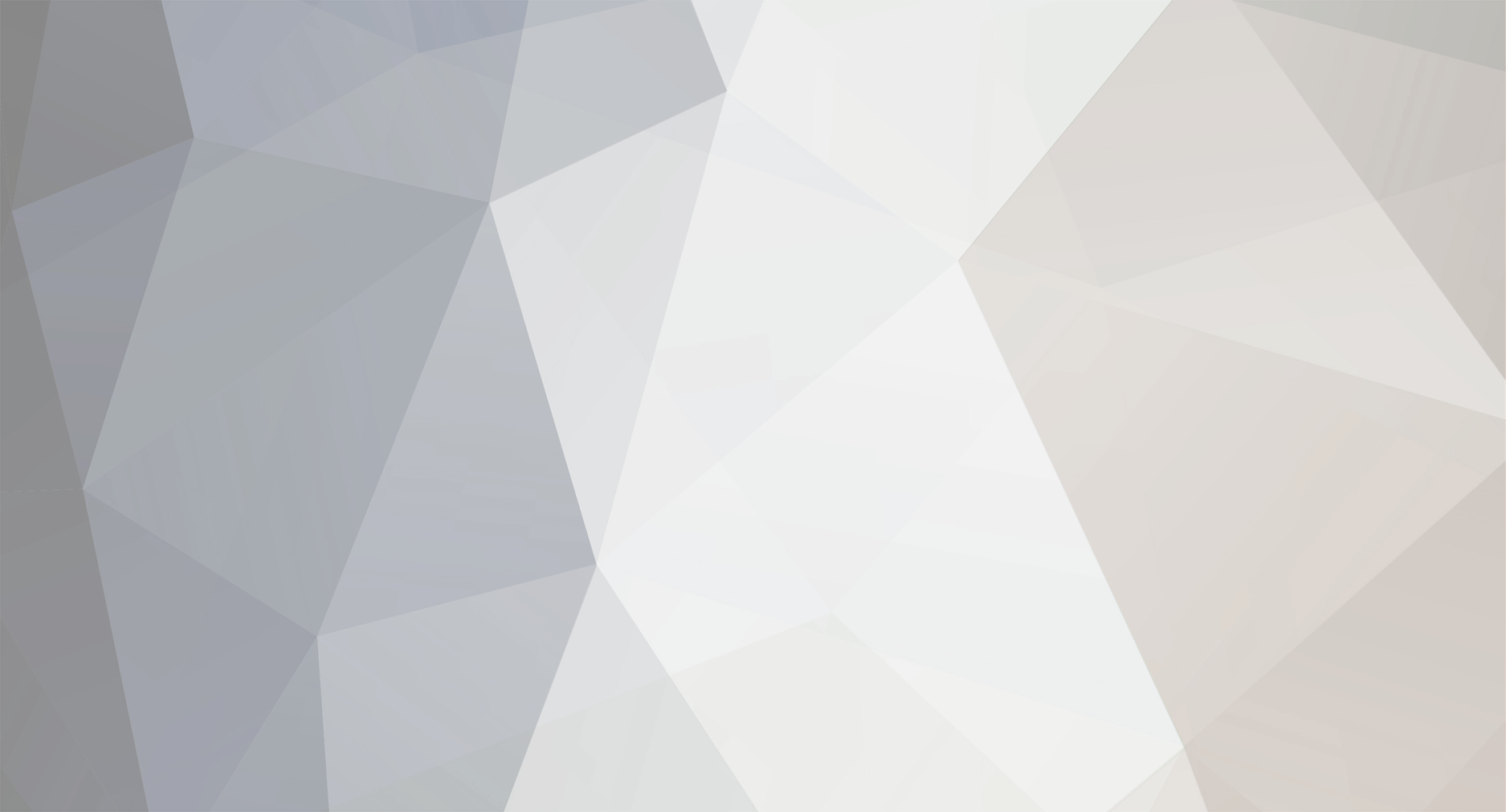
Jonathon
Members-
Posts
1064 -
Joined
-
Last visited
-
Days Won
55
Everything posted by Jonathon
-
Hello, I have been writing a login script, which was failing and then I got it to work. But i'm having trouble understanding a couple of occurrences. If i register using this script: if($_SERVER['REQUEST_METHOD'] == 'POST'){ // check for first name // allows letters, a space, full stop, apostrophe, hyphen if (preg_match ('/^[A-Z \'.-]{2,20}$/i', $_POST['first_name'])) { $fn = mysqli_real_escape_string ($dbc, $_POST['first_name']); } else { $errors['first_name'] = 'Please enter your first name!'; } // check for last name if(preg_match('/^[A-Z\'.-]{2,40}$/i', $_POST['last_name'])) { $ln = mysqli_real_escape_string($dbc, $_POST['last_name']); } else { $errors['last_name'] = 'Please enter your last name'; } // check email address if(filter_var($_POST['email'], FILTER_VALIDATE_EMAIL)) { $e = mysqli_real_escape_string($dbc, $_POST['email']); } else { $errors['email'] = 'Please enter a valid email address'; } // check for company if(preg_match('/^[A-Z\'.-]{2,40}$/i', $_POST['company'])) { $comp = mysqli_real_escape_string($dbc, $_POST['company']); } else { $errors['company'] = 'Please enter your company name or state individual'; } // check for a password and match against the confirmed password: if (preg_match ('/^(\w*(?=\w*\d)(?=\w*[a-z])(?=\w*[A-Z])\w*){6,20}$/', $_POST['pass1']) ) { if ($_POST['pass1'] == $_POST['pass2']) { $p = mysqli_real_escape_string($dbc, $_POST['pass1']); } else { $errors['pass2'] = 'Your password did not match the confirmed password!'; } } else { $errors['pass1'] = '6-20 characters long with at least 1 lower-case letter, 1 upper-case letters & 1 number'; } if(empty($errors)) { $q = "SELECT `email` FROM `users` WHERE `email`='$e'"; $r = mysqli_query($dbc, $q); $rows = mysqli_num_rows($r); if($rows === 0){ // No users exist $active = md5(uniqid(rand(), true)); $query = "INSERT INTO `users` (`first_name`, `last_name`, `email`, `company`, `pass`, `active`) VALUES ('$fn', '$ln', '$e', '$comp', '" . passwordHash($p) . "', '$active')"; $r = mysqli_query($dbc, $query); if(mysqli_affected_rows($dbc) == 1) { echo '<div id="content"> <div class="register"> <h3>Thanks For Registering</h3><p>You have been succesfully registered please check your email account for an activation email.</p> </div></div>'; // call activation email script // pass name, email and firstname to script activationEmail($active, $fn, $e); //include footer include('include/footer.inc.php'); echo '</body></html>'; exit(); } else { // query failed trigger_error('<div id="content"><div class="register"><p class="error">You Could not be registered at this time due to a system error. We apologize for any inconvenience</p></div></div>'); } } else { // email in use $row = mysqli_fetch_array($r, MYSQLI_NUM); if($row[0] == $_POST['email']){ $errors['email'] = ' This email is already registered,<a href="' . BASE_URL . 'forgotten-password"> forgot password</a>'; } } // end of email registered already } } I am registered just fine and if I try to login using this script I can log in. $errors = array(); // validate the email address if(filter_var($_POST['email'], FILTER_VALIDATE_EMAIL)) { $e = mysqli_real_escape_string($dbc, $_POST['email']); } else { $errors['email'] = 'Please enter a valid email address'; } // validate password if(!empty($_POST['pass'])) { $p = mysqli_real_escape_string($dbc, $_POST['pass']); } else { $errors['pass'] = 'Please enter your password'; } // if there are no errors if(empty($errors)) { // execute query $q = "SELECT id, first_name, type FROM users WHERE (email='$e' AND pass='" . passwordHash($p) . "')"; $r = mysqli_query ($dbc, $q); if (mysqli_num_rows($r) == 1) { $row = mysqli_fetch_array($r, MYSQLI_NUM); $_SESSION['user_id'] = $row[0]; $_SESSION['first_name'] = $row[1]; } else { // not valid credentials $errors['login'] = 'The email and passwords do not match those on file'; } } // end of login But originally I used prepared statements to register and I noticed that when I use prepared statements it sets the DB password value differently to that of the non-prepared statements query. They are the same password but one appears as: and the other appears as My prepared statements script to register (which works fine in that it registers me) is: if($_SERVER['REQUEST_METHOD'] == 'POST'){ // prepare databse $query = "INSERT INTO `users` (`first_name`, `last_name`, `email`, `company`, `pass`, `active`) VALUES (?, ?, ?, ?, ?, ?)"; $stmt = mysqli_prepare($dbc, $query); mysqli_stmt_bind_param ($stmt, 'ssssss', $fn, $ln, $e, $comp, $p, $active); // check for first name // allows letters, a space, full stop, apostrophe, hyphen if (preg_match ('/^[A-Z \'.-]{2,20}$/i', $_POST['first_name'])) { $fn = mysqli_real_escape_string ($dbc, $_POST['first_name']); } else { $errors['first_name'] = 'Please enter your first name!'; } // check for last name if(preg_match('/^[A-Z\'.-]{2,40}$/i', $_POST['last_name'])) { $ln = mysqli_real_escape_string($dbc, $_POST['last_name']); } else { $errors['last_name'] = 'Please enter your last name'; } // check email address if(filter_var($_POST['email'], FILTER_VALIDATE_EMAIL)) { $e = mysqli_real_escape_string($dbc, $_POST['email']); } else { $errors['email'] = 'Please enter a valid email address'; } // check for company if(preg_match('/^[A-Z\'.-]{2,40}$/i', $_POST['company'])) { $comp = mysqli_real_escape_string($dbc, $_POST['company']); } else { $errors['company'] = 'Please enter your company name or state individual'; } // check for a password and match against the confirmed password: if (preg_match ('/^(\w*(?=\w*\d)(?=\w*[a-z])(?=\w*[A-Z])\w*){6,20}$/', $_POST['pass1']) ) { if ($_POST['pass1'] == $_POST['pass2']) { $p = mysqli_real_escape_string($dbc, $_POST['pass1']); $p = passwordHash($p); } else { $errors['pass2'] = 'Your password did not match the confirmed password!'; } } else { $errors['pass1'] = '6-20 characters long with at least 1 lower-case letter, 1 upper-case letters & 1 number'; } if(empty($errors)) { $q = "SELECT `email` FROM `users` WHERE `email`='$e'"; $r = mysqli_query($dbc, $q); $rows = mysqli_num_rows($r); if($rows === 0){ // No users exist $active = md5(uniqid(rand(), true)); // execute query mysqli_stmt_execute($stmt); if(mysqli_stmt_affected_rows($stmt) == 1) { echo '<div id="content"> <div class="register"> <h3>Thanks For Registering</h3><p>You have been succesfully registered please check your email account for an activation email.</p> </div></div>'; // call activation email script // pass name, email and firstname to script activationEmail($active, $fn, $e); //include footer include('include/footer.inc.php'); echo '</body></html>'; exit(); } else { // query failed trigger_error('<div id="content"><div class="register"><p class="error">You Could not be registered at this time due to a system error. We apologize for any inconvenience</p></div></div>'); } } else { // email in use $row = mysqli_fetch_array($r, MYSQLI_NUM); if($row[0] == $_POST['email']){ $errors['email'] = ' This email is already registered,<a href="' . BASE_URL . 'forgotten-password"> forgot password</a>'; } } // end of email registered already } // clode statement mysqli_stmt_close($stmt); // close connection mysqli_close($dbc); } // end of submitted form And this script I used to login which fails is $errors = array(); // login query $q = "SELECT id, first_name, type FROM users WHERE (email=? AND pass=?)"; $stmt = mysqli_prepare($dbc, $q); mysqli_stmt_bind_param($stmt, 'ss', $e, $p); // validate the email address if(filter_var($_POST['email'], FILTER_VALIDATE_EMAIL)) { $e = mysqli_real_escape_string($dbc, $_POST['email']); } else { $errors['email'] = 'Please enter a valid email address'; } // validate password if(!empty($_POST['pass'])) { $p = mysqli_real_escape_string($dbc, $_POST['pass']); $p = passwordHash($p); } else { $errors['pass'] = 'Please enter your password'; } // if there are no errors if(empty($errors)) { // execute query mysqli_stmt_execute($stmt); if(mysqli_stmt_num_rows($stmt) == 1){ // query worked $row = mysqli_fetch_array($r, MYSQLI_NUM); $_SESSION['user_id'] = $row[0]; $_SESSION['first_name'] = $row[1]; } else { // not valid credentials $errors['login'] = 'The email and passwords do not match those on file'; } // close statement mysqli_stmt_close($stmt); //close connection mysqli_close($dbc); } // end of login I'd like to use prepared statements but I'm at a loss really as to why the prepared statements query inserts a different hashed representation of the password and subsequently why I don't seem to be able to login using prepared statements. I understand why If i mixed and matched between SQL and prepared SQL as the hash is not the same. (I apologise for the length of code too).
-
Right, I am getting somewhere maybe here. I took the password, hashed it in a test script and echoed it out, it returned: Then I queried the database just using the id field and returned the within the row password and that returned: Notice how the final character is missing from it. I shall re-look at my registration script to see if I can see anything. I shall look at this, but it still doesn't explain to me how when I just use the value of the pass field in the SQL query within the client it returns 0 results my password column is that of the ecommerce book `pass` varbinary(32) To Update: I feel i'm making progress, i've reworked my query within PHP and it seems to let me log in now, I was using prepared statements, which I've never used before, so I went back to basics and used standard SQL. But the reason I did this was because I built the ecommerce project 1 in Larry's book a while ago and I still had the database knocking around, so I ran the same query, i.e. trying to find a row within the client using the pass fields value. This FAILED (Which is really strange to me, perhaps Larry knows why, maybe its something to do with the field being varbinary). But the login work(s|ed) and always has within the browser for Knowledge is Power. So I suspect that the initial prepared statements SQL i was using through PHP somewhere had a fault in it, although I did first of all try to look there for a problem. Then I turned to the client as I was getting nowhere, which it would appear wouldn't verify that the pass field was correct even though it is. I know that the logins worked for larry's first ecommerce project and have tested them today, so I went back to my PHP script rewrote them all in standard SQL and it would appear to be working now. I am still clueless as to why this occured really. But I thank all who have put suggestions forward. I hope that this is resolved now, but we'll see, i'll do some more tidying/testing.
-
Thanks Craig, the only thing I can see now is that If just return the whole row using just an id field to find it using PHP in the browser. Is that the pass field although inside the DB as it is returned from the DB in the browser as I exported the table to see what was happening and it's stored as also.
-
Hi Craig & Josee, This is the password field as requested -> I've just double clicked on it to show that it is all of the hash. I'm just copying it from the row and pasting it into the query. So i don't see how I've accidentally missed a character or anything. I'm not sure if what you speak of has happened to me Josee, I would think not as I have tried it so many times. Rob thanks for your input, I have tried with and without back-ticks, either way i believe the query is still valid and it doesn't return any rows. I think you would still have to quote the password value as it's a string not an integer however.
-
Login In Chapter 4
Jonathon replied to capecodheaven's topic in Effortless E-Commerce with PHP and MySQL
Simply, somewhere in your code or your included files you are calling the same function escape_data(). What is your full code? -
Larry & all, I'm having a strange occurrence and quite frankly it's beaten me (so far). I'm writing a login script which is failing, so I debugged and then hit the client with the query. The query executes fine but 0 results are returned. SO i started to dissect the query, this query worked fine: SELECT `user_id` FROM users WHERE `email`='myemail@email.com' and every subsequent query worked using the WHERE clause except for when I searched using the WHERE clause for the `pass` column. Every time I try to find the password column the query failed. What am I doing wrong? I'm really bamboozled by this: This is as simple a query I could make and it returns 0 results and the column is definitely pass and the value of the row (There is only 1 row in the table) is definitely correct. SELECT * FROM `users` WHERE `pass`='bb4c572b60661fe7dff6cca2fffc8c2155cb2cf85c5ce9f367f1c8df1228029d' I feel very confused and somewhat embarrassed asking this question
-
This error is often associated with missing braces. Do you have a text editor that can help you balance them? I know Notepad++ does for windows, that's free to download too. Anyway I think the problem at a quick glance is your missing '}' after the last echo statement. } else { //invalid submit values echo '<h1>Error!</h1> <p>Please submit valid distance, price per gallon and feul efficiency</p>'; } // Missing brace } //end of main submission "if" statement //Leave the PHP section and create the html form ?>
-
First Site Password Regex
Jonathon replied to Jonathon's topic in Effortless E-Commerce with PHP and MySQL
I think I worked out what the problem is?? Although I may be wrong, there is a missing full stop/period (in American) before {6,20} (I think) I think this should be the code. ^(\w*(?=\w*\d)(?=\w*[a-z])(?=\w*[A-Z])\w*).{6,20}$ I did check the errata for page 86, but there was no mention of it. -
Hello, I feel like there may already be something about this somewhere on here, if so please delete my post/ direct me to it.I am writing a registration script and referencing the first site in this book. I'm currently doing some testing of the form and I noticed that this regex ^(\w*(?=\w*\d)(?=\w*[a-z])(?=\w*[A-Z])\w*){6,20}$ allows for passwords shorter than 6 characters. At least on my system, I even copied the code from the download files and it still let a password in, along as it contained 1 capital letter, 1 lower-case letter and 1 digit that was smaller than 6 characters in length. I also ran it through a Regex tool and it allowed a string smaller than 6 characters through as long as it met the letter specs. Is this me? This is the code that I am running // check for a password and match against the confirmed password: if (preg_match ('/^(\w*(?=\w*\d)(?=\w*[a-z])(?=\w*[A-Z])\w*){6,20}$/', $_POST['pass1']) ) { if ($_POST['pass1'] == $_POST['pass2']) { $p = mysqli_real_escape_string ($dbc, $_POST['pass1']); } else { $errors['pass2'] = 'Your password did not match the confirmed password!'; } } else { $errors['pass1'] = '6-20 characters long with at least 1 lower-case letter, 1 upper-case letters & 1 number'; }
-
Pdf Image Inclusion Errors
Jonathon replied to Jonathon's topic in PHP 5 Advanced: Visual QuickPro Guide (2nd Edition)
Since reading either your e-commerce book or blog posts I have now started to consider Zend as an alternative to a lot of other (often outdated) packages. I used it for sending a HTML email with the ecommerce book and it's very clean and one would automatically presume that their security is also very top notch. That's not saying that mine isn't but accidents and bugs do happen, so that's reassuring. I've also started to use it for my Lucene project too and I could very well start using it for the PDFs too. I haven't had chance really to look at either my Lucene or PDF attempts since I posted, something inevitably comes up, but I shall let you know how I get on. Although I haven't looked into it, I wouldn't be surprised if Zend on a whole provides similar examples of some of PEARs better features, maybe such as a GEO-location package. Though I don't think I'd ever warrant using Zend from scratch or actually want to use it unless a was attempting something fairly colossal. -
Hi Larry,Please Help Me About Pear
Jonathon replied to Malaysian's topic in Effortless E-Commerce with PHP and MySQL
Malaysian, you shouldn't really start a new topic just because you haven't been given the answer you want in another topic.I have tried to help you, obviously there is a language barrier, which I sympathise with, but unfortunatley it doesn't help us trying to resolve your problem. please answer these questions: 1) Have you used the link i gave you to try and set up your system environment. 2) what happens when you try to use the command prompt? 3) What do you need PEAR for? -
Pdf Image Inclusion Errors
Jonathon replied to Jonathon's topic in PHP 5 Advanced: Visual QuickPro Guide (2nd Edition)
Hi Larry, No they're not very helpful, you'd think even just a couple of words wouldn't have been too hard. I shall try and fix this error tomorrow and let you know how I get on. Thanks for the help. -
Zend_Lucene
Jonathon replied to Jonathon's topic in PHP 5 Advanced: Visual QuickPro Guide (2nd Edition)
I've used Sphider before and I was fairly happy with it, but I feel (possibly wrongly that Lucene would be a more powerful tool, maybe that's just because it's from Zend). A nice little spider would be nice, but I suppose using a function to pull up all the URLs isn't so bad really, I imagine it's a lot easier too. I'm intrigued by these .gen files now, I'd like to see what exactly they have put into the index and how it differs loading the urls as HTML documents rather than using something like this (Which is an extended class of the Zend_Search_Lucene_Document: public function __construct($document) { $this->addField(Zend_Search_Lucene_Field::Keyword('document_id', $document->id)); $this->addField(Zend_Search_Lucene_Field::UnIndexed('url', $document->url)); $this->addField(Zend_Search_Lucene_Field::UnIndexed('created', $document->created)); $this->addField(Zend_Search_Lucene_Field::UnIndexed('teaser', $document->teaser)); $this->addField(Zend_Search_Lucene_Field::Text('title', $document->title)); $this->addField(Zend_Search_Lucene_Field::Text('author', $document->author)); $this->addField(Zend_Search_Lucene_Field::UnStored('content', $document->body)); } Which is how the article not about Yii indexed documents. But I'm sure all will become clear (I hope). I'll look into searching/querying the indexes now, I guess that's the next step. Thanks for the help -
Is this is Larry's book? I don't have it. To pen the command prompt you need to type cmd in the run/search facility in Windows start up. But you also need to set you system environmental path to the write location. Maybe this will help you achieve this so the command prompt works. http://www.blog.highub.com/php/running-php-using-windows-command-line-with-xampp/
-
Zend_Lucene
Jonathon replied to Jonathon's topic in PHP 5 Advanced: Visual QuickPro Guide (2nd Edition)
Thanks Larry, i'll keep trying to read up and trying to get something. -
Hello Larry and all, I have finally got round to trying to integrate Zend's lucene into a website (Well not really, just my local host, but if all goes well, I'll roll it on a live project, in place of Sphider). I should mention that this local project will not be using Zend as a framework on a whole but it will use Zend for the Lucene module. I've had a little bash at it although not a lot as I wanted to check my logic first, i've read up on it at Zend, but obviously that is all geared through their framework, but it was still good to see their code, I've also read a good series of posts at http://www.phpriot.com/articles/zend-search-lucene (Hope that link is ok here, I feel like you said anything that can aid people is ok?If not, feel free to remove my link). I've also read your articles on it with Yii, just using small snippets of stuff from phpriot I have managed to generate a folder which I guess holds the documents. These appear as .gen files such as segments.gen, segment_1, write.lock.file, read.lock.file. I guess those are the documents for the index is that correct? I'm far from a) fully understanding lucence yet and b: getting a working search engine but I wanted to run a couple of questions by you. On your creating an index series you say that So to me that would suggest loading every url through the $doc = Zend_Search_Lucene_Document_Html::loadHTMLFile($url); Which I also guess wouldn't be that hard by using something as my Sitemap.xml and extrapolating the raw urls out. I imagine that this too, is the safer/easier bet as the full HTML page would already have everything that I could want to be indexed, although more computationally expensive i'm sure. The main reason that i'm leaning to this approach is that I can't guarantee that all my content will be stored in a DB and for my first attempt, I always think trying to keep it simple is a good starting block. So trying to piece together a start would you say that this overview is ok?bad?wrong or ugly Or ALL of THEM! <?php require_once('Zend/Search/Lucene.php'); // where to save our index $indexPath = 'C:\xampp\htdocs\ZendSearch\\'; // create index $index = Zend_Search_Lucene::create($indexPath); /** * list of urls * this should be a function to return each url * rather than a hardcode **/ $urlArray = array( 'http://www.link1.com/', 'http://www.link2.com/', 'http://www.link3.com/', 'http://www.link4.com/' ); // iterate urls and load the html and add to index foreach ($urlArray as $url) { $doc = Zend_Search_Lucene_Document_Html::loadHTMLFile($url); $index->addDocument($doc); } After that I'm unsure, that comits the documents (urls) to the index I take it? I also note in both your example and phpriots that you can add your own fields, by doing what I suggest do I severely limit the ability of the search as I don't pass it any fields. Thanks Jonathon
-
Changing Size Of Text Fields
Jonathon replied to Marie's topic in Effortless E-Commerce with PHP and MySQL
I'm pretty sure early on I showed you the line of css code you need to change to adjust the physical width of the textarea or input (I can't remember exactly and my phone is slow). You can use either HTML as suggested or CSS to achieve the same result, but I do feel that CSS is the more accepted method. However, you should focus on just trying to get the form to display however you want it to visually appear at the moment, so I would leave out the php for the moment as that is causing you some kind of error. Also have you looked into using firefox and the web developer extension?? It is extremely helpful for situations like this, where you have CSS code that you haven't written as it tells you the html element and path through the document to that element along with the associated CSS and also allows you to edit the CSS in the browser. No messing around, then you know where to start with yo adjustments. -
There's nothing to activate I don't think. What are you trying to do? The only thing you may have to do is make sure the package you want to use is up to date. But experience tells me that often PEAR packages aren't maintained I just tried to require the benchmark timer script and also the Auth.php it returned a Deprecated error and fatal error respectively. The fatal error problem I am going to look into as I think that it can be rectified. But what are you trying to do with PEAR?
-
Can you not use the user_id to then query the database and retrieve the person's name if I understand your problem?
-
Regex Query
Jonathon replied to Jonathon's topic in PHP 5 Advanced: Visual QuickPro Guide (2nd Edition)
No problem, thanks for the pointers :-)