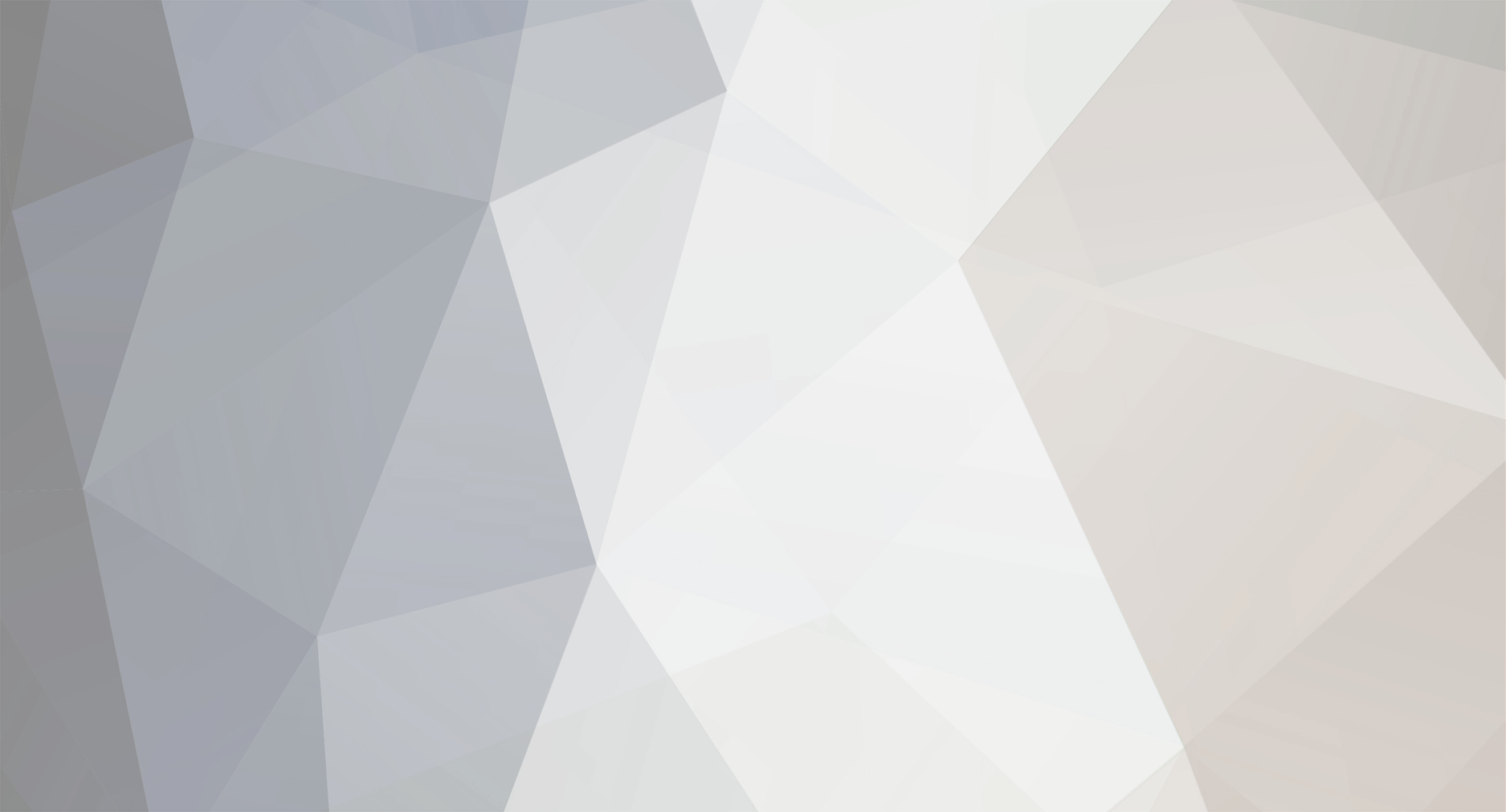
Antonio Conte
Members-
Posts
1084 -
Joined
-
Last visited
-
Days Won
126
Everything posted by Antonio Conte
-
Several ways to do this. I would add result to an array and use for-loops to get half in each section. Btw. With this example, I pretend you have rows called "id" and "name" in your DB. You just switch that to what you use. // Add result to a new array called $rows while ( $row = mysqli_fetch_array($result, MYSQL_ASSOC) ) { $rows[] = $row; } $size = count($rows); // Get size of rows array $half = floor($size / 2); // Get half the row size, round down // Get first section for ( $i = 0; $i < $half; $i++ ) { echo $rows[$i]['id'] . ' '. $rows[$i]['name']; } // Get second section for ( $i = $half; $i < $size; $i++) { echo $rows[$i]['id'] . ' '. $rows[$i]['name']; } You might need to change the last loop to $i <= $size. If one row gets dropped, do that. Remember that this way would give you 50 rows in each section if 100 rows are returned. You need to use LIMIT in your queries or something like that.
-
Approaching The Dbms. Tips, Tricks And Tools For The Trade
Antonio Conte replied to Antonio Conte's topic in Social
Dude. Not to get pissed here, but you really need to start reading. This is posted in SOCIAL. It's meant to share possibilities, not to discuss fully blown ideas you'll replace your existing systems with because "they are bad ass", "they really scale" or "they make water into wine". You are right. I just want to code, expand my horizons and know more about existing technologies. THAT is what this thread is about. As stated, I like to try new things out on small personal SIDE PROJECTS. I won't be using large code bases on anything major, therefor I take time to experiment. If I should like the approach taken by these code bases, and I've learned how the works and tested them, then MAYBE I'll use it on a large project later on. "Sometimes questions are more important than answers," Nancy Willard -
This is not related to YII. What you seek to do should primarily be done with CSS as this is styling of presentation. Any menu structure could be made by HTML and CSS, while the content MAY be provided by a db. Short version: HTML and CSS. Not YII. Not PHP. Not Javascript. (Unless loading HTML with JS)
-
Approaching The Dbms. Tips, Tricks And Tools For The Trade
Antonio Conte replied to Antonio Conte's topic in Social
Hehe. No problem. The only reason for using CI is that I've used it before. I didn't want to learn a new one for this project. I can tell your right now that it doesn't take much to be better than CodeIgniter. I knew CI. It was a safe bet. Choice made. Also, Edward. I did not ask how to perform a task. I can read documentation perfectly fine. I've never used YII before, though, and I know several users here has done that. As this thread is related to approaching the DBMS, I asked what they thought about YII's active records or query builder. I want to know their EXPERIENCE using these tools. I did not ask how it works. You really need to read what I write properly, my friend. -
Haha. Ok. Guess PHP is really retarded sometimes. Good example on why I'm starting to hate PHP. My new readir function: /** * Read entry from directory handle * * @param resource $handle The directory handle resource previously opened with opendir(). * @return unsure Returns the entry name on success or FALSE on failure. * (But if something weird happens, which we don't really know what is, * you may expect "." or ".." as return values too. * We don't really know why. DON'T ASK!!!) * @see http://stinky-code.com */ function readir( $handle ) { return iHaveNoIdeaWhatIamDoing("dog.jpg"); }
-
if (($file !== '.') && ($file !== '..')) { This line is really bad, and won't work. You are basically checking if the filename is dot or double dot. I don't really understand why you'd ever need that. If you want to make sure your files has an extension, is hidden (.name-files) etc, you need another check. A very simple one for hidden files. $max = 5; // Max number to output $count = 0; // The actuall count while (($file = readdir($handle)) !== false && ($count++ < $max) ) { if ($file[0] === ".") { $count--; continue; } /* If first char is "." (hidden file), continue loop */
-
Approaching The Dbms. Tips, Tricks And Tools For The Trade
Antonio Conte replied to Antonio Conte's topic in Social
Active records is a pattern where you map values to the object's properties. One row in a table/view will correspond to one object, and the relationships will also be objects. The normal approach looks something like: $user = new User(); $user->id = 1; $user->username = "Thomas"; $user->email = "email@email.com"; The objects will then have methods for adding, usually called save(), finding with find() and etc. The point is of course that this approach will limit the amount of knowledge needed for working with a DB, as it's abstracted into a more common form for many programmers. There's both advantages and disadvantage to this approach, the largest disadvantage often being memory use and speed. The benefits will, at least sometimes, result in shorter development time. These solutions are often really clever things that needs access to a lot of meta data for your tables to work. ORMs are also known for producing sloppy DB design, (normalization, relations, etc) but that is not really the ORMs fault. An ORM should only make DB interactions easier, (as this thread is about) not build your DB design for you. CodeIgniter has a different approach to this, and while it does not really feel wonky or bad, I just don't like it. My biggest problem is the same as with several other CodeIgniter (CI) libraries/helpers. They offer to many weird methods, and the methods often have bad design in my opinion. They offer several methods for SELECT, FROM, JOIN, WHERE, GROUP BY and ORDER BY, but seem more interested in short syntax than clever methods. In the end, you end up with weird method SQL, that won't work without second or third parameters with false, or null. It's not hard to understand or difficult to use, I just don't see the gain. How is this...: // Select part of query $this->db->select('brand.brand_id AS make_id'); $this->db->select('model.model_id AS model_id'); $this->db->select('brand.brand_name AS make_name'); $this->db->select("CONCAT(brand.brand_name,' ',model.model_name) AS model_name", false); // Join data $this->db->from('eddy_cars_brands AS brand'); $this->db->join('eddy_cars_models AS model','brand.brand_id = model.model_brand_id', 'left outer'); $this->db->order_by('brand_name'); $this->db->order_by('make_name'); Really better than this...: SELECT brand.brand_id AS make_id, model.model_id AS model_id, brand.brand_name AS make_name, CONCAT(brand.brand_name,' ',model.model_name) AS model_name FROM cars_brands AS brand LEFT OUTER JOIN cars_models AS model ON (brand.brand_id = model.model_brand_id) ORDER BY brand_name, make_name One of the benefits of Active Records is being able to do something like this: // Add a User public function add( User $u ) { $this->db->insert('table', $$u); } // Update a User public function modify( User $u ) { $this->db->insert('table', $$u); } // Delete a User public function remove ( User $u ) { $this->db->where('user_id', $u->get_user_id())->delete('table'); } Active records is simple supposed to easy your work, but you need to expect some drawbacks too. I don't really think it's a good solution unless you know what you're doing. -
First issue is very easy. Add something like this to your while loop: (This will work) $max = 5; // Max number to output $count = 0; // The actuall count while ( ($file = readdir($dh)) !== false && ($count++ < $max) ) {} I'm not a devil on string splitting, but I'm guessing you could use substring, explode the string into parts, use ucwords and a lot of other functions to format it. Please note $count is increased during the loop. If you want it to start $count on 1, use <= as comparison instead.
-
Approaching The Dbms. Tips, Tricks And Tools For The Trade
Antonio Conte replied to Antonio Conte's topic in Social
Seems we are starting to get on the right track now. Seems like a lot of you use YII, or at least will consider using it with the new release. What do you think of YII's Active Record? Do you use that our the queryBuilder in your projects? What do you like/dislike it for? Have been working with CodeIgniter for a couple of months now. Still loath their "active record" implementation. Will be good to get this project released and never touch that again. It's not that it's not working. I just don't like how you're supposed to use the damn thing. #first-world-problems -
Without knowing the question really good here, this sounds like a JOIN problem. Try variations of LEFT or RIGHT OUTER JOIN instead of the inner join on the size table. The main difference is that inner joins are exclusive (rows without joins are ignored) while outer will include those rows will null values instead. Try experimenting a bit. If you want help solving this, I need example data from the tables. (Paste two-three rows from each table)
-
<?php // Assign pages $pages = array( 'Home Page' => 'index.php', 'Register' => 'register.php' ); // Get the current page $current = basename($_SERVER['PHP_SELF']); ?> <nav> <ul> <?php foreach ( $pages as $desc => $page) { $active = ($page == $current) ? ' class="current"' : null; echo '<li'.$active.'><a href="'.$page.'"><span>'.$desc.'</span></a>' } ?> </ul> </nav>
-
As I told you, echo out $this_page. You'll then see you'll never get a match. You need a way to match that value with something in your pages array. For that to work, you need to be sure you are comparing the right values. You also do a foreach with $k and $v. Lets say index.php is active. That test will check if "front page" is equal to "something in you link structure." You would more likely check $v. Writing from mobile now, so I'm a little limited here.
-
Never heard of it. Sound interesting, but I do not have the time for such events right now. I'm currently working on a large car advertisement system for a company through school, and the deadline is coming shortly. We are soon to enter the user testing phase, with release not soon after. I work on it solo, so I have little time to spear right now. I will definitely give your guys a tour later on. It's in Norwegian, but I'll provide translations for you.
-
A Norwegian guy spoke about living abroad, and working in Silicon Valley, at JavaZone some weeks ago. He said San Fransisco is the "hip" town for startup companies, while Silicon Valley is extremely result oriented. Very interesting to hear how he got the job, what's expected of you, how you get a green card, etc. By the sound of it, you got to be prepared to work hard and long hours. I would be interested in working abroad now that I'm still young. Not that it's a real goal, but it would be a very fun experience. On that account, Scandinavia is pretty much the only place in Europa without economic crisis. Not very hard to get jobs here, and the salary is good. When I'm done with my education, I will definitely consider it, thought. What might draw me, if given the chance, is the possibility to do something you can be truly proud of.
-
Approaching The Dbms. Tips, Tricks And Tools For The Trade
Antonio Conte replied to Antonio Conte's topic in Social
I agree with every word, Jon. I'm not really interested in NoSQL because of speed or "scaling", that is the buzzword for NoSQL. It's not really those things that interest me, as the project in question is intended for fewer than 10 peoples. I'm interested in NoSQL, and MongoDB in particular, for the sake of learning and testing. Small projects are good for testing new things out. -
Approaching The Dbms. Tips, Tricks And Tools For The Trade
Antonio Conte replied to Antonio Conte's topic in Social
It has never been about database performance. I talked about relative performance considering I needed four joins to get four strings. The example is an illustration of how annoying assembling data that has been normalized can be. Of course, these data has relevance elsewhere, so it's not a bad solution. Just very annoying sometimes. It was a border land example — a worst case scenario to make an example of why I hate working with relational databases. The example was supposed to illustrate the difference between how you really use the data and how you save it in a DB. To conclude. DBMS systems don't really let you save your data the same way you use them. However, you can make your life easier by using some tools, like the Active Record pattern, including ORMs, creating wrappers to make this easier, or even switch to NoSQL. (And, I don't mean to direct anyone towards that. - But someone may have expirence to share with others). -
Approaching The Dbms. Tips, Tricks And Tools For The Trade
Antonio Conte replied to Antonio Conte's topic in Social
You are not getting the point here, guys. I am not seeking help solving actual problems. I'm wondering if anyone of you do anything to make your life easier? As an example, my DB wrappers allow you to group by a column. This mean I can get structures like these very simple: $result = DB::fetch_array('players', 'position'); // Returns array grouped by the column "position" $result = DB::fetch_objects('players', 'nation'); // Returns array of objects grouped by nation array( "keeper" => array( 1 => array( "name" => 'Gianluigi Buffon', "country" => 'Italy', ' ), 13 => array( "name" => 'Marco Storari', "country" => 'Italy', ' ), ), "defender" => array( 3 => array( "name" => 'Giorgio Chiellini', "country" => 'Italy', ' ), 6 => array( "name" => 'Leonardo Bonucci', "country" => 'Italy', ' ), ), ); The same wrapper will also return a single result array when only one row is returned. Usually, you need to pop from the array or use $result[0], which, again, I hate. My wrapper also build an internal result array. This means I can iterate trough the result several times if I need to. It also has internal escaping, so no need to worry about that. Starting to get me here? The thread is meant to help others discover tools for the job, not how to actually do the job itself. Regarding the Framework discussion, Edward, you could extend the framework with your own libraries or helpers. YII ships with a query builder, but you don't actually have to use it if you don't like it. The models should help you interact with your DB, but how that is done is up to you how to implement. -
Approaching The Dbms. Tips, Tricks And Tools For The Trade
Antonio Conte replied to Antonio Conte's topic in Social
Good post, Jon. As stated, I don't like relational databases from a programming standpoint. I was wondering what people here do to make their lifes easier in this regard, and not trying to say relation DBs are a bad/slow thing. My latest experience come from CodeIgniters active records class, which I hate. I've on the other hand heard great things about an Orm called RedBeam PHP. I have also developed several DB wrappers over the years myself to make working with relational DBs easier to work with. Thanks for a great post, Jon. Hope you'll answer this one as well. I've updated the original post to clearify some points. -
Hey, everyone. Please note that this thread is meant as a general purpose discussion thread. My thoughts are merely meant to get the discussion going. I want to create a general discussion thread about working with a database system from a programming standpoint. The thread is intended for discussing tools for abstracting the process of doing CRUD-operations against a database. I'm talking about Active Records, Wrapper Classes and Database Helpers. Please feel to talk about what you like here.