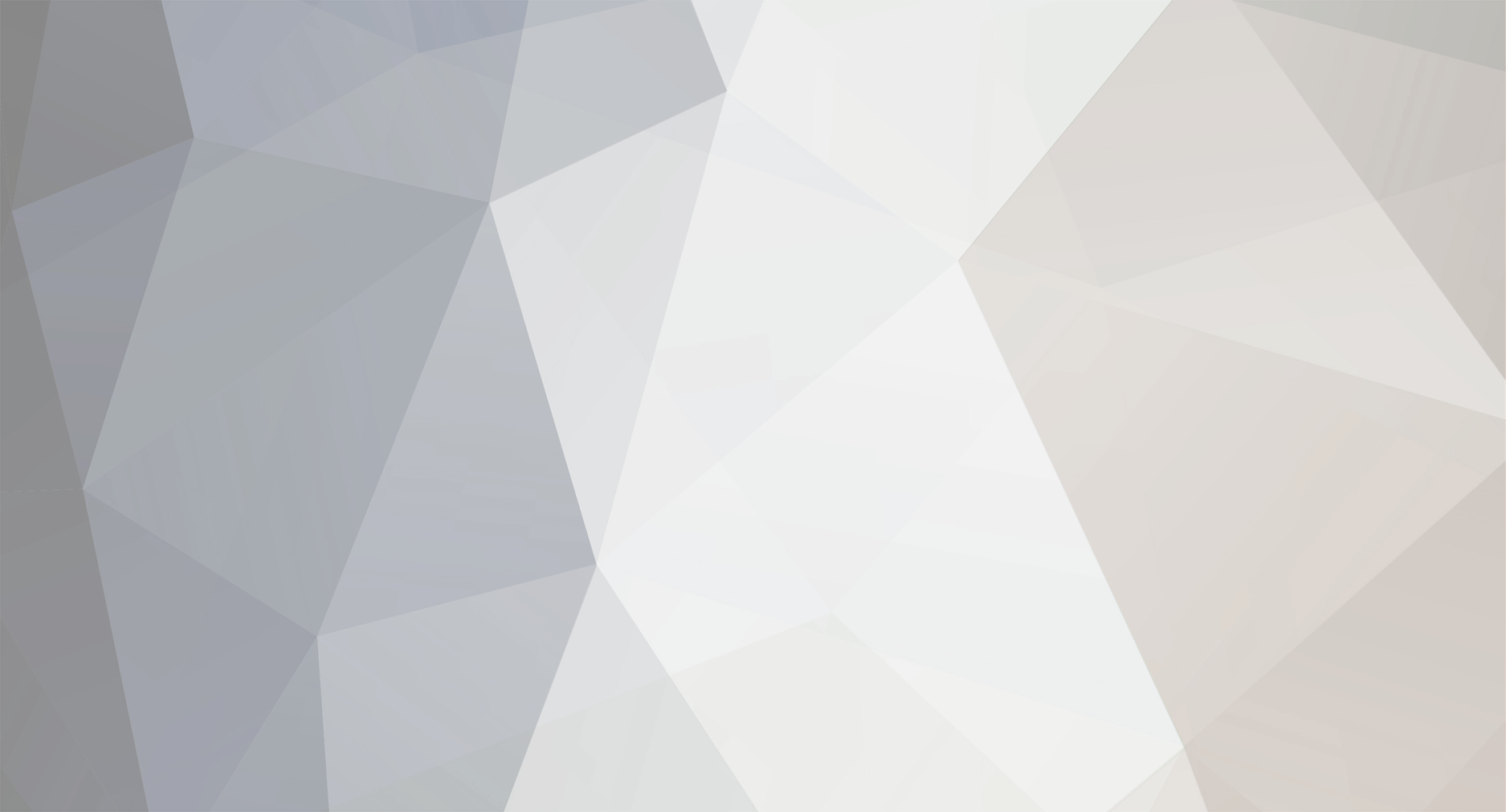
HartleySan
Members-
Posts
3047 -
Joined
-
Last visited
-
Days Won
243
Everything posted by HartleySan
-
Js Event Handling
HartleySan replied to HartleySan's topic in Building a Web Site with Ajax: Visual QuickProject
Well, my code is purely demonstrating a concept. It's not actually useful as is. Basically, I have a bunch of DIVs being generated on the page load. By clicking on any one of the DIVs, details regarding that DIV are displayed. However, along with the DIV info being loaded, left and right arrows are also loaded, which when clicked, need to go to the previousSibling/nextSibling DIV. As such, I wanted to be able to do something like (and this is pseudo code): for (x iterations) create DIV node add to DOM DIV.onclick = someFunction; . . . (And once DIV information is displayed with the arrows) leftArrow.onclick = previousSibling.someFunction; rightArrow.onclick = nextSibling.someFunction; I hope that pseudo code makes sense. Also, I can already get it to easily work by passing a parameter to the someFunction function, but I was wondering if it was possible without having to pass a parameter and just using this in someFunction. Anyway, I have gotten it to work as follows (just the code for the left arrow): document.getElementById('leftArrow').onclick = function() { someFunction(document.getElementById('the-current-DIV-node-whose-info-is-displayed').previousSibling); }; Well, I hope that's semi-clear. Anymore than that, and I'd have to start burying you in unnecessary amounts of code, which aren't relevant to the issue at hand. -
I have created a very basic HTML page to illustrate a problem I am having. Feel free to copy it into a text editor and create your own HTML page to understand my problem below. <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01//EN" "http://www.w3.org/TR/html4/strict.dtd"> <html lang="en"> <head> <title>Event Test</title> <meta http-equiv="Content-Type" content="text/html; charset=utf-8"> <script type="text/javascript"> window.onload = function() { document.getElementById('button1').onclick = toggleMe; // button2 event goes here. }; function toggleMe() { (this.style.background == 'red') ? this.style.background = 'white' : this.style.background = 'red'; } </script> <style type="text/css"> #button1, #button2 { border: 1px solid red; height: 100px; margin: 10px; width: 100px; } </style> </head> <body> <div id="button1"></div> <div id="button2"></div> </body> </html> Basically, I want to assign an onclick event to button2 that will cause button1 to be toggled. Basically, regardless of whether you click on button1 or button2, only button1 will toggle. Is this possible? I was originally thinking that the following would work: document.getElementById('button2').onclick = document.getElementById('button1').onclick; But that's essentially the same as saying the following: document.getElementById('button2').onclick = toggleMe; As such, it doesn't work. Is there any solution to my problem? Thank you.
-
Larry, you are absolutely right about there being a common ground among programming languages, and to some degree, once you've learned one of them, you've learned them all. Nevertheless, the average non-technical manager doesn't understand that, and will look for people with knowledge of a specific system/language, which is why it's important to have studied them all, I think. Anyway, back on the topic of your JS book. I'll be honest, I couldn't wait, so I went out and purchased O'Reilly's 900-page behemoth of a JS book. It's definitely a good book, but it's more a reference book than one you sit down and read cover to cover (which I actually did for whatever reason). One thing I think that's important to touch on with a JS book in the near future is the advent of JS 2.0, and the OOP paradigm. As it stands, the O'Reilly book covers the current version of JS (1.5/1.6), and talks about pseudo-classes (i.e., prototyping, etc.), but even that book acknowledges that everything will change as of 2.0. Anyway, I'll still buy your JS book, if you ever bring it out. On another note, I read a story the other day about a first-time Web dev book writer who wanted to get his name out there, so he had a printed copy of his book on Amazon, which sold for about $20, and then he had a free, PDF copy on Amazon. He found that for every 400-or-so customers that downloaded his free PDF version, one would buy his printed edition. He still made some decent money from it though, and you gotta figure that you're in a much better position than he is. I mean, let's put it this way, type "PHP" into Amazon, and see who has the top-rated book.
-
My current regex conundrum is as follows: I have used the following regex pattern to capture all the HTML between a bunch of pre tags (of which there are many sets): /(?<=<pre>)([\s\S]+?)(?=\<\/pre>)/ All the content (the HTML between the pre tags) is stored in group reference $1. I can output the results as follows: echo preg_replace('/(?<=<pre>)([\s\S]+?)(?=\<\/pre>)/','$1',$content); However, because the HTML contains all sorts of angle brackets, etc., you can't actually see anything. As such, I want to run the content (i.e., $1) through a function like htmlentities as follows: echo preg_replace('/(?<=<pre>)([\s\S]+?)(?=\<\/pre>)/',htmlentities('$1'),$content); But this doesn't work. Is this even possible (what I'm trying to do), or is it just that my syntax is no good? Thank you all in advance.
-
Yeah, I know jQuery makes it simple; almost too simple for my tastes. What I mean by that is that I want to challenge myself. Anyway, if I use two expressions, one to grab the id="2" ul, and one to parse the content, it's pretty simple. First I do a substring match with the following regex: <ul id="2">[\s\S]*?<\/ul> And then I simply do I global search for the li tags, as such: <li> Well, the pattern is /<li>/g, but the PHP functions work differently. Anyway, my point is, that's a piece of cake, but I'm thinking that where two expressions suffice, there must be a way to do it with just one.
-
Also, I should mention that the reason I'm doing this is that I want to be able to dynamically count the number of li tags within certain uls. The other trick though is that I don't know how many lis there actually are, and it changes all the time. I suppose it would have been helpful if I had better explained the situation at the start. Sorry.
-
That's a good point, except I don't know how to change a string containing HTML data into XML data. Do you? Also, the thought had crossed my mind of doing one regex search to grab the substring <ul id="2">...</ul>, and then parsing within that, but I suppose I'd rather just use one regex, if possible...if only for a challenge.
-
Perhaps what I'm trying to do is not possible with regexes, but I'm gonna ask anyway, as more likely, I'm just incapable of thinking of a decent regex. Let's say we have the following code within an HTML file: <ul id="1"> <li>List item</li> <li>List item</li> <li>List item</li> <li>List item</li> <li>List item</li> <li>List item</li> </ul> <ul id="2"> <li>List item</li> <li>List item</li> <li>List item</li> <li>List item</li> <li>List item</li> <li>List item</li> </ul> <ul id="3"> <li>List item</li> <li>List item</li> <li>List item</li> <li>List item</li> <li>List item</li> <li>List item</li> </ul> I want to come up with a regex that'll capture all six <li> tags within the id="2" ul. Is this possible?
-
I honestly have no idea what is going on, as I'm grabbing HTML from an external HTML page that is clearly marked as Shift JIS, but when I use that encoding and output the HTML to my page, it's all garbled. The real kicker though is that if I use some sort of regex to grab a piece/pieces of data from the external HTML, and output that regex-processed data to the screen, even though the content is in Japanese, it displays perfectly fine. I am truly confused.
-
I have always loved those epiphany moments. It's like the natural high that programmers get. Congrats on finishing your page. Even after seeing your page, it took me a second to figure out what was going on, but I finally figured it out. I guess you just wanted to point out spelling mistakes, etc., right? Anyway, it seems to work well, although honestly, I'm still not sure why you're using PHP and Ajax, but all the same. If you don't mind posting your final code sometime, I wouldn't mind critiquing things a bit (and I'll try to be nicer this time).
-
Bennie, you're killing me, man. First off, I'm no veteran. I have asked enough of my own questions on this forum to make Larry's head spin. I apologize if I came off as insulting, but frankly, you're not listening. First off, Larry's book talks in plenty of detail about how to cycle through an array. Matthaus also clearly outlined it in his first reply to your question. Again, I can only reiterate this so many times: You do NOT need PHP for what you are doing. If you are simply reading sentences from an array in the code itself, JavaScript is perfectly fine. Also, the code I gave you is a perfect starting point. However, your code is way, waaaay over the top and out of control. If you want to help people like yourself that want to do these sorts of things, please listen to the people that know what they're talking about and try to learn a thing or too. It's fine to ask questions if you don't know the answer, but please don't come in here with that attitude. I really do understand your frustration though with scouring the Web, and never being able to find a straight answer from the "pros". I still experience that all the time. The fact of the matter though, is that many people who are good at coding are horrible at explaining things. That's why Larry is such a savior. Anyway, back to your code. The code I gave you practically does everything you want. With a few extra lines of some code and some CSS styles, you will have exactly what you're looking for. If there is some part of my code you don't understand, please ask. But you have to recognize the value in having a script that is one JS function, one event assignment and two lines of code in the body to get the job done, as opposed to the three scripts you have interacting for no reason whatsoever. You also have so many unnecessary variable assignments that I don't know where to begin. Look, I'm trying to be nice and helpful, but you gotta listen a bit more, or I'm gonna stop trying. And while I'm the main one replying to your threads, I seriously doubt that anyone else on this forum could read your posts and clearly understand what you're trying to do. So I'll ask one more time: What do you want to do? You want to take sentences defined in an array in the code, place them in a text area one at a time (tags included), edit them, and then hit the next button to load the next sentence and store the revised one back into the array, right? If that's the case, please say so, and I'll revise my code above to help you out.
-
Not sure if there are any experts on the topic, but I'm simply trying to load Japanese text from a text file and print it to the screen, but something is obviously wrong with the encoding/charset. I really am at a loss with this sort of thing. Basically, the file is save with Shift JIS encoding (and the text looks fine in the file), so I tried setting the following meta tag and then outputting the data, but it comes back all garbled: <meta http-equiv="Content-Type" content="text/html; charset=shift_jis"> If anyone could show me the error of my ways, I would be most appreciative.
-
Bennie8, please do me a favor and stop posting long blocks of code. It's not helping any. For starters, I'm not going to write your code for you. We're all here to help and give you pointers, not to do everything for you. With that said, I have looked at your code, and while your effort is admirable, and I'm happy that you're trying so hard, I can't help but feel like you're just randomly throwing pieces of code together you have gotten from other places, and now you can't figure out why the code is not working. Really, I cannot stress this enough: You really need to take a step (or two) back, and rethink things. For starters, I still don't completely understand what you're trying to accomplish. I mean, it seems like your trying to display different parts of an HTML page, upon which you want to edit the content for any spelling/grammar mistakes, and then reinsert the corrected sentences somewhere. That's about the extent of what I can imagine. So from here on out, instead of posting huge blocks of code, let's take this one step at a time, taking baby steps along the way, and make sure you understand what you're doing while you're doing it and slowing building your site, okay? Fair enough? Now, before we go any further, could you please explain to me in detail exactly what you want to make? I need all the details about what you want, where your data is coming from (e.g., a text file, a database), who your audience is going to be, what functionality you think the site should have, etc. After everyone that's here trying to help you is on the same page, we can proceed, okay? Cool. And again, please don't rush things. Perhaps you have a deadline you have to meet, but rushing's not going to help, and it makes everyone on this board less likely to help you. We anxiously await your reply. Thank you.
-
Yeah, I usually use the JS debugger in Chrome. (Chrome rocks!) But I was at work when I wrote that code, and aside from being busy and not having a decent JS resource handy, I am stuck in IE6 in XP at work. (Oh, it sucks!) Anyway, I checked my big ol' JS resource when I got home, and instantly saw the problem with my code. The revised code is below, and I have confirmed that it does work. Note that I added some other tags that are good to have in any HTML document. <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01//EN" "http://www.w3.org/TR/html4/strict.dtd"> <html lang="en"> <head> <meta http-equiv="content-type" content="text/html; charset=UTF-8"> <title>Testing</title> <script type="text/javascript"> var i = 0; var typos = ['element1','element2','element3']; window.onload = function() { document.getElementById('txt1').onclick = showHint; }; function showHint() { document.getElementById('data-here').innerHTML = typos[i]; i++; } </script> </head> <body> <p>For Next Sentence: <button id="txt1">Click</button></p> <div id="data-here"></div> </body> </html>
-
All right. I'm gonna try and help you out a bit more here. I'm gonna write some code that "shuold" work (although I'm not able to test it now). HTML file <html> <head> <script type="text/javascript"> var i = 0; // This is your global counter variable, which you will increment each time. // If you decide to put your JS in an external file, make sure the scope of your variables is okay. var typos = ['element1','element2','element3']; // I am honestly still confused, as you don't seem to need PHP or Ajax. // If you are grabbing the info from a database, then please give // us some more info about that. Otherwise, we can't help. // I am just declaring typos globally here to show off the "loop" mechanics. // Note that you're not really looping though. window.onload = function() { document.getElementById('txt1').onclick = showHint(i); }; function showHint(i) { document.getElementById('data-here').innerHTML = typos[i]; i++; } </script> </head> <body> <p>For Next Sentence: <button id="txt1">Click</button></p> <div id="data-here"></div> </body> </html> Please note the small amount of code necessary to do what you wanted. Please let me reiterate this point: You do not need to use PHP or Ajax unless a database is involved, in which case, please tell us. Otherwise, very simple JS and HTML (without a form) will suffice. Again, please let us know if there's something you don't understand.
-
Delaying An Ajax Call
HartleySan replied to David's topic in Building a Web Site with Ajax: Visual QuickProject
Yeah, I know. I know the code is just pre'ing everything, but it would have been nice. Oh well. -
Yeah, walking away does help. I have been stuck on problems for days, and then when I come back to it with a clear head, I instantly spot the problem. Happens to all programmers. Glad you solved your problem though. I kinda wish it was one of those "once bitten, twice shy" sort of things, but I seem to have to make the same mistake many a-time before I learn my lesson.
-
Well, the answer is pretty obvious. You're sending 1 to the JS script, and subsequently to the PHP script, every time. That's gonna get you the "1" value (the second element in the array) every time. The simple solution is to make a global i counter variable in the JS script, start it at zero, and increment it by 1 every time the button is clicked. Also, be sure you send that i counter variable, and not 1, to the onclick function. That should solve your problem. One thing you need to be aware of though is the scope of variables. I noticed that you're using all kinds of variables that don't need to be used, and have no effect on anything, like the $j in the PHP script. My point is, while sending the global i counter variable to the onclick function should solve your problem for now, I would encourage you to continue to read Larry's book carefully, so that you can better understand how PHP (and other programming languages for that matter) work. (Kinda that whole "teach a man how to fish" thing.) Anyway, good luck. Edit: Also, your HTML is invalid. When you have the time, take a step back and learn things from the start again. It'll make your life so much easier.