p><br /></p>'; } else { // If it did not run OK. // Public message: echo '<h1>System Error</h1> <p class="error">You could not be registered due to a system error. We apologize for any inconvenience.</p>'; // Debugging message: echo '<p>' . mysqli_error($dbc) . '<br /><br />Query: ' . $q . '</p>'; } // End of if ($r) IF. mysqli_close($dbc); // Close the database connection. // Include the footer and quit the script: exit(); } else { // Report the errors. echo '<h1>Error!</h1> <p class="error">The following error(s) occurred:<br />'; foreach ($errors as $msg) { // Print each error. echo " - $msg<br />\n"; } echo '</p><p>Please try again.</p><p><br /></p>'; } // End of if (empty($errors)) IF. } // End of the main Submit conditional. ?> <h1>Register</h1> <form action="register.php" method="post"> <fieldset> <legend>Enter Your Details</legend> <p>Screen Name: <input type="text" name="useralias" size="15" maxlength="20" value="<?php if (isset($_POST['useralias'])) echo $_POST['useralias']; ?>" /></p> <p>First Name: <input type="text" name="firstname" size="15" maxlength="20" value="<?php if (isset($_POST['firstname'])) echo $_POST['firstname']; ?>" /></p> <p>Last Name: <input type="text" name="lastname" size="15" maxlength="40" value="<?php if (isset($_POST['lastname'])) echo $_POST['lastname']; ?>" /></p> <p>Email Address: <input type="text" name="email" size="20" maxlength="60" value="<?php if (isset($_POST['email'])) echo $_POST['email']; ?>" /> </p> <p>Gender: (<input type="radio" name="gender" value="<?php if (isset($_POST['gender'])) echo $_POST['gender']; ?>Male"> Male) (<input type="radio" name="gender" value="<?php if (isset($_POST['gender'])) echo $_POST['gender']; ?>Female"> Female)</p> <!--<p>Date of Birth: (DD/MM/YY)<input type="date" name="udob" value="<?php if (isset($_POST['dob'])) echo $_POST['udob']; ?>"/></p> --> <p>Date of Birth: (Date) <select name="udobdate"><?php for ($day = 1; $day <=31; $day++) { echo "<option value=\"$day\">$day</option>\n"; } echo '</select>'; ?> (Month) <select name="udobmonth"><option value="01">January</option><option value="02">February</option><option value="03">March</option><option value="04">April</option><option value="05">May</option><option value="06">June</option><option value="07">July</option><option value="08">August</option><option value="09">September</option><option value="10">October</option><option value="11">November</option><option value="12">December</option></select> Year <select name="udobyear"><?php date_default_timezone_set('GMT'); for ($year = DATE(Y); $year >= 1900; $year--) { echo "<option value=\"$year\">$year</option>\n"; } echo '</select>'; ?> <p>Password: <input type="password" name="pass1" size="10" maxlength="20" value="<?php if (isset($_POST['pass1'])) echo $_POST['pass1']; ?>" /></p> <p>Confirm Password: <input type="password" name="pass2" size="10" maxlength="20" value="<?php if (isset($_POST['pass2'])) echo $_POST['pass2']; ?>" /></p> <p><input type="reset" name"clear" action"clear" value="clear"/> <input type="submit" name="submit" value="Register" /></p> </fieldset> </form> </body> </html>
Here's the whole script for reference. I'm 99.99999% sure I'm doing something wrong with the IF..
-
if (!empty($_POST['useralias'])) { $ua = $_POST['useralias']; $q = "SELECT useralias from USERS where email='$ua'"; $r = mysqli_query($dbc, $q); $rowcount=mysqli_num_rows($r); if ($rowcount > 1) { $errors[] = 'That user alias has already been taken.'; } else { $ua = mysqli_real_escape_string($dbc, trim($_POST['useralias'])); } } else { $errors[] = 'You forgot to enter your Screen Name.'; }
Like this?
I think the problem is more that the IF function isn't catching it to create the error before the SQL Query tries to write it and then I get the SQL to the screen rather than the output of the $errors[ ] array...
-
Hi guys
So here's what I'm trying to do.
1. Validate a user alias isn't already in use in the database - if it is - add it to errors
2. Validate if it's empty - if it is add it to errors
3. If not chuck it write into the database.
if (!empty($_POST['useralias'])) { $ua = $_POST['useralias']; $q = "SELECT useralias from USERS where email='$ua'"; $r = mysqli_query($dbc, $q); $row = mysqli_fetch_array($r, MYSQLI_ASSOC); if ($row) { $errors[] = 'That user alias has already been taken.'; } else { $ua = mysqli_real_escape_string($dbc, trim($_POST['useralias'])); } } else { $errors[] = 'You forgot to enter your Screen Name.'; }
Does this look about right?
Because when I run the script the error doesn't show if it's a duplicate - but I do get the SQL error telling me it needs to be unique.
-
I missed the obvious Hartley, thanks.
I've now put the session start right at the top it's the opening line before the <html> tag.
I'm guessing now that I can declare session variables throughout the script and then terminate that particular session when the registration is complete right?
-
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title></title>
<!--[if lt IE 9]>
<script src="http://html5shim.googlecode.com/svn/trunk/html5.js"></script>
<![endif]-->
</head>
<body>
<?
// Check for form submission:
session_start();
if ($_SERVER['REQUEST_METHOD'] == 'POST') {
require ('../includes/mysqli_connect_dating.php'); // connect to the DB
$errors = array(); // Initialize an error array.
// Check for a useralias name:
if (empty($_POST['useralias'])) {
$errors[] = 'You forgot to enter your Screen Name.';
} else {
$ua = mysqli_real_escape_string($dbc, trim($_POST['useralias']));
}
// Check for a first name:
if (empty($_POST['firstname'])) {
$errors[] = 'You forgot to enter your first name.';
} else {
$fn = mysqli_real_escape_string($dbc, trim($_POST['firstname']));
}
// Check for a last name:
if (empty($_POST['lastname'])) {
$errors[] = 'You forgot to enter your last name.';
} else {
$ln = mysqli_real_escape_string($dbc,trim($_POST['lastname']));
}
// Check for an email address:
if (empty($_POST['email'])) {
$errors[] = 'You forgot to enter your email address.';
} else {
$e = mysqli_real_escape_string($dbc, trim($_POST['email']));
}
// Check for an GENDER:
if (empty($_POST['gender'])) {
$errors[] = 'You forgot to enter your Gender.';
} else {
$g = mysqli_real_escape_string($dbc, trim($_POST['gender']));
}
//TRY IT THIS WASY
if ($_POST['udobdate'] == '' && $_POST['udobmonth'] == '' && $_POST['udobyear'] == '') {
$errors[] = 'You forgot to enter your Date of Birth.';
} else {
$udob = $_POST['udobyear'] . '/' . $_POST['udobmonth'] . '/' . $_POST['udobdate'];
$dob = mysqli_real_escape_string($dbc, $udob);
}
// Check for a password and match against the confirmed password:
if (!empty($_POST['pass1'])) {
if ($_POST['pass1'] != $_POST['pass2']) {
$errors[] = 'Your password did not match the confirmed password.';
} else {
$p = mysqli_real_escape_string($dbc, trim($_POST['pass1'])); //USE THE ESCAPE PIECE TO SECURE THE USER INPUT
}
} else {
$errors[] = 'You forgot to enter your password.';
}
if (empty($errors)) { // If everything's OK.
// Register the user in the database...
require ('../includes/mysqli_connect_dating.php'); // Connect to the db.
// Make the query:
$_SESSION['useremail'] = $_POST['email'];
$q = "INSERT INTO users (useralias, firstname, lastname, email, gender, dob, password, registration_date) VALUES ('$ua', '$fn', '$ln', '$e', '$g', '$dob', SHA1('$p'), NOW() )";
$r = @mysqli_query ($dbc, $q); // Run the query.
if ($r) { // If it ran OK.
// Start a session and go to the next page ? - Print a message:
echo '<h1>Thank you! ' . $_SESSION['user_email'] . '</h1>
//<p>You are now registered. In Chapter 12 you will actually be able to log in!</p><p><br /></p>';
} else { // If it did not run OK.
// Public message:
echo '<h1>System Error</h1>
<p class="error">You could not be registered due to a system error. We apologize for any inconvenience.</p>';
// Debugging message:
echo '<p>' . mysqli_error($dbc) . '<br /><br />Query: ' . $q . '</p>';
} // End of if ($r) IF.
mysqli_close($dbc); // Close the database connection.
// Include the footer and quit the script:
exit();
} else { // Report the errors.
echo '<h1>Error!</h1>
<p class="error">The following error(s) occurred:<br />';
foreach ($errors as $msg) { // Print each error.
echo " - $msg<br />\n";
}
echo '</p><p>Please try again.</p><p><br /></p>';
} // End of if (empty($errors)) IF.
} // End of the main Submit conditional.
?>
<h1>Register</h1>
<form action="register.php" method="post">
<fieldset>
<legend>Enter Your Details</legend>
<p>Screen Name: <input type="text" name="useralias" size="15" maxlength="20" value="<?php if (isset($_POST['useralias'])) echo $_POST['useralias']; ?>" /></p>
<p>First Name: <input type="text" name="firstname" size="15" maxlength="20" value="<?php if (isset($_POST['firstname'])) echo $_POST['firstname']; ?>" /></p>
<p>Last Name: <input type="text" name="lastname" size="15" maxlength="40" value="<?php if (isset($_POST['lastname'])) echo $_POST['lastname']; ?>" /></p>
<p>Email Address: <input type="text" name="email" size="20" maxlength="60" value="<?php if (isset($_POST['email'])) echo $_POST['email']; ?>" /> </p>
<p>Gender: (<input type="radio" name="gender" value="<?php if (isset($_POST['gender'])) echo $_POST['gender']; ?>Male"> Male) (<input type="radio" name="gender" value="<?php if (isset($_POST['gender'])) echo $_POST['gender']; ?>Female"> Female)</p>
<!--<p>Date of Birth: (DD/MM/YY)<input type="date" name="udob" value="<?php if (isset($_POST['dob'])) echo $_POST['udob']; ?>"/></p> -->
<p>Date of Birth: (Date) <select name="udobdate"><?php for ($day = 1; $day <=31; $day++) { echo "<option value=\"$day\">$day</option>\n"; } echo '</select>'; ?> (Month) <select name="udobmonth"><option value="01">January</option><option value="02">February</option><option value="03">March</option><option value="04">April</option><option value="05">May</option><option value="06">June</option><option value="07">July</option><option value="08">August</option><option value="09">September</option><option value="10">October</option><option value="11">November</option><option value="12">December</option></select> Year <select name="udobyear"><?php date_default_timezone_set('GMT'); for ($year = DATE(Y); $year >= 1900; $year--) { echo "<option value=\"$year\">$year</option>\n"; } echo '</select>'; ?>
<p>Password: <input type="password" name="pass1" size="10" maxlength="20" value="<?php if (isset($_POST['pass1'])) echo $_POST['pass1']; ?>" /></p>
<p>Confirm Password: <input type="password" name="pass2" size="10" maxlength="20" value="<?php if (isset($_POST['pass2'])) echo $_POST['pass2']; ?>" /></p>
<p><input type="reset" name"clear" action"clear" value="clear"/> <input type="submit" name="submit" value="Register" /></p>
</fieldset>
</form>
</body>
</html> -
Thanks HartleySan - I've started a new thread for this question
-
Warning: Cannot modify header information - headers already sent by (output started at ... etc
-------
Hi everyone
I think I'm being thick, or not understanding this properly; but I'm modifying the registration script to have a couple of phases. So firstly username, password, email address, then if that's all ok a second page for more information.
Once registration has passed (and the fields are put into the mySQL database) then the second page continues with more info that I can link into the other tables.
Problem is I'm trying to pass the unique email address or the user_ID (don't care at this stage which until I can get it working). I'd like to open a session ideally once the user has submitted the form and the data has been written to the database.
That's when the WARNING comes.
Note - it even does it if it's line 1 of the code.
I am being thick aren't I?
Can someone explain a little further please?
Thanks
-
Yes thanks I did. I managed to get the code working in the end; but then I figured it was also open to a number of problems. So I created 3 select fields. Date / Month / Year.
Then made this code
if ($_POST['udobdate'] == '' && $_POST['udobmonth'] == '' && $_POST['udobyear'] == '') {$errors[] = 'You forgot to enter your Date of Birth.';} else {$udob = $_POST['udobyear'] . '/' . $_POST['udobmonth'] . '/' . $_POST['udobdate'];$dob = mysqli_real_escape_string($dbc, $udob);}That's done the trick. I'll probably refine it a little more but in terms of functionality - works a treat.Now I'm trying to figure out cookies and sessions that lovelyWarning: Cannot modify header information.problem.Even when the cookie is at the top of the page - before any code. Unless of course I'm misunderstanding the book. -
update to this.
I've tried using the php function date_format($STRING IM USING, YY/MM/DD). I get the SQL error that there's also a time stamp expected.
I've thought about creating three fields on the form. Year, Month, Date - then concatenating the results into a single string to put into the database. That seems to have a long code base though. Firstly, validating that each of those fields isn't empty; is numeric and then finally bundling them all together.
Would welcome ideas
-
Hi all
Please excuse the newbie question, but am struggling a little. I'm reading the book and I'm also trying to put the practical lessons into play chapter by chapter into my own project. Hence the question.
I've a form with a 'Date of Birth Field' - now I'm in the UK and the format is DD/MM/YY. In MySQl it's YY/MM/DD right? How do I get it to reverse the date?
Thanks in advance..
Struggling A Little With Ifs
in PHP and MySQL for Dynamic Web Sites: Visual QuickPro Guide (4th Edition)
Posted
Thanks Hartley - for some reason, when I use a different operator then the whole thing fails. Could it be that the field in the SQL database is UQ that there can never be more than 1?