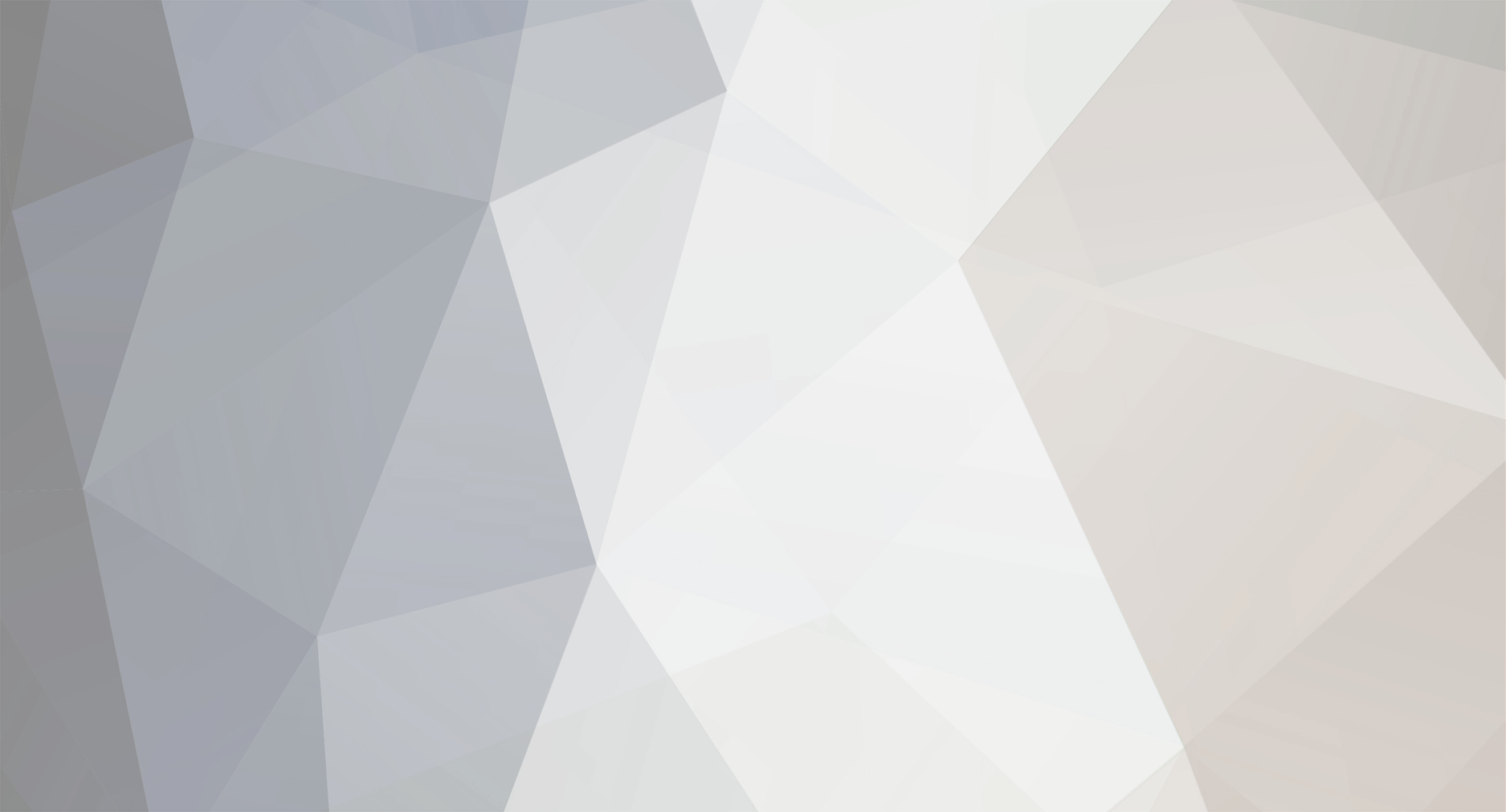
HartleySan
Members-
Posts
3047 -
Joined
-
Last visited
-
Days Won
243
Everything posted by HartleySan
-
Honestly, I think that a quick Google search would get you there, but array_search will return the key that corresponds to the first instance of a value in an array, and array_splice allows you to remove one or more elements from an array. For example: $arr = array('red', 'green', 'blue', 'yellow'); $key = array_search('blue', $arr); // Returns 2. array_splice($arr, $key, 1); // $arr is now array('red', 'green', 'yellow'). You could easily combine these two steps into an easy-to-use function as well: function remove_val_from_arr($val, &$arr) { array_splice($arr, array_search($val, $arr), 1); } $arr = array('red', 'green', 'blue', 'yellow'); remove_val_from_arr('red', $arr); // $arr is now array('green', 'blue', 'yellow'). That all make sense?
-
The whole point of recursion is that you call a function within itself, but the argument you send that function each time is different, thus allowing you to do things like advance through the hierarchy of a multidimensional array, regardless of how deep or complex it is. To that end, make sure you're passing the recursively called function the right argument each call. Here's a simply example: function list_array($arr) { foreach ($arr as $val) { if (is_array($val)) { list_array($val); } else { echo "$val<br>"; } } }
-
Stumped
HartleySan replied to selliottsxm's topic in PHP and MySQL for Dynamic Web Sites: Visual QuickPro Guide (4th Edition)
Let's take this discussion outside the forum for a minute. Please see the private message I sent you. -
Stumped
HartleySan replied to selliottsxm's topic in PHP and MySQL for Dynamic Web Sites: Visual QuickPro Guide (4th Edition)
You're comparing the return value of CURDATE (which I assume is the current date; where is that function defined?) with a MySQLi results object. It won't work. Do you understand what I'm saying? -
Stumped
HartleySan replied to selliottsxm's topic in PHP and MySQL for Dynamic Web Sites: Visual QuickPro Guide (4th Edition)
Just so you know, $r2 is not a date. It's a MySQLi results object. You need to first retrieve the date from the results object, then do the comparision. Add the following code before your if statement, and you'll see what I mean: echo '<pre>'; var_dump($r2); echo '</pre>'; -
Stumped
HartleySan replied to selliottsxm's topic in PHP and MySQL for Dynamic Web Sites: Visual QuickPro Guide (4th Edition)
Steve, could you please format your code using the <> button and give us only the relevant parts? Thanks. -
My main gripe with Git is that in my experience, people don't know how to properly use it, and thus cause all sorts of issues like doing a force push and overwriting all changes they hadn't themselves captured yet. (This happened several times at my last employer and caused us a ton of frustration and days of lost dev time. And it's so easy to do. If you simply force push something or even have your config set up to automatically force push without your knowledge, I guarantee this will happen.) With that said, I agree with Larry that there isn't much better out there than Git for large dev groups, and certainly, the version control is the most important thing. However, I still believe that in your case, as a single dev looking to maintain your codebase across Windows and Mac OS, a flash drive is sufficient and the easiest. Just my opinion though.
-
Yes, I agree that it's overblown, but I think the main reason it's a concern is because most people are not smart enough to create secure passwords. And more than that, the biggest problem is that people use the same or very similar passwords across all sites, meaning that if Bob's Online Comic Shop gets hacked and you have an account with them, then your Gmail, LinkedIn, etc. accounts are now also compromised. I personally have a pitiful password that I use for all accounts I don't care about, and then separate and unique passwords for accounts I do care about (e.g., Gmail). It's annoying, but that seems to be the world we live in. Edit: Larry, you and I posted at the same time I think, which is why I pretty much just repeated what you said.
-
BitBucket is pretty good from what I've heard. You should try it out. With that said, I honestly am not always that gung-ho about these "new-age solutions". For example, you can easily format a flash drive on Mac OS X to work on both Windows systems and Mac systems. With that alone, you can easily move files back and forth. Perhaps it's a bit more manual than you prefer, but really, how often do you have to move files between devices anyway? And on a side note, I had to use Git a lot at my last job, and frankly, it caused the team more headaches than it was worth in my opinion. I'm not saying Git is bad, but it's not the cure-all it's made out to be.
-
There are a lot of ways you can approach this, but because the data set will always be fairly limited, I would get all the data for all the possible job categories and skills from the DB at the start, and then use solely JavaScript to render everything from there. Furthermore, I would likely render all of the job skills drop-downs from the start, but have them all hidden, and when the user selects a job category, unhide the corresponding job skills drop-down, and hide all the others. To start with, I'd organize the data into a JS object like the following: var jobData = { categories: [ '--', 'Agriculture', 'Engineering', ... ], skills: { agriculture: [ 'Farming', 'Milking Cows', ... ], engineering: [ 'Computer Engineering', 'Electrical Engineering', ... ], ... } }; Then, I would create a string of HTML markup, and use the innerHTML property to spit it out to the screen as follows: var html = '<select id="job_categories" name="job_categories">', skillSet; jobData.categories.forEach(function (option, i) { html += '<option value="' + i + '">' + option + '</option>'; }); html += '</select>'; for (skillSet in jobData.skills) { html += '<select id="job_skills_' + skillSet + '" name="job_skills_' + skillSet + '" class="job_skills hide">'; jobData.skills[skillSet].forEach(function (option, i) { html += '<option value="' + i + '">' + option + '</option>'; } html += '</select>'; } document.getElementById('content-or-whatever').innerHTML = html; That's all the code you need for rendering. Note that the hide class would have to reference some CSS that applied display: none;, and you'd still have to set up the onchange event for the job categories drop-down, but that's very trivial. If you need further assistance, please let us know. Thanks.
-
You should be able to either do a merge: http://stackoverflow.com/questions/2862590/how-to-replace-master-branch-in-git-entirely-from-another-branch Or you should be able to just push the entirety of the dev branch into the prod one: http://stackoverflow.com/questions/3789298/how-to-replace-git-repo That help?
-
Great post and great story. Thanks. Getting that first site going is always hard, but if you're like me, you'll be hooked and never look back. Also, I totally know what you mean by that high you feel when you finally get something working that you're stuck on forever. It's a great feeling. As for new stuff to learn, yeah, there's always something new to read about, but after a while, you do begin to plateau in your knowledge, and you realize that must new stuff is more just a slightly different view on the stuff we already have. And as for Yii, Yii is a PHP framework (and seemingly a pretty popular one, at that). Larry is the premier Yii writer, so you should definitely check out his Yii book, if you're interested. Also, I agree with what your PhD boss said about the truth being in the algorithms. The algorithm are ultimately what make a site a site; what give the site substance. Everything beyond that is just basic interface stuff to make the info on the site more easily accessible. Anyway, thanks a lot for your post. It was very interesting and I think very beneficial to people that are still starting off. Thanks.
-
I've never been an Apple fan, but I will say that Steve Jobs was a hell of a salesman. He knew how to make the most mundane things sound exciting.