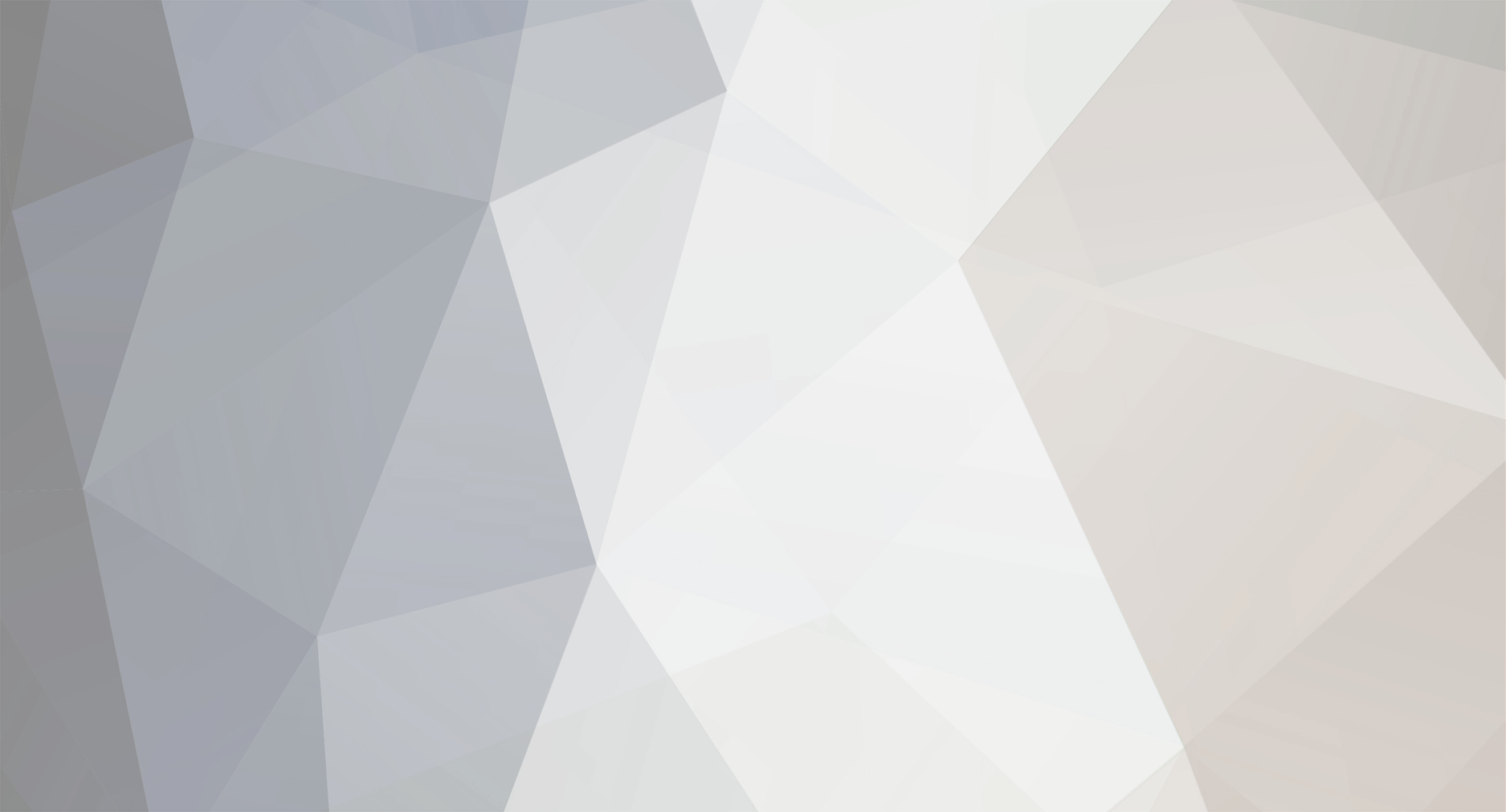
Jonathon
-
Posts
1064 -
Joined
-
Last visited
-
Days Won
55
Posts posted by Jonathon
-
-
Hi,
After reading the pars on widgets I need to use them more for easy pagination and sorting. So i'm rewriting some functionality. I currently have this code in my Area Controller.
public function actionIndex($id) { //->with('areaItems')->find('t.id=:id', array(':id'=>$id)) $dataProvider=new CActiveDataProvider('Area', array( 'criteria' => array( 'with' =>'areaItems', 'condition' => 't.id=:area_id', 'params' => array(':area_id'=>$id), ) )); $this->render('index',array( 'dataProvider'=>$dataProvider, ));
What this does is take the url say localhost/area/2/birmingham and gets all the items from the Items table that have the area_id (FK) 2.
I've printed the returned object and relations and I'm getting the right information.
In my index.php view file I have the default code
<?php $this->widget('zii.widgets.CListView', array( 'dataProvider'=>$dataProvider, 'itemView'=>'_view', 'sortableAttributes'=>array('date_added') )); ?>
"date_added" was me just playing with the sortable aspect of the widget. However I have a small problem, I'm only able to use the column names of the primary model it seems, in this case Area(). What i'd like to be able to do is use column names from the related model such as "price". How would I do this? Have I got things in the wrong model (Again)?
Additionally my index.php was made up of this loop
// There are items, iterate through model foreach($model['areaItems'] as $row):?> // do stuff to present data
I took this out of the index.php view and put it within the _view.php. As I felt that was the right place for it?
Thanks
-
Hi Larry,
Thanks for the nice words. I forgot about the create functions that come built into Yii, so I'm glad you pointed that out to me. I should really have twigged when I realised I had two sets of rules(). Anyway, thanks, I had a re-jig of things and came up with the following.
User Model/* * function to register new user internal variables */ public function newUserRegistrationVariables() { // Internally assigned variables $this->active = md5(uniqid(rand(), true)); $this->date_modified = NULL; $this->date_created = new CDbExpression('NOW()'); return array($this->active, $this->date_modified, $this->date_created); } }
User Controller
public function actionCreate() { // new User model $model=new User; // Bring internal registration variables from model() $registrationInternalVariables = $model->newUserRegistrationVariables(); // Uncomment the following line if AJAX validation is needed // $this->performAjaxValidation($model); if(isset($_POST['User'])){ $model->attributes=$_POST['User']; // Validate date from form using rules() if ($model->validate()) { $model->first_name; $model->last_name; $model->email; $model->pass = hash_hmac('sha256', $model->pass, Yii::app()->params['encryptionKey']); $model->active = $registrationInternalVariables[0]; $model->date_modified = $registrationInternalVariables[1]; $model->date_created = $registrationInternalVariables[2]; if($model->save()) { // Everything was saved ok return success message Yii::app()->user->setFlash('register-success','Thank you! An activation email has been sent to your email address.'); } } } $this->render('create',array( 'model'=>$model, )); }
So I hope that's a secure and better way to go about it
Thanks
Jonathon -
Not your fault!
-
I sadly forgot to copy it before posting last night which annoyed me as it wasn't the first time.
-
Hi Larry
I've experienced this a few times, but it is monumentally more upsetting when you have a large post. But I'll write a big post have several blocks of code and it will just chop most of the message away. If I had to guess I'd say it tends to be when there is a use of [ code ] blocks. But I can't be sure. Obviously this isn't your fault, but I thought I'd let you know.
Thanks
Jonathon
-
Right, I'm trying to put together some code to register a user in Yii.
I just wanted some feedback on it for problems or general logic within the whole Yii framework. This is what I did.
I created a new file called register.php
<?php /* @var $this SiteController */ /* @var $model RegistrationForm */ /* @var $form CActiveForm */ $this->pageTitle=Yii::app()->name . ' - Register'; /*$this->breadcrumbs=array( 'Login', );*/ $this->layout = 'sidebar'; ?> <div class="search"> <div class="form-center span5"> <h1>Register</h1> <div class="form"> <?php $form=$this->beginWidget('CActiveForm', array( 'id'=>'registration-form', 'enableClientValidation'=>true, 'clientOptions'=>array( 'validateOnSubmit'=>true, ), )); ?> <div class="row"> <?php echo $form->textField($model,'first_name', array('placeholder'=>'First Name')); ?> <?php echo $form->error($model,'first_name'); ?> </div> <div class="row"> <?php echo $form->textField($model,'last_name', array('placeholder'=>'Last Name')); ?> <?php echo $form->error($model,'last_name'); ?> </div> <div class="row"> <?php echo $form->textField($model,'email', array('placeholder'=>'Your Email')); ?> <?php echo $form->error($model,'email'); ?> </div> <div class="row"> <?php echo $form->passwordField($model,'pass', array('placeholder'=>'Password')); ?> <?php echo $form->error($model,'pass'); ?> </div> <div class="row buttons"> <?php echo CHtml::submitButton('Submit', array('class'=>'btn btn-primary')); ?> </div> <?php $this->endWidget(); ?> </div><!-- form --> </div> </div>
saved it in my views/site folder.
Then I created a RegisterForm.php that I basically copied from the LoginForm.php and edited.
<?php /** * RegistrationForm class. * RegistrationForm is the data structure for submitting * user data. It is used by the 'registration' action of 'SiteController'. */ class RegistrationForm extends CFormModel { public $first_name; public $last_name; public $email; public $pass; public $active; /** * Declares the validation rules.
* The rules state that first name, last name, email and pass are required, * email must be valid email. * email must be unique using User class. */ public function rules() { return array( // username and password are required array('first_name, last_name, email, pass', 'required'), // trim date for first names, further validation? strip_tags? array('first_name, last_name, email, pass', 'filter', 'filter' => 'trim'), // email must be valid email array('email', 'email'), // email must be unique and use User class array('email', 'unique', 'className' => 'User'), // default for date created ); } /** * Declares attribute labels. */ public function attributeLabels() { return array( 'first name'=>'First Name', 'last_name'=>'Last Name', 'email'=>'Email', 'password'=>'Password', ); } }
And saved that in my models folder.
Thenin my site controller I added the following action
/* * Displays and handles the registration process */ public function actionRegister() { $model=new RegistrationForm; $newUser = new User; // if it is ajax validation request if(isset($_POST['ajax']) && $_POST['ajax']==='registration-form') { echo CActiveForm::validate($model); Yii::app()->end(); } // collect user input data if(isset($_POST['RegistrationForm'])) { $model->attributes=$_POST['RegistrationForm']; if ($model->validate()) { $newUser->first_name = $model->first_name; $newUser->last_name = $model->last_name; $newUser->email = $model->email; $newUser->pass = hash_hmac('sha256', $model->pass, Yii::app()->params['encryptionKey']); $newUser->active = md5(uniqid(rand(), true)); $newUser->date_modified = NULL; $newUser->date_created = new CDbExpression('NOW()'); //$newUser->live = 1; if($newUser->save()) { // Everything saved, redirect $this->redirect(array('site/index')); } } } // display the register form $this->render('register',array('model'=>$model)); }
It does work, but I wanted an opinion of it it has any potential security flaws/issues or if the logic is in the wrong files/locations etc. You may see some of my own questions in there like "strip_tags()?" for example. Also I wondered about the rules() Those rules are in the RegistrationForm.php but I also have rules() in my User model. This would appear to be a wrong thing to be occurring. However, I wasn't sure what the best way to fix this was?
Thanks in advance
Jonathon
-
-
Hello,
I'm just about to build (hopefully) my registration form for my Yii project. I wasn't sure though if you use hash_hmac() and sha256 and just wanted to confirm that it returns a string of 40 characters? Is that right. Just so I can set my DB table to CHAR (40).
I take it the key can also be any length and combination of any kind of characters?
Thanks
Jonathon
-
-
No problem. I bet you will be a happy man for for the new formatting changes too. So thanks for getting back to me.
-
Just saw your tweet. So question answered. Thanks
-
Just a quick question Larry, what would you say is the ETA on the next update to the Yii book? Apologies if you've a) mentioned this already or
are sick of being asked about it
-
Seems there is talk of it being packaged with Yii 2.0 too. http://www.yiiframework.com/forum/index.php/topic/28222-embedded-css-framework/
-
I've been reading about Foundation 4. IT was released today I think http://foundation.zurb.com/ I hear very good thinks about it. Maybe some of you will enjoy it.
-
Hello,
Just having a look through my php.ini records, my php.ini file has an error_reporting value of
error_reporting = E_ALL | E_STRICT
Which I always thought was the best setting to develop a site on.
However when i run phpinfo() error_reporting has this value '32767' - which is All errors and warnings, as supported, except of level E_STRICT prior to PHP 5.4.0.
http://php.net/manual/en/errorfunc.constants.php
So my question is why does phpinfo() not seem to include the strict errors and what value would I need to change to make it also include the strict values?
I know I could use
<?php error_reporting(-1); ?>
But i'd prefer to use php.ini if possible.
-
NOT NULL means a row cannot have a null value. In your case user_id means that it updates itself as you have set auto increment. Meaning you can leave that field blank and the DB will do the rest.
To clarify the default options in phpmyadmin are None, As defined, NULL, current timestamp.
You are looking at two different areas. The NOT NULL field is a check box and not an available option under DEFAULT
-
1
-
-
NOT NULL isn't a default. It's saying that the row cannot have a NULL value.
-
-
I would suspect you need to start your command with "php" first.
so
php C:\xampp\yii-1-1-13\framework..........rest of command
-
That's an aspect of the HTML5 doctype more than anything else though :-)
-
To spice it up, you don't necessarily need a JS library you could just use hand coded JS to find out whether JS in enabled firstly then things like does the username meet requirements (i.e only letters and numbers). If not it can then be used to change the display of the form such as an error class that changes the input box to red to highlight that there is an error.
-
1
-
-
From the tutorial series? As in http://www.larryullman.com/2009/11/10/basic-model-edits-in-yii/ ?
Which part of code?
The rules() method is inside your model.
If this is the right article then it's your Employee Model.
-
1
-
-
I actually wrote a reply to this with that allowable tags part for strip tags in it, but I re-read your question and assumed it wasn't what you were after. Glad you sorted it.
-
I always browse FB in HTTPS too. I found it interesting that Google said moving Gmail to pure SSL didn't harm performance much. However their servers probably rock.
Registration In Yii
in The Yii Book
Posted
Thanks Larry